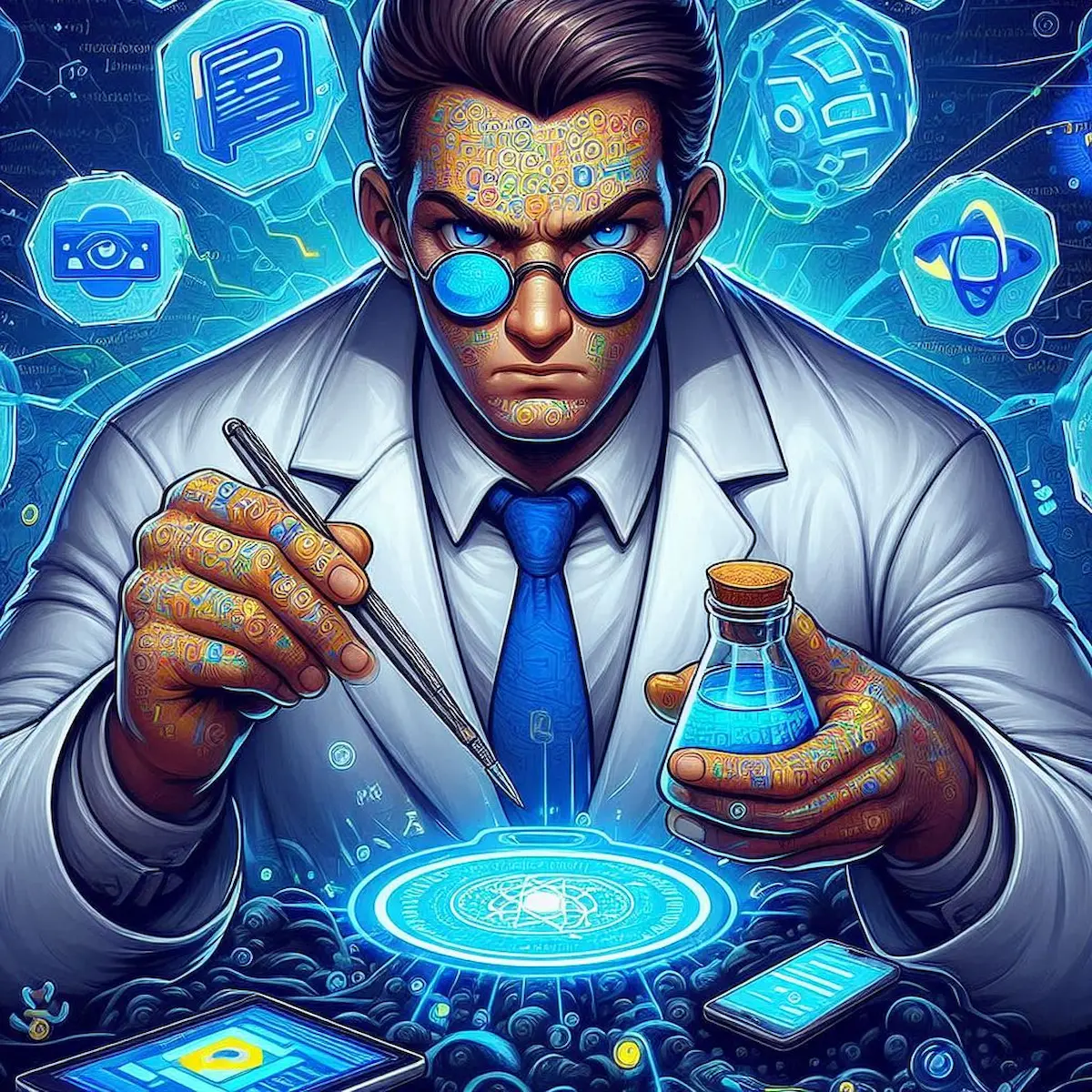
Mastering useCallback in React: Optimizing Function Management
- Ctrl Man
- Web Development , JavaScript Frameworks
- 30 Jul, 2024
Mastering useCallback
in React: A Beginner’s Guide to Optimizing Function Management
Introduction
In the dynamic world of React development, performance optimization is key to creating smooth, responsive applications. One powerful tool in your React toolkit is the useCallback
hook. This guide will demystify useCallback
, explaining its importance, usage, and best practices for beginners looking to level up their React skills.
What is useCallback?
useCallback
is a React hook that allows you to memoize (or cache) function definitions across multiple renders of your component. Instead of recreating the same function on every render, it maintains a consistent function reference as long as its dependencies remain unchanged.
Why Use useCallback?
1. Performance Optimization
useCallback
can significantly improve your app’s performance by:
- Preventing unnecessary re-renders when passing functions to child components
- Optimizing components wrapped with
React.memo
- Reducing the overhead of function creation on each render
2. Dependency Control
With useCallback
, you have explicit control over when a memoized function should be updated. This ensures that your application doesn’t suffer from unintended re-rendering due to function changes.
How to Use useCallback
Let’s look at a practical example:
import React, { useState, useCallback } from 'react';
function Counter() {
const [count, setCount] = useState(0);
// Memoize the increment function
const handleIncrement = useCallback(() => {
setCount((prevCount) => prevCount + 1);
}, []); // Empty dependency array means this function never changes
return (
<div>
<p>Count: {count}</p>
<ChildComponent onIncrement={handleIncrement} />
</div>
);
}
// A memoized child component
const ChildComponent = React.memo(({ onIncrement }) => {
console.log("ChildComponent rendered");
return <button onClick={onIncrement}>Increment</button>;
});
In this example, handleIncrement
is memoized and only created once, regardless of how many times Counter
re-renders.
Best Practices and Considerations
-
Use Sparingly: Don’t wrap every function in
useCallback
. Use it when passing functions as props, especially to memoized child components. -
Manage Dependencies Carefully: Include all values in the dependency array that the function uses from the component’s scope. This ensures the function updates when necessary.
-
Combine with useMemo: For complex calculations, consider using
useMemo
alongsideuseCallback
to memoize both functions and values. -
Profile Performance: Use React DevTools to measure the impact of
useCallback
on your app’s performance.
Advanced Techniques: Managing Hooks Effectively
As your React projects grow, managing hooks becomes increasingly important. Here are some advanced techniques to consider:
1. Custom Hooks
Create reusable custom hooks to encapsulate related logic:
function useCounter(initialValue = 0) {
const [count, setCount] = useState(initialValue);
const increment = useCallback(() => setCount(c => c + 1), []);
const decrement = useCallback(() => setCount(c => c - 1), []);
return { count, increment, decrement };
}
2. State Management Libraries
For complex applications, consider using state management libraries:
- Redux or MobX: Centralize global state management
- Recoil: Manage atomic state with a flexible API
- Zustand: A minimalistic state management solution
- XState: Use state machines for complex state logic
3. Performance Profiling
Utilize React’s built-in Profiler or browser developer tools to identify performance bottlenecks and optimize hook usage accordingly.
Conclusion
Mastering useCallback
is a crucial step towards building efficient React applications. By understanding its role in optimizing performance through function memoization and controlling dependencies, you can significantly enhance your code quality and application performance.
Remember, the key to effective use of useCallback
and other React hooks lies not just in using them, but in applying them judiciously based on your specific project needs. Happy coding!