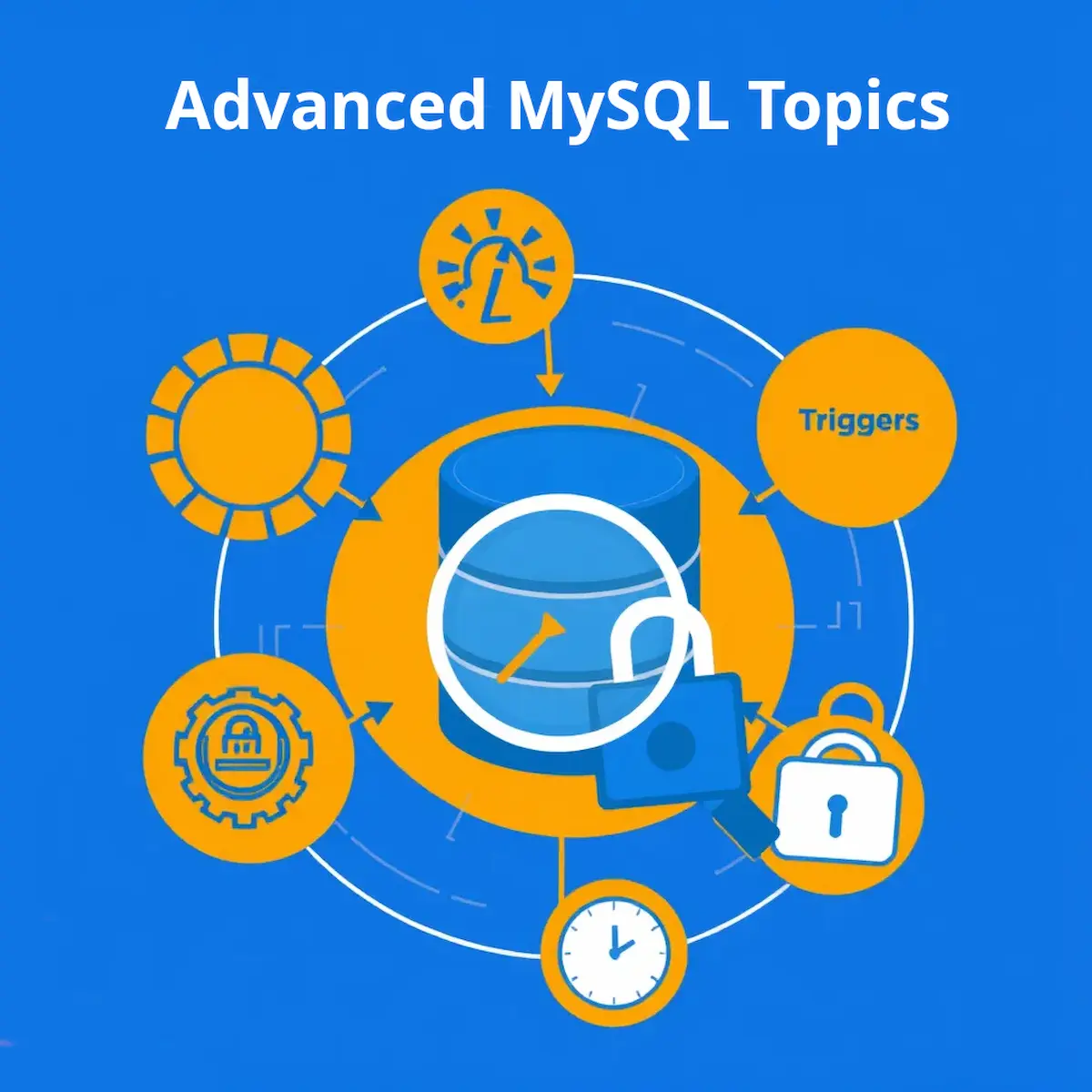
Advanced MySQL Topics: Mastering Stored Procedures, Triggers, and Transactions
- Ctrl Man
- Database Management , MySQL
- 29 Aug, 2024
Advanced MySQL Topics: Mastering Database Efficiency and Functionality
1. Stored Procedures and Functions
1.1 Overview
Stored procedures and functions are powerful tools in MySQL that enhance database functionality, efficiency, and security. They allow developers to encapsulate complex operations into reusable code blocks, which can be executed with a single call. These features offer several benefits:
- Improved performance by reducing network traffic
- Enhanced security through encapsulation of sensitive operations
- Easier maintenance and code organization
- Reusability across multiple applications
1.2 Stored Procedures
Creating Stored Procedures
To create a stored procedure in MySQL, use the CREATE PROCEDURE
statement:
DELIMITER //
CREATE PROCEDURE CalculateBonus (IN salary DECIMAL(10, 2), IN bonusRate DECIMAL(4, 2))
BEGIN
SELECT salary * bonusRate AS bonus FROM dual WHERE EXISTS (
SELECT 1 FROM employees WHERE employees.salary = salary AND employees.bonusRate = bonusRate);
END //
DELIMITER ;
Executing Stored Procedures
Execute stored procedures using the CALL
statement:
CALL CalculateBonus(5000.00, 0.1);
1.3 Stored Functions
Creating Stored Functions
Stored functions are similar to procedures but return a single value:
DELIMITER //
CREATE FUNCTION CalculateBonusRate(salary DECIMAL(10, 2), bonus DECIMAL(10, 2)) RETURNS DECIMAL(4, 2)
BEGIN
DECLARE new_rate DECIMAL(4, 2);
SET new_rate = bonus / salary;
RETURN new_rate;
END //
DELIMITER ;
Using Stored Functions
Use stored functions directly in SQL statements:
SELECT CalculateBonusRate(5000.00, 500.00) AS 'Bonus Rate';
1.4 Best Practices
- Implement robust error handling
- Use appropriate parameter types and validate inputs
- Optimize for performance, especially for frequently called procedures/functions
- Document your code thoroughly
2. Triggers and Events
2.1 Triggers
Triggers are automatically executed in response to specific events on a table, such as INSERT, UPDATE, or DELETE operations.
Creating Triggers
Use the CREATE TRIGGER
statement to define a trigger:
CREATE TRIGGER AfterSalaryUpdate
AFTER UPDATE ON employees
FOR EACH ROW
BEGIN
IF NEW.salary > OLD.salary THEN
INSERT INTO salary_changes (employee_id, old_salary, new_salary, change_date)
VALUES (NEW.id, OLD.salary, NEW.salary, NOW());
END IF;
END;
Managing Triggers
- List triggers:
SHOW TRIGGERS;
- Remove a trigger:
DROP TRIGGER trigger_name;
2.2 Events
