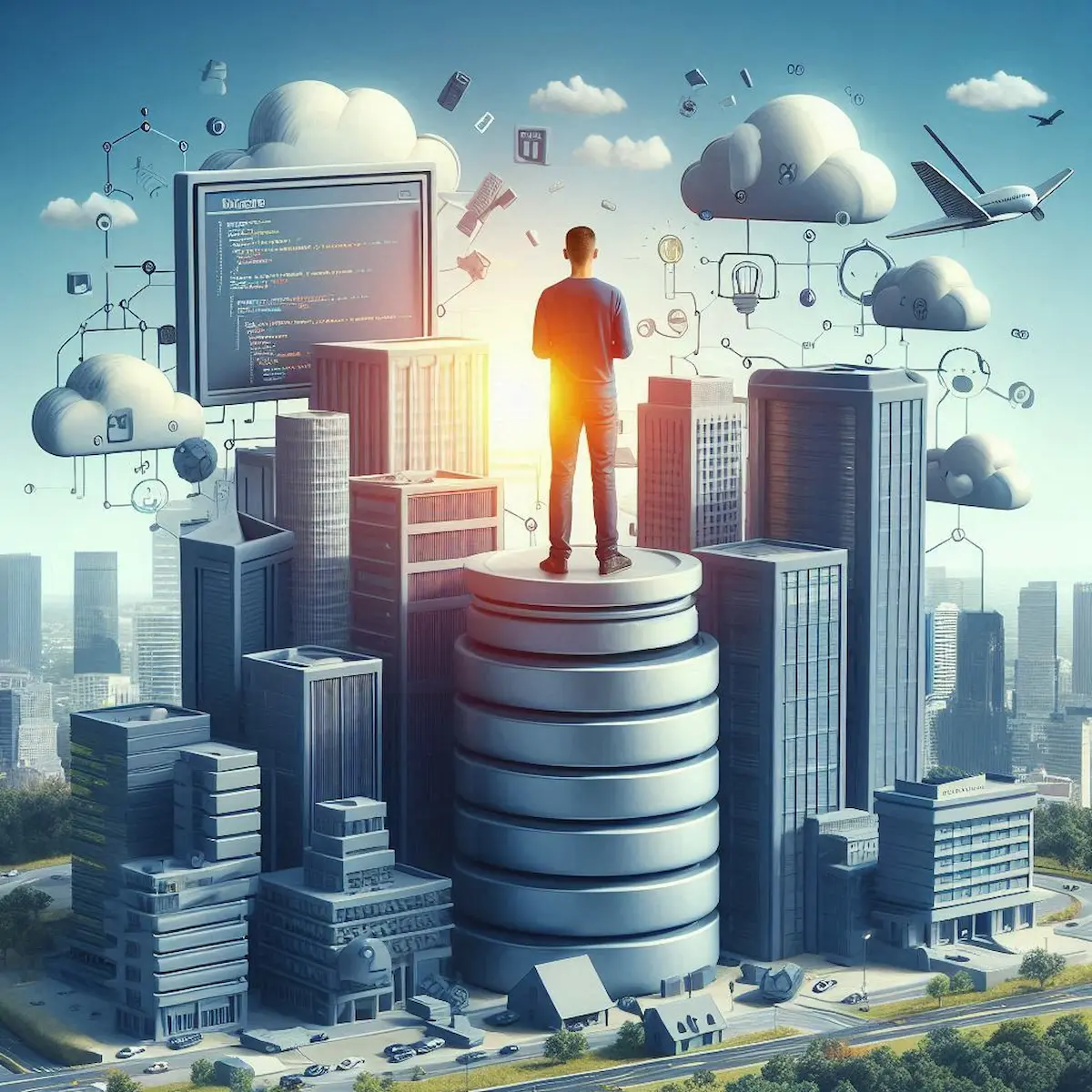
Mastering MySQL Integration in Modern Application Development
- Tech Explorer
- Database , Application Development , Programming
- 27 Aug, 2024
Mastering MySQL Integration in Modern Application Development
Connecting to MySQL from Different Programming Languages
Introduction
In today’s fast-paced world of application development, seamlessly integrating a robust database system is paramount for efficient data management. MySQL, a cornerstone in the realm of relational database management systems (RDBMS), offers versatile connectivity options across multiple programming languages. This comprehensive guide delves into the intricacies of connecting various programming languages with MySQL databases, empowering developers to create powerful, data-driven applications.
