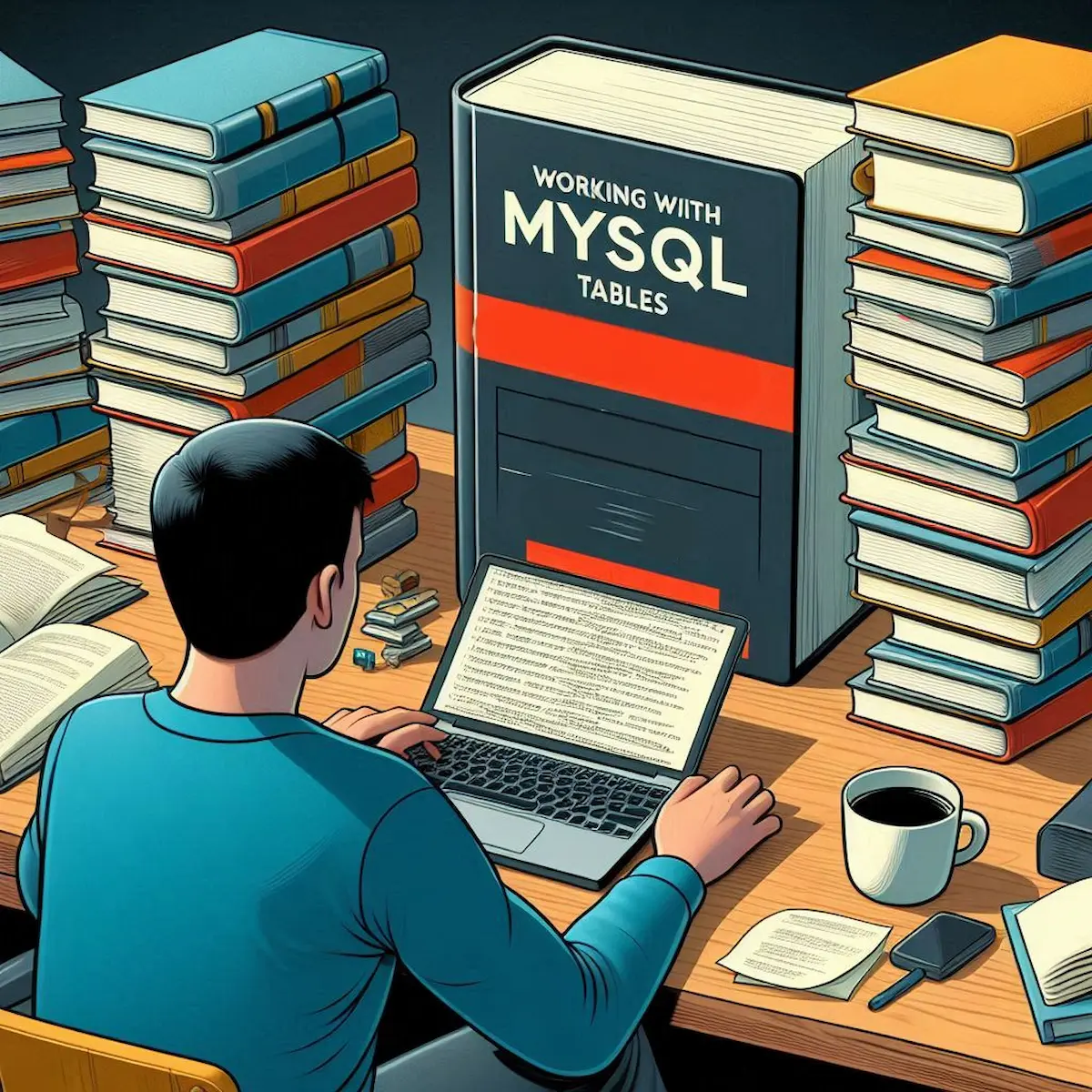
Working with MySQL Tables: A Comprehensive Guide
- Ctrl Man
- Database , MySQL
- 15 Jul, 2024
Working with MySQL Tables: A Comprehensive Guide
Introduction
In the dynamic world of database management, the ability to efficiently modify and manage MySQL tables is crucial. As applications evolve and business requirements change, database administrators and developers often need to alter table structures, add or remove columns, modify data types, and sometimes even drop entire tables. This guide provides a comprehensive overview of working with MySQL tables, equipping you with the knowledge to perform these operations confidently and safely.
1. Altering Table Structure (ALTER TABLE)
The ALTER TABLE
statement in MySQL is a powerful tool for modifying existing tables. It allows you to add, remove, or modify columns, as well as change table properties.
1.1 Adding Columns
To add a new column to an existing table, use the ADD COLUMN
clause:
ALTER TABLE table_name ADD COLUMN column_name datatype [constraints];
Example:
ALTER TABLE employees ADD COLUMN email VARCHAR(100) NOT NULL;
You can also add multiple columns in a single statement:
ALTER TABLE employees
ADD COLUMN phone VARCHAR(20),
ADD COLUMN hire_date DATE;
1.2 Removing Columns
To remove a column from a table, use the DROP COLUMN
clause:
ALTER TABLE table_name DROP COLUMN column_name;
Example:
ALTER TABLE employees DROP COLUMN email;
Be cautious when dropping columns, as this operation permanently deletes the associated data.
