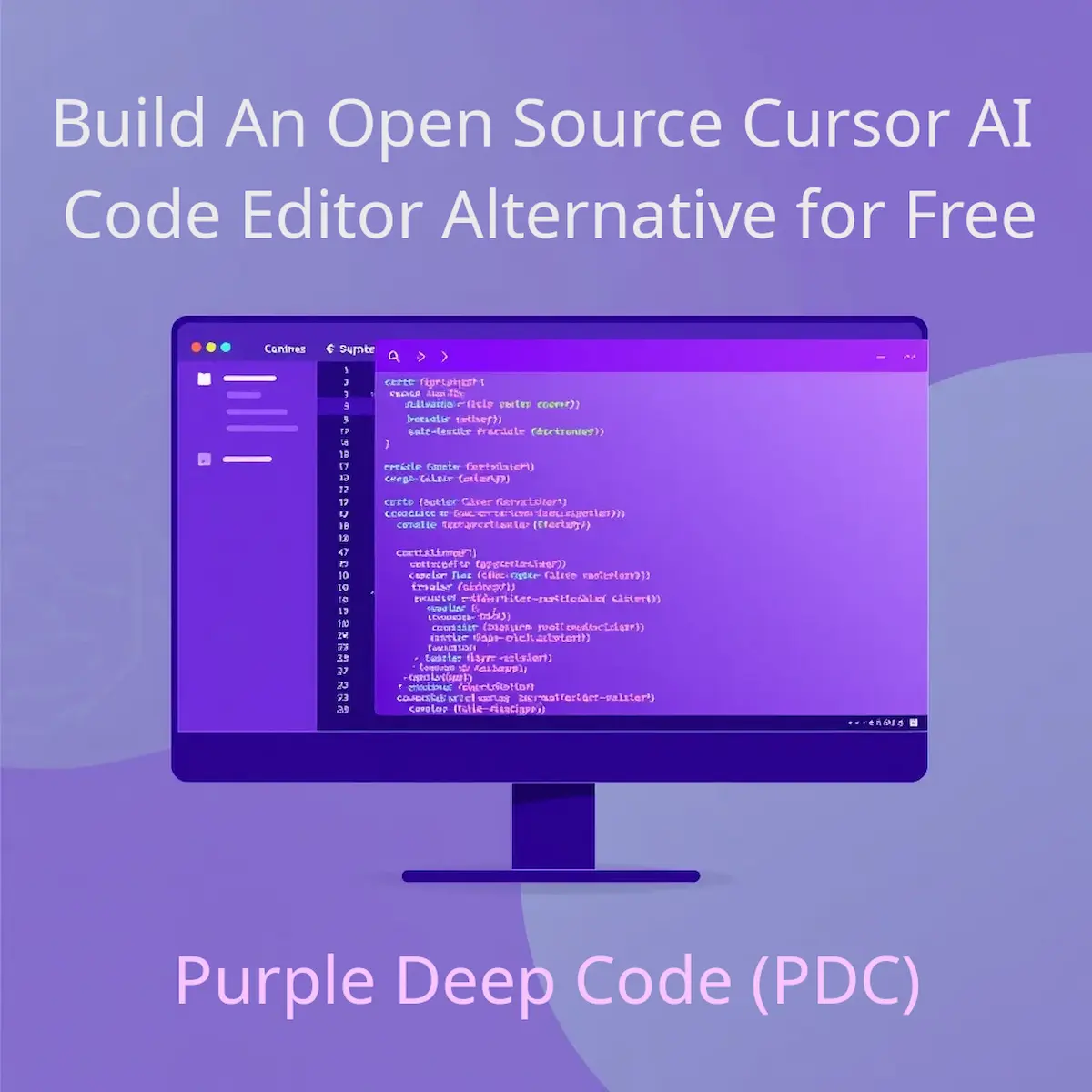
Building PurpleDeepCode: Your Open-Source AI-Powered Code Editor
- Ctrl Man
- Technology , Software Development , AI
- 02 Oct, 2024
Building PurpleDeepCode: Your Open-Source AI-Powered Code Editor
1. Introduction
In today’s fast-paced world of software development, AI-powered code editors like Cursor and PearAI have gained significant traction. However, what if you could create your own open-source alternative, tailored to your specific needs and available for everyone to use? Enter PurpleDeepCode (PDC) – a Visual Studio Code fork that integrates powerful language models and sports a sleek purple theme. By following this article, you’ll learn how to build your very own AI-enhanced code editor.
Benefits of AI in Code Editing:
- Increased productivity
- Better code quality
- Fewer errors
2. Setting the Stage: Forking Visual Studio Code
The first step in our journey is to create a fork of Visual Studio Code. This gives us a solid foundation to build upon, leveraging VSCode’s robust architecture and extensive feature set.
git clone https://github.com/microsoft/vscode.git
cd vscode
npm install
Background on Forking:
Forking VSCode allows you to have a local copy of the editor that you can modify and customize without affecting the original project. This ensures you have all the necessary dependencies installed, including Node.js, npm, and other development tools.