Events are tasks that run according to a schedule, similar to cron jobs in Unix-like systems.
Creating Events
Use the CREATE EVENT
statement:
CREATE EVENT daily_cleanup
ON SCHEDULE EVERY 1 DAY
DO
BEGIN
DELETE FROM temp_logs WHERE created_at < DATE_SUB(NOW(), INTERVAL 7 DAY);
END;
Managing Events
- Enable event scheduler:
SET GLOBAL event_scheduler = ON;
- List events:
SHOW EVENTS;
- Disable an event:
ALTER EVENT event_name DISABLE;
3. Transactions and ACID Properties
3.1 Understanding ACID Properties
- Atomicity: All operations in a transaction succeed or the entire transaction is rolled back.
- Consistency: The database remains in a consistent state before and after the transaction.
- Isolation: Concurrent transactions do not interfere with each other.
- Durability: Once committed, transactions are permanent and survive system failures.
3.2 Working with Transactions
Basic Transaction Structure
START TRANSACTION;
-- SQL operations here
COMMIT;
-- Or ROLLBACK; if needed
Transaction Isolation Levels
MySQL supports four isolation levels:
- READ UNCOMMITTED
- READ COMMITTED
- REPEATABLE READ (default in InnoDB)
- SERIALIZABLE
Set the isolation level:
SET TRANSACTION ISOLATION LEVEL READ COMMITTED;
3.3 Advanced Transaction Techniques
- Use savepoints for partial rollbacks
- Implement proper error handling and logging
- Be mindful of lock contention in high-concurrency scenarios
4. Query Optimization Techniques
4.1 Indexing Strategies
- Create appropriate indexes based on query patterns
- Use composite indexes for multi-column conditions
- Avoid over-indexing, which can slow down write operations
4.2 EXPLAIN and Query Analysis
Use the EXPLAIN
statement to analyze query execution plans:
EXPLAIN SELECT * FROM employees WHERE department_id = 5;
4.3 Query Rewriting
- Avoid using
SELECT *
in production code - Use JOINs efficiently
- Leverage subqueries and derived tables for complex operations
5. Conclusion
Mastering these advanced MySQL topics is crucial for building robust, efficient, and secure database applications. By leveraging stored procedures, functions, triggers, and events, you can create more modular and maintainable code. Understanding transactions and ACID properties ensures data integrity in complex operations. Finally, query optimization techniques help in achieving peak performance for your database-driven applications.
Remember to always test thoroughly in a non-production environment before implementing these advanced features in live systems. Stay updated with MySQL’s documentation and community resources to continue improving your database management skills.