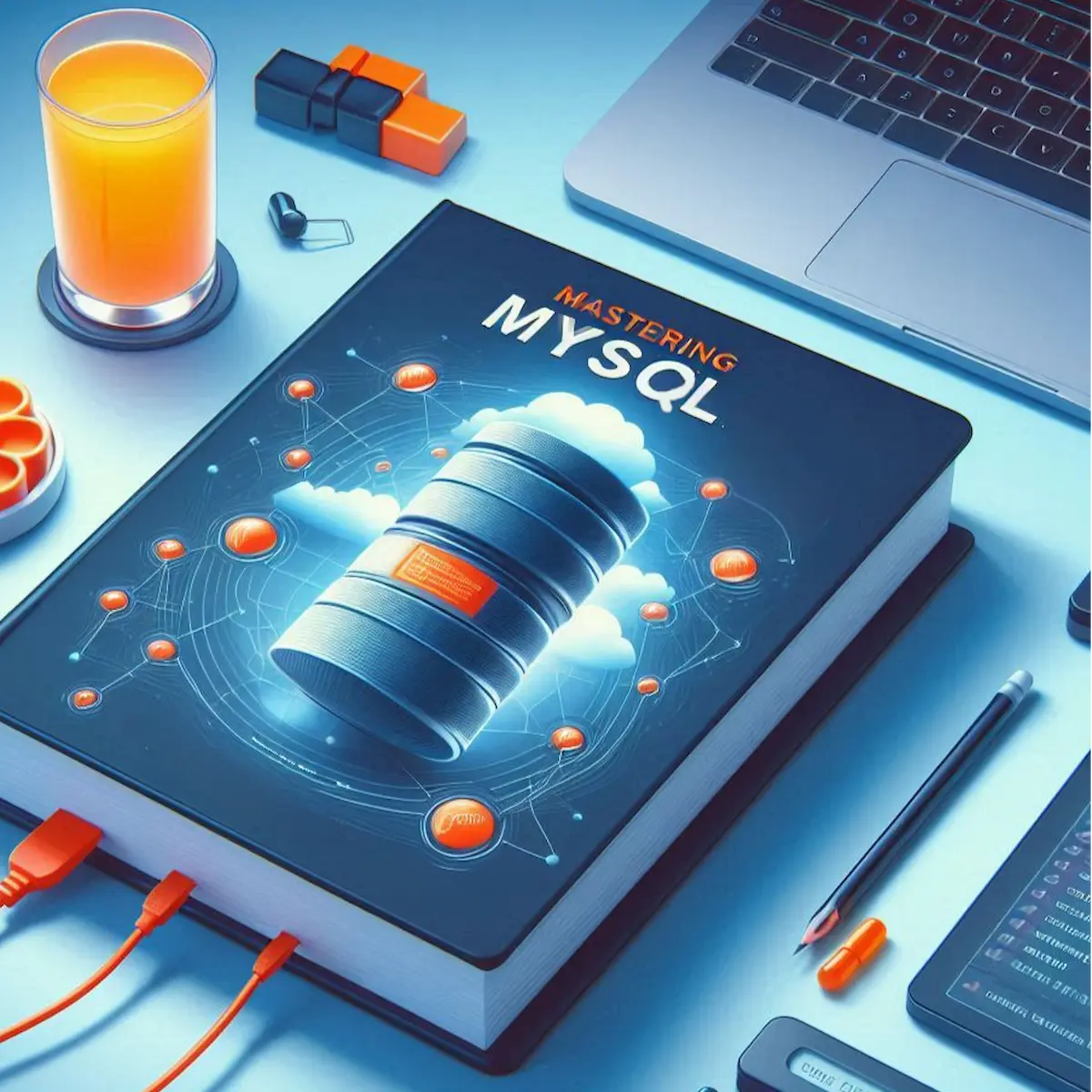
SQL Basics for MySQL: A Beginner's Guide
- Ctrl Man
- Database , Programming
- 12 Jul, 2024
SQL Basics for MySQL: A Beginner’s Guide
Introduction to SQL and MySQL
SQL, which stands for Structured Query Language, is a domain-specific language used in managing and manipulating databases. It is designed to interact with databases like MySQL and Oracle. Learning SQL is essential for anyone looking to work with databases as it allows you to create, manage, and manipulate data efficiently.
MySQL, on the other hand, is an open-source relational database management system that provides high-level data integrity and concurrency control features. In this article, we’ll dive into some fundamental SQL concepts using MySQL as our example. By the end of this guide, you’ll be able to create tables, insert data, query your database, update records, and delete data.
Creating Tables (CREATE TABLE)
Creating a table in MySQL involves defining the structure of your database by specifying columns, their types, and constraints such as primary keys or unique values. Tables are the backbone of any database, storing the actual data in a structured format.
-- Create a simple 'employees' table with an auto-incrementing ID as the primary key.
CREATE TABLE employees (
id INT AUTO_INCREMENT PRIMARY KEY,
first_name VARCHAR(100) NOT NULL,
last_name VARCHAR(100) NOT NULL,
email VARCHAR(255) UNIQUE NOT NULL
);
In this example:
id
is an auto-incrementing integer serving as the primary key.first_name
andlast_name
are variable character fields, both mandatory (NOT NULL
).email
is a unique and mandatory variable character field.
Inserting Data (INSERT INTO)
After creating a table, you might want to populate it with data. The INSERT INTO
command is used to add rows of data into your database. Ensuring data integrity during insertion is crucial, and constraints defined during table creation are enforced at this stage.
-- Insert an employee record.
INSERT INTO employees (first_name, last_name, email)
VALUES ('John', 'Doe', 'john.doe@example.com');
This command inserts a new row into the employees
table with the specified values for first_name
, last_name
, and email
.
Querying Data (SELECT)
Select queries are used to fetch rows of data from a database. They can filter and sort the results based on specific conditions. The SELECT
statement is powerful for data retrieval and analysis, allowing you to specify exactly what data you need.
-- Select all employees with emails containing "example.com".
SELECT * FROM employees WHERE email LIKE '%@example.com';
This query retrieves all columns for employees whose email addresses contain “example.com”.
Updating Data (UPDATE)
The UPDATE
command allows you to modify existing data in a database, provided certain conditions are met. Updating data must be done carefully to ensure only the intended records are modified.
Example Code
-- Update the email of John Doe.
UPDATE employees SET email = 'new.email@example.com' WHERE first_name = 'John' AND last_name = 'Doe';
This command updates the email address for the employee named John Doe. The use of AND
ensures the update is applied to the correct record.
Deleting Data (DELETE)
The DELETE
command is used to remove rows from your database. It’s important to use this command carefully, as it permanently removes data. Always back up your database before performing delete operations to avoid losing important information unintentionally.
Example Code
-- Delete the record of John Doe.
DELETE FROM employees WHERE first_name = 'John' AND last_name = 'Doe';
This command deletes the record of John Doe from the employees
table.
Conclusion
Understanding basic SQL commands like CREATE TABLE
, INSERT INTO
, SELECT
, UPDATE
, and DELETE
is crucial for managing databases in MySQL. These commands form the backbone of database operations, allowing you to efficiently manipulate your data.
Remember, always back up your database before performing any delete operations to avoid losing important information unintentionally. As you continue learning, explore more advanced SQL concepts and commands to enhance your database management skills.
Happy coding!