2.1 Ethical Considerations in Forking Open-Source AI Tools
The AI development space is highly competitive, and the boundary between innovation and replication can be blurry, especially with open-source projects. This was made evident recently when the AI coding editor PearAI, which was incubated by Y Combinator, faced significant backlash. PearAI openly admitted to cloning another AI tool, Continue, which itself is a fork of the popular VSCode. PearAI’s founder, Duke Pan, initially released the project with a closed-source license, which was later revealed to have been generated by AI. This caused outrage within the open-source community, as the original Continue project is licensed under the Apache open-source license.
After the uproar, Pan apologized and shifted PearAI back to the proper Apache license, acknowledging the misstep. However, the damage had already been done. Critics, including other developers and open-source advocates, felt the project had overstepped by failing to clearly acknowledge its origins from Continue, leading to accusations of dishonesty and exploitation of the open-source ecosystem.
Y Combinator’s involvement in the project further fueled the controversy, with detractors questioning the accelerator’s due diligence. Some voiced concerns that this case reflected a larger trend of VCs funding AI projects without proper oversight, particularly regarding licensing and intellectual property. PearAI’s misstep serves as a reminder of the ethical and legal challenges that can arise when working with or forking open-source projects.
For developers and startups working with open-source AI tools, transparency and respect for intellectual property are essential. Projects like PearAI highlight the importance of not only building on existing work but also making meaningful contributions to the open-source community, rather than simply replicating and rebranding existing tools. *
3. Painting it Purple: Customizing the UI
To give PurpleDeepCode its distinct identity, we’ll create a custom purple theme by modifying the source code before compiling. Here’s how you can add a new color theme to the forked version of VSCode:
Editing the Theme in the Forked Source Code
a. Navigate to the Themes Directory: In the forked VSCode repository, find the directory where default themes are stored. The typical path for this is:
extensions/theme-defaults/themes/
b. Create a New Theme File: Inside the themes/
folder, create a new JSON file for your custom theme, such as purpledeepcode-color-theme.json
. This file will contain the color configuration for your theme. Here’s an example:
{
"name": "PurpleDeepCode",
"type": "dark",
"colors": {
"editor.background": "#241F3E",
"sideBar.background": "#1C1826",
"activityBar.background": "#1D1927",
"statusBar.background": "#1B1A25",
"list.activeSelectionBackground": "#612FA4",
"editor.foreground": "#F2EAE5"
}
}
c. Modify the package.json
in the theme-defaults
Extension: After creating the theme file, you need to register it in the appropriate package.json
file inside the theme-defaults
extension. Navigate to the extensions/theme-defaults/
directory and open the package.json
file. Add your new theme to the contributes
section like so:
{
"name": "theme-defaults",
"displayName": "Default Themes",
"description": "Default color themes for VSCode",
"version": "1.0.0",
"publisher": "Microsoft",
"engines": {
"vscode": "^1.50.0"
},
"contributes": {
"themes": [
{
"label": "PurpleDeepCode",
"uiTheme": "vs-dark",
"path": "./themes/purpledeepcode-color-theme.json"
}
]
}
}
d. Compile the Forked Version: After making these changes, you’ll need to recompile your forked version of VSCode to apply the new theme. Follow the typical steps for compiling VSCode from source (such as running yarn
or npm run compile
depending on your setup).
e. Test Your Theme: Once your forked version is compiled, launch it and navigate to the Color Theme selection in the settings (Ctrl+K Ctrl+T
). Your PurpleDeepCode theme should now be available in the list of themes.
Why This Approach?
By following these steps, you’re directly modifying the theme configuration in the source code of the forked version of VSCode. This method ensures that your custom theme is available by default in your fork, eliminating the need to install or manage it as an external extension.
4. Integrating AI: Setting Up a Local Language Model Server
The heart of PurpleDeepCode lies in its AI capabilities. We’ll set up a local server to host our language models, starting with Qwen2.5-coder-7B and Qwen2.5-coder-1.5B.
const express = require('express');
const app = express();
const port = 3000;
app.get('/api/qwen2.5-coder-7B', (req, res) => {
res.json({ message: 'Response from Qwen2.5-coder-7B' });
});
app.get('/api/qwen2.5-coder-1.5B', (req, res) => {
res.json({ message: 'Response from Qwen2.5-coder-1.5B' });
});
app.listen(port, () => {
console.log(`AI Model Server running at http://localhost:${port}`);
});
Explanation of Models: Qwen2.5-coder models are designed for specific tasks within coding, making them ideal for integrating into an AI-powered code editor. The local server allows you to experiment with these models without the need for constant API calls.
