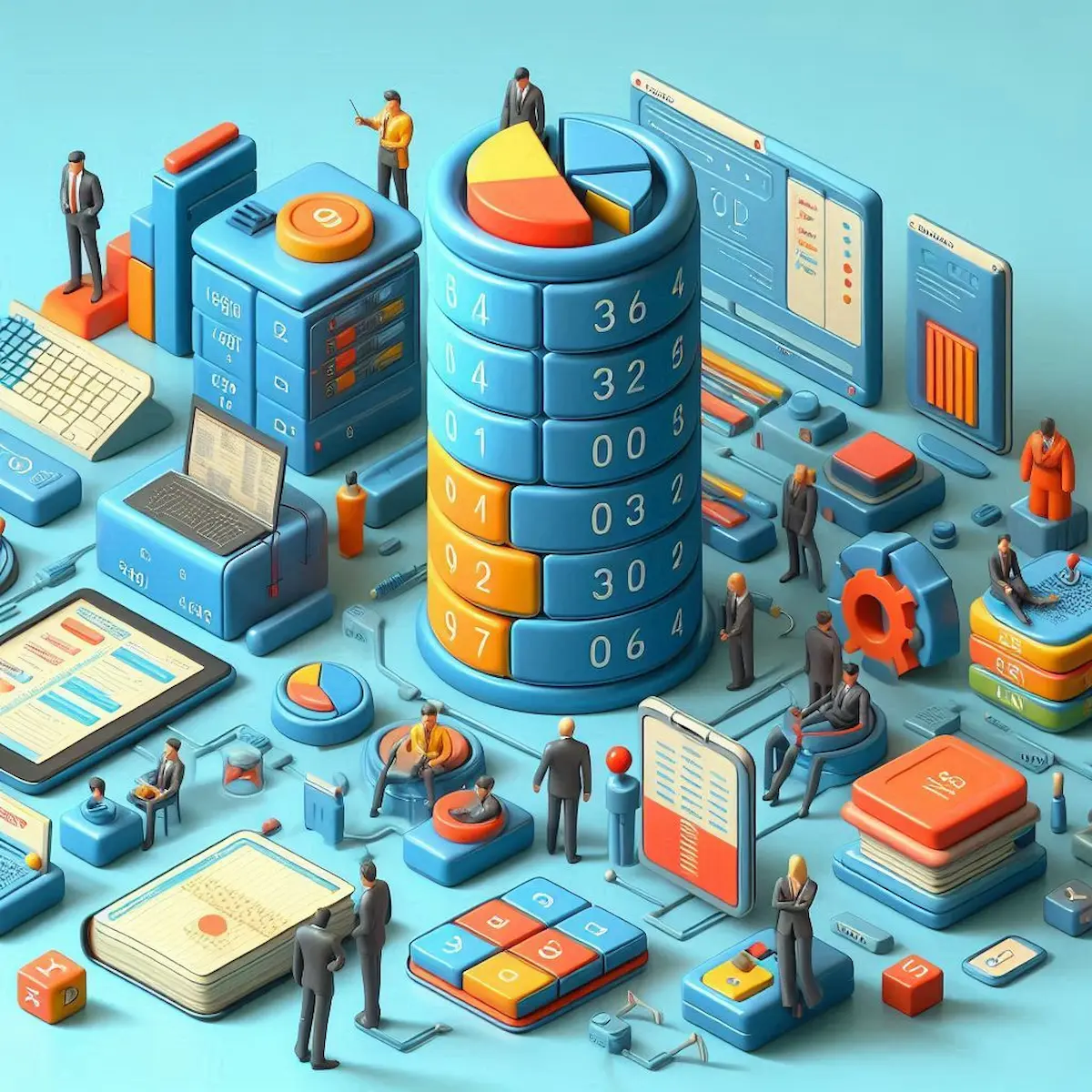
MySQL and Localization: Mastering Number Formats and Character Sets
- Ctrl Man
- Database , MySQL , Localization
- 23 Jul, 2024
MySQL and Localization: A Comprehensive Guide to Handling Different Number Formats, Character Sets, and Best Practices
Introduction
In today’s globalized digital landscape, localization is crucial for software applications to cater to diverse users across different regions. This comprehensive guide explores the intricacies of handling various number formats within MySQL databases, managing character sets and collations, and provides best practices for storing and displaying localized data.
Different Number Formats
Decimal Separators
Different countries use various symbols as decimal separators, which can significantly impact database management. For instance, the United States uses a period (.), while many European countries, including Poland, use a comma (,).
Code Example
-- Setting up a table to store numeric values with different formats
CREATE TABLE numbers (
id INT AUTO_INCREMENT PRIMARY KEY,
number VARCHAR(25) NOT NULL,
country ENUM('US', 'PL') NOT NULL
);
-- Inserting records with various decimal separators
INSERT INTO numbers(number, country)
VALUES ('1.1234', 'US'), ('1,1234', 'PL');
SELECT * FROM numbers;
Grouping Thousands Separator
To improve readability of large numbers, many locales use a thousands separator. In the US, this is typically a comma, while in Poland, it’s often a space.