Connecting with C#
C# developers can harness the power of the MySql.Data
library to establish a strong connection with MySQL databases:
using MySql.Data.MySqlClient;
public class DatabaseConnection
{
private readonly MySqlConnection _connection;
private readonly string _connectionString;
public DatabaseConnection(string server, string database, string username, string password)
{
_connectionString = $"Server={server};Database={database};Uid={username};Pwd={password};";
_connection = new MySqlConnection(_connectionString);
}
public void Connect()
{
try
{
_connection.Open();
Console.WriteLine("Successfully connected to the database!");
}
catch (MySqlException ex)
{
Console.WriteLine($"Error connecting to the database: {ex.Message}");
}
}
public void ExecuteQuery(string query)
{
using var command = new MySqlCommand(query, _connection);
using var reader = command.ExecuteReader();
while (reader.Read())
{
// Process each row of the result
}
}
public void Dispose()
{
_connection.Close();
}
}
public class Program
{
static void Main()
{
using var db = new DatabaseConnection("localhost", "mydatabase", "root", "");
db.Connect();
db.ExecuteQuery("SELECT * FROM users");
}
}
Connecting with Python
Python offers a sleek interface to MySQL through libraries like mysql-connector-python
:
import mysql.connector
from mysql.connector import Error
def connect_to_db(host, database, user, password):
try:
connection = mysql.connector.connect(
host=host,
database=database,
user=user,
password=password
)
if connection.is_connected():
print("Successfully connected to MySQL database")
return connection
except Error as e:
print(f"Error connecting to MySQL database: {e}")
return None
def execute_query(connection, query):
try:
cursor = connection.cursor()
cursor.execute(query)
result = cursor.fetchall()
return result
except Error as e:
print(f"Error executing query: {e}")
finally:
if connection.is_connected():
cursor.close()
# Example usage:
connection = connect_to_db('localhost', 'mydatabase', 'root', '')
if connection:
results = execute_query(connection, "SELECT * FROM users")
for row in results:
print(row)
connection.close()
Connecting with Java
Java developers can leverage the mysql-connector-java
library for robust MySQL interactions:
import java.sql.*;
public class DatabaseConnection {
private Connection connection;
private final String url;
private final String user;
private final String password;
public DatabaseConnection(String url, String user, String password) {
this.url = url;
this.user = user;
this.password = password;
}
public void connect() throws SQLException {
connection = DriverManager.getConnection(url, user, password);
System.out.println("Connected to database successfully!");
}
public ResultSet executeQuery(String query) throws SQLException {
Statement statement = connection.createStatement();
return statement.executeQuery(query);
}
public void close() throws SQLException {
if (connection != null && !connection.isClosed()) {
connection.close();
}
}
public static void main(String[] args) {
DatabaseConnection db = new DatabaseConnection(
"jdbc:mysql://localhost/mydatabase", "root", ""
);
try {
db.connect();
ResultSet rs = db.executeQuery("SELECT * FROM users");
while (rs.next()) {
// Process each row
System.out.println(rs.getString("username"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
db.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
Connecting with JavaScript (Node.js)
Node.js developers can utilize the mysql2
library for efficient MySQL operations:
const mysql = require('mysql2/promise');
async function connectToDatabase() {
try {
const connection = await mysql.createConnection({
host: 'localhost',
user: 'root',
password: '',
database: 'mydatabase'
});
console.log("Successfully connected to MySQL!");
return connection;
} catch (error) {
console.error('Error connecting to the database:', error);
throw error;
}
}
async function executeQuery(connection, query) {
try {
const [rows, fields] = await connection.execute(query);
return rows;
} catch (error) {
console.error('Error executing query:', error);
throw error;
}
}
// Example usage:
(async () => {
let connection;
try {
connection = await connectToDatabase();
const results = await executeQuery(connection, 'SELECT * FROM users');
console.log(results);
} catch (error) {
// Handle any errors
} finally {
if (connection) {
await connection.end();
}
}
})();
Best Practices for Database Interaction in Applications
1. Optimize Queries
-
Use indexes wisely: Improve data retrieval speed by adding appropriate indexes.