5. Bridging the Gap: Integrating AI with Continue.dev
To bring AI capabilities into our editor, we’ll use the Continue.dev extension. This powerful tool allows us to leverage locally hosted models through Ollama and LM Studio, providing a flexible and customizable AI-powered coding experience.
Setting up Continue extension
First, install the Continue.dev extension in your VSCode fork. Then, configure it to use Qwen2.5-coder as the base model for general tasks and Llama3.2-1b for autocompletion. Here’s an example config.json
for the Continue extension:
{
"models": [
{
"title": "LM Studio",
"provider": "lmstudio",
"model": "qwen2-7b"
}
],
"customCommands": [
{
"name": "test",
"prompt": "{{{ input }}}\n\nWrite a comprehensive set of unit tests for the selected code. It should setup, run tests that check for correctness including important edge cases, and teardown. Ensure that the tests are complete and sophisticated. Give the tests just as chat output, don’t edit any file.",
"description": "Write unit tests for highlighted code"
}
],
"tabAutocompleteModel": {
"title": "llama3.2:1b",
"model": "llama3.2:1b",
"provider": "ollama"
},
"contextProviders": [
{
"name": "code",
"params": {}
},
{
"name": "docs",
"params": {}
},
{
"name": "diff",
"params": {}
},
{
"name": "terminal",
"params": {}
},
{
"name": "problems",
"params": {}
},
{
"name": "folder",
"params": {}
},
{
"name": "codebase",
"params": {}
}
],
"slashCommands": [
{
"name": "edit",
"description": "Edit selected code"
},
{
"name": "comment",
"description": "Write comments for the selected code"
},
{
"name": "share",
"description": "Export the current chat session to markdown"
},
{
"name": "cmd",
"description": "Generate a shell command"
},
{
"name": "commit",
"description": "Generate a git commit message"
}
],
"embeddingsProvider": {
"provider": "transformers.js"
}
}
Role of Context Providers:
Context providers enhance the AI’s understanding of your project. By including codebase-level context, you help the model generate more accurate and relevant suggestions.
Implementing AI Features
With Continue.dev configured, we can now easily implement AI-powered features in PurpleDeepCode. For example, to add a command that generates unit tests for selected code:
import * as vscode from 'vscode';
export function activate(context: vscode.ExtensionContext) {
let disposable = vscode.commands.registerCommand('purpledeepcode.generateTests', async () => {
const editor = vscode.window.activeTextEditor;
if (editor) {
const selection = editor.selection;
const selectedCode = editor.document.getText(selection);
// This will trigger the Continue.dev extension to use the 'test' custom command
await vscode.commands.executeCommand('continue.chat', `/test ${selectedCode}`);
}
});
context.subscriptions.push(disposable);
}
Explanation of Code:
This implementation leverages the power of Continue.dev and our configured models to generate sophisticated unit tests based on the selected code. The custom command /test
is triggered when the user runs the purpledeepcode.generateTests
command.
6. Optimizing for Performance
To ensure PurpleDeepCode runs smoothly on budget Windows machines, we need to focus on optimization. This includes efficient data structures, lazy loading of features, and careful memory management.
// Example of lazy loading a heavy feature
let heavyFeature: any;
export async function activateHeavyFeature() {
if (!heavyFeature) {
heavyFeature = await import('./heavyFeature');
}
heavyFeature.activate();
}
// Use when needed
vscode.commands.registerCommand('purpledeepcode.useHeavyFeature', activateHeavyFeature);
Optimization Strategies:
Lazy loading is a great way to improve performance by deferring the initialization of heavy features until they are actually needed. This ensures that your editor remains responsive, even on budget machines.
7. Packaging and Distribution
Finally, we need to package our creation for easy distribution. We can use Electron Builder to create an executable for Windows.
{
"scripts": {
"dist": "electron-builder"
},
"build": {
"appId": "com.yourcompany.purpledeepcode",
"productName": "PurpleDeepCode",
"win": {
"target": [
"nsis"
]
}
}
}
Cross-Platform Distribution:
Electron Builder supports cross-platform distribution, making it easy to create executables for macOS and Linux. Ensure you handle code signing for Windows applications to avoid issues with unsigned executables.
Conclusion
Building PurpleDeepCode, an open-source alternative to AI-powered code editors, is an exciting journey that combines the robust foundation of Visual Studio Code with cutting-edge AI capabilities. By following these steps, you can create a powerful, customized coding environment that’s free for everyone to use and contribute to.
Benefits of Open-Source Collaboration:
- Enhanced community contributions
- Continuous improvements and bug fixes
- A larger pool of developers contributing to your project
Remember, the true power of open-source lies in community contributions. As you develop PurpleDeepCode, consider how you can foster a community around your project, encouraging others to contribute features, optimizations, and improvements.
Happy coding, and may your PurpleDeepCode light the way to more efficient and intelligent software development!