Code Example of Grouping Thousands Separator
SET SESSION sql_mode = '';
-- Check current group separator
SELECT @@lc_numeric AS CurrentLocale;
-- Set to Polish locale
SET lc_numeric = 'pl_PL';
-- Displaying numbers with a thousands separator
SELECT FORMAT(1234567.89, 2) AS FormattedNumber;
Handling Different Formats in SQL Queries
When working with data across different formats, it’s essential to ensure correct interpretation and processing. MySQL provides functions to handle locale-specific string operations.
Code Example of Handling Different Formats in SQL Queries
-- Converting string to number, considering locale
SELECT CAST(REPLACE(number, ',', '.') AS DECIMAL(10,2)) AS converted_number
FROM numbers
WHERE country = 'PL';
Character Sets and Collations
Understanding Character Sets
Character sets define how characters are encoded in a database. Different locales may require specific encodings to support their alphabets or scripts.
Code Example of Character Sets
-- Setting up a table for storing text with localized language
CREATE TABLE texts (
id INT AUTO_INCREMENT PRIMARY KEY,
content TEXT CHARACTER SET utf8mb4 COLLATE utf8mb4_polish_ci NOT NULL,
language ENUM('EN', 'PL') NOT NULL
);
-- Inserting records with different languages
INSERT INTO texts(content, language)
VALUES ('Cześć!', 'PL'), ('Hello!', 'EN');
SELECT * FROM texts;
Collations in Action
Collations determine how strings are compared within a database. Choosing the correct collation ensures that string comparisons behave as expected across various locales.
Code Example of Collations
-- Setting up tables with different collations for English and Polish
CREATE TABLE texts_en (id INT, content VARCHAR(255) COLLATE utf8mb4_unicode_ci) ENGINE=InnoDB;
CREATE TABLE texts_pl (id INT, content VARCHAR(255) COLLATE utf8mb4_polish_ci) ENGINE=InnoDB;
-- Inserting records with non-ASCII characters
INSERT INTO texts_en(id, content) VALUES (1, 'łódź');
INSERT INTO texts_pl(id, content) VALUES (1, 'łódź');
-- Comparing sorting behavior
SELECT * FROM texts_en ORDER BY content;
SELECT * FROM texts_pl ORDER BY content;
Best Practices for Storing and Displaying Localized Data
Proper Encoding and Storage Formats
Always store text data in a compatible encoding that supports all required locales. This prevents issues with displaying characters correctly.
Code Example of Encoding
CREATE TABLE localized_data (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(50) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
description TEXT CHARACTER SET utf8mb4 COLLATE utf8mb4_polish_ci NOT NULL
);
Dynamic Formatting
When displaying data, format the output dynamically based on user preferences or application settings to provide a localized experience.
Code Example (PHP)
<?php
$number = 1234567.89;
$locale = 'pl_PL';
// Set number formatting for Polish locale
setlocale(LC_NUMERIC, $locale);
$formatted_number = number_format($number, 2, ',', ' ');
echo "$formatted_number is in the $locale format.";
?>
Error Handling and Validation
Implement robust error handling and validation mechanisms to deal with unexpected input formats or characters that might not fit into your database schema.
Code Example (PHP) of Error Handling
<?php
function validatePolishNumber($input) {
// Polish number format: optional thousands separator (space), comma as decimal separator
if (!preg_match("/^(\d{1,3}( \d{3})*|\d+)(,\d+)?$/", $input)) {
throw new Exception("Invalid Polish number format.");
}
return str_replace([' ', ','], ['', '.'], $input);
}
try {
$input = "1 234,56";
$validNumber = validatePolishNumber($input);
echo "Valid number: $validNumber";
} catch (Exception $e) {
echo $e->getMessage();
}
?>
Additional Considerations
Time and Date Formats
Different locales have varying conventions for representing dates and times. MySQL provides functions to handle these differences.
Code Example of Time and Date Formats
-- Setting the time zone
SET time_zone = '+01:00'; -- Central European Time (Poland)
-- Inserting a date
INSERT INTO events (event_date) VALUES ('2023-07-19 14:30:00');
-- Displaying the date in different formats
SELECT DATE_FORMAT(event_date, '%d.%m.%Y %H:%i') AS polish_format,
DATE_FORMAT(event_date, '%Y-%m-%d %h:%i %p') AS us_format
FROM events;
Currency Handling
When dealing with monetary values, consider storing amounts in a standard format (e.g., cents) and applying formatting when displaying.
Code Example (PHP) of Currency Handling
<?php
$amount_in_cents = 123456; // Stored in database
$locale = 'pl_PL';
setlocale(LC_MONETARY, $locale);
$formatted_amount = money_format('%.2n', $amount_in_cents / 100);
echo "Amount in PLN: $formatted_amount";
?>
Conclusion
Effective localization in MySQL databases requires a deep understanding of number formats, character sets, and collations to ensure compatibility across different locales. By implementing best practices for storing, displaying, and validating localized data, you can create robust applications that cater to diverse user needs.
As technology continues to evolve globally, staying informed about these nuances is crucial for developing inclusive and accessible software solutions. Regularly review and update your database management strategies to meet the ever-changing demands of localization requirements.
Remember that localization goes beyond just database management - it also involves adapting user interfaces, content, and overall user experience to meet the cultural and linguistic expectations of your target audience.