CREATE INDEX idx_username ON users(username);
-
Write efficient queries: Avoid full table scans by targeting specific columns and using
WHERE
clauses effectively.-- Good SELECT username FROM users WHERE user_id = 1; -- Avoid SELECT * FROM users;
2. Implement Transactions
Ensure data integrity with proper transaction management:
START TRANSACTION;
INSERT INTO orders (user_id, product_id, quantity) VALUES (1, 100, 2);
UPDATE inventory SET stock = stock - 2 WHERE product_id = 100;
COMMIT;
3. Parameterize Queries
Prevent SQL injection attacks by using parameterized queries:
using (var command = new MySqlCommand("SELECT * FROM users WHERE username = @username", connection))
{
command.Parameters.AddWithValue("@username", userInput);
// Execute command
}
cursor.execute("SELECT * FROM users WHERE username = %s", (username,))
PreparedStatement pstmt = conn.prepareStatement("SELECT * FROM users WHERE username = ?");
pstmt.setString(1, username);
ResultSet rs = pstmt.executeQuery();
const [rows] = await connection.execute('SELECT * FROM users WHERE username = ?', [username]);
4. Connection Pooling
Implement connection pooling to manage database connections efficiently:
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
public class ConnectionPool {
private static HikariConfig config = new HikariConfig();
private static HikariDataSource ds;
static {
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase");
config.setUsername("root");
config.setPassword("");
config.addDataSourceProperty("cachePrepStmts", "true");
config.addDataSourceProperty("prepStmtCacheSize", "250");
config.addDataSourceProperty("prepStmtCacheSqlLimit", "2048");
ds = new HikariDataSource(config);
}
public static Connection getConnection() throws SQLException {
return ds.getConnection();
}
}
5. Error Handling and Logging
Implement robust error handling and logging mechanisms:
import logging
logging.basicConfig(filename='app.log', level=logging.ERROR)
try:
# Database operations
except mysql.connector.Error as err:
logging.error(f"Database error: {err}")
# Handle the error appropriately
Advanced Topics
1. Database Migrations
Use tools like Flyway or Liquibase to manage database schema changes:
<!-- pom.xml for Flyway -->
<dependency>
<groupId>org.flywaydb</groupId>
<artifactId>flyway-core</artifactId>
<version>7.15.0</version>
</dependency>
2. ORM (Object-Relational Mapping)
Consider using ORMs like Hibernate (Java), Entity Framework (C#), or SQLAlchemy (Python) for more complex applications:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
username = Column(String(50), unique=True)
email = Column(String(120), unique=True)
engine = create_engine('mysql://root:@localhost/mydatabase')
Session = sessionmaker(bind=engine)
# Usage
session = Session()
new_user = User(username='johndoe', email='john@example.com')
session.add(new_user)
session.commit()
Conclusion
Mastering MySQL integration across various programming languages is a crucial skill in modern application development. By following these best practices, implementing robust error handling, and leveraging advanced concepts like connection pooling and ORMs, developers can create scalable, efficient, and secure applications that harness the full power of MySQL databases.
Remember, the key to successful database integration lies not just in connecting to the database, but in doing so securely, efficiently, and in a way that scales with your application’s needs. Continue exploring these concepts, stay updated with the latest MySQL features, and always prioritize data integrity and security in your development practices.