1.3 Modifying Column Data Types
You can change the data type of an existing column using the MODIFY COLUMN
clause:
ALTER TABLE table_name MODIFY COLUMN column_name new_datatype [constraints];
Example:
ALTER TABLE employees MODIFY COLUMN salary DECIMAL(10,2);
When modifying column data types, ensure that the new type is compatible with the existing data to avoid loss of information.
2. Advanced Table Alterations
2.1 Adding and Removing Indexes
To add an index:
ALTER TABLE table_name ADD INDEX index_name (column1, column2, ...);
To remove an index:
ALTER TABLE table_name DROP INDEX index_name;
2.2 Adding and Removing Primary Keys
To add a primary key:
ALTER TABLE table_name ADD PRIMARY KEY (column1, column2, ...);
To remove a primary key:
ALTER TABLE table_name DROP PRIMARY KEY;
2.3 Adding and Removing Foreign Keys
To add a foreign key:
ALTER TABLE table_name
ADD CONSTRAINT constraint_name
FOREIGN KEY (column_name) REFERENCES referenced_table(referenced_column);
To remove a foreign key:
ALTER TABLE table_name DROP FOREIGN KEY constraint_name;
3. Dropping Tables (DROP TABLE)
The DROP TABLE
statement is used to remove an entire table from the database.
3.1 Basic Syntax
DROP TABLE [IF EXISTS] table_name;
Example:
DROP TABLE IF EXISTS employees;
The IF EXISTS
clause prevents an error if the table doesn’t exist.
3.2 Dropping Multiple Tables
You can drop multiple tables in a single statement:
DROP TABLE table1, table2, table3;
3.3 Considerations When Dropping Tables
- Dropping a table permanently deletes all data and the table structure.
- Ensure you have proper backups before dropping tables.
- Check for dependencies (e.g., foreign key constraints) before dropping tables.
4. Error Handling and Common Warnings
When working with MySQL tables, you may encounter various errors and warnings. Here are some common ones and how to handle them:
-
Duplicate column name: Error: “Duplicate column name ‘column_name’” Solution: Ensure you’re not adding a column that already exists or rename the new column.
-
Data truncation: Warning: “Data truncated for column ‘column_name’ at row X” Solution: Check that the new data type can accommodate existing data when modifying column types.
-
Cannot drop index needed in a foreign key constraint: Error: “Cannot drop index ‘index_name’: needed in a foreign key constraint” Solution: Drop the foreign key constraint before dropping the index.
-
Row size too large: Error: “Row size too large. The maximum row size for the used table type, not counting BLOBs, is 65535” Solution: Consider using vertical partitioning or moving some columns to separate tables.
To handle errors gracefully in your applications, always wrap DDL statements in try-catch blocks and implement proper logging and notification systems.
5. Performance Considerations for Large Tables
Altering large tables can be a time-consuming process and may impact database performance. Here are some strategies to minimize downtime:
-
Use Online Schema Change Tools: Tools like
pt-online-schema-change
from Percona Toolkit can perform alterations with minimal table locking. -
Batched Updates: For large data migrations, process the data in smaller batches to reduce memory usage and lock duration.
-
Off-Peak Hours: Schedule major alterations during off-peak hours to minimize disruption to users.
-
Replication: Use replication to perform changes on a slave server first, then promote it to master once the changes are complete.
-
Optimize Before Alter: Run
OPTIMIZE TABLE
before complex alterations to defragment the table and potentially speed up the process.
Example using pt-online-schema-change:
pt-online-schema-change --alter "ADD COLUMN email VARCHAR(100)" D=database_name,t=table_name --execute
6. Real-World Scenarios
Scenario 1: E-commerce Platform Expansion
An e-commerce platform needed to add support for multiple currencies. This required adding a new ‘currency’ column to the ‘orders’ table, which contained millions of records.
Solution:
- A new ‘currency’ column was added using pt-online-schema-change to minimize downtime.
- A background job was created to populate the new column with default values.
- Application code was updated to use the new column.
pt-online-schema-change --alter "ADD COLUMN currency CHAR(3) DEFAULT 'USD'" D=ecommerce,t=orders --execute
Scenario 2: GDPR Compliance
A company needed to implement a data retention policy to comply with GDPR, requiring the deletion of user data after a certain period.
Solution:
- A ‘last_activity_date’ column was added to the ‘users’ table.
- A scheduled job was set up to soft-delete user data based on this date.
- Indexes were added to optimize the deletion queries.
ALTER TABLE users
ADD COLUMN last_activity_date DATE,
ADD INDEX idx_last_activity (last_activity_date);
Conclusion
Mastering MySQL table management is essential for maintaining a flexible and efficient database schema. By understanding how to alter table structures, handle errors, and optimize performance for large-scale operations, you can confidently manage your MySQL databases as they grow and evolve. Always remember to back up your data before making significant changes, test alterations in a non-production environment first, and consider the impact on existing queries and application code. With these skills and best practices, you’ll be well-equipped to handle the dynamic needs of modern database management.
Best Practices and Tips
- Always backup your database before making significant structural changes.
- Use transactions for complex alterations to ensure data integrity.
- Consider the impact on existing queries and application code when modifying table structures.
- For large tables, alterations can be time-consuming. Plan for downtime or use online schema change tools for production environments.
- Regularly review and optimize your table structures for better performance.
- Implement a change management process for database schema modifications in team environments.
- Keep your MySQL version up-to-date to benefit from the latest performance improvements and features related to table management.
By following these guidelines and understanding the intricacies of MySQL table management, you’ll be well-prepared to handle database schema changes efficiently and safely in your projects.