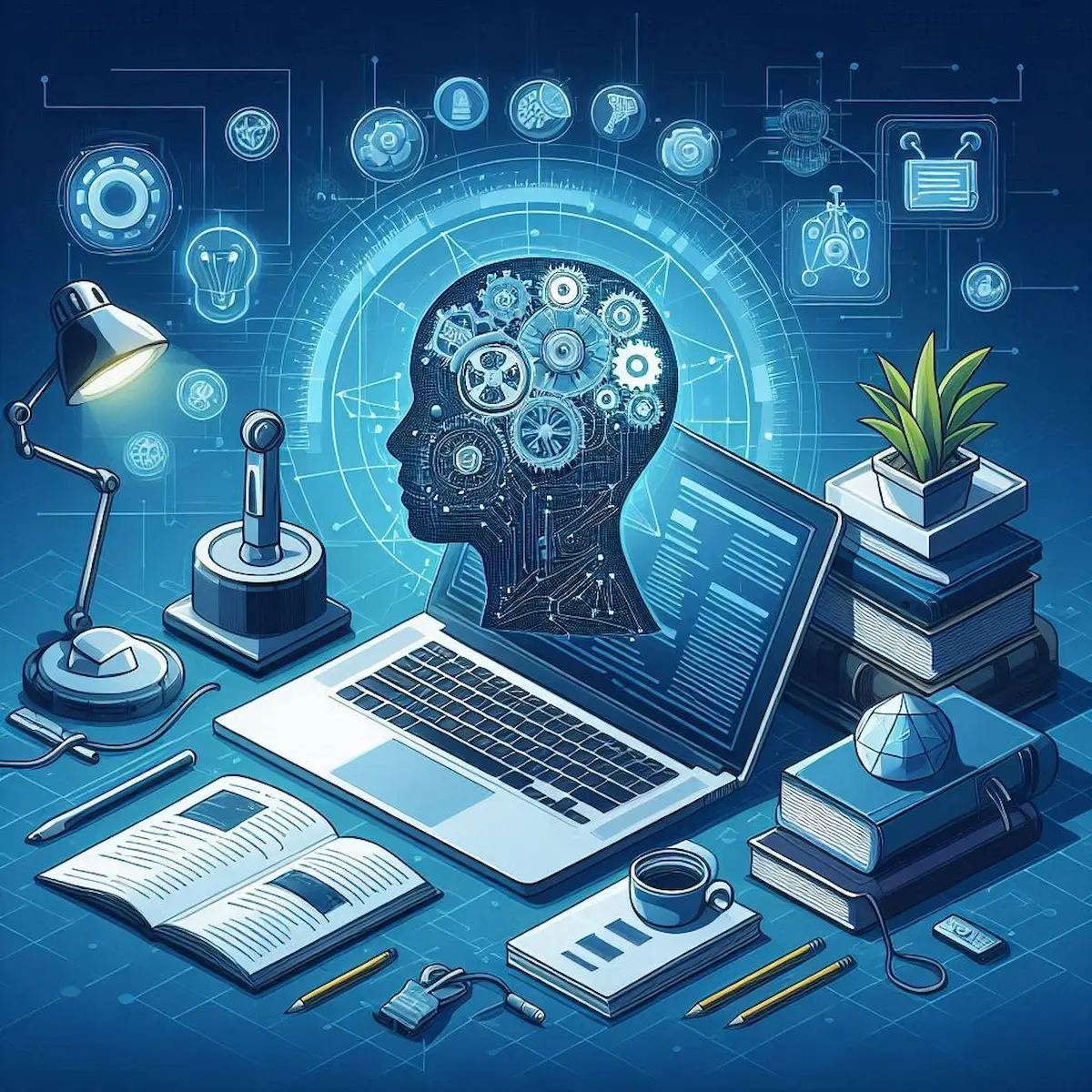
Web Development Mastery: A Comprehensive Guide for Beginners
- Ctrl Man
- Web Development , Programming , Technology
- 05 Jul, 2024
Web Development Mastery: A Comprehensive Guide for Beginners
Unlocking the World of Web Creation
Welcome to the exciting realm of web development! Whether you’re a coding novice or an experienced programmer looking to expand your skill set, this guide will equip you with the knowledge and tools to create captivating websites and web applications. Let’s embark on this journey together, exploring the fundamental concepts, cutting-edge technologies, and industry best practices that will transform you into a proficient web developer.
1. Crafting Your Development Sanctuary
Before diving into code, it’s crucial to set up a conducive development environment. Here’s how to create your digital workspace:
-
Choose Your Code Editor: Select a powerful and user-friendly Integrated Development Environment (IDE) or code editor. Popular choices include:
- Visual Studio Code: Feature-rich and highly customizable
- Sublime Text: Lightning-fast and lightweight
- JetBrains WebStorm: Robust IDE with advanced features (paid)
-
Master Dependency Management: Install Node.js to leverage npm (Node Package Manager) or Yarn for efficient JavaScript package management.
-
Embrace Version Control: Learn Git basics and choose a hosting service like GitHub or GitLab for seamless collaboration and code backup.
-
Configure Local Servers: Set up development servers to test your projects:
- Mac: MAMP
- Windows: XAMPP
- Cross-platform: Node.js with Express
2. HTML & CSS: Building the Foundation
HTML (Hypertext Markup Language) and CSS (Cascading Style Sheets) form the cornerstone of web development. Let’s explore these essential technologies:
HTML Essentials
- Document structure:
<!DOCTYPE html>
,<html>
,<head>
, and<body>
- Semantic elements:
<header>
,<nav>
,<main>
,<article>
,<aside>
,<footer>
- Text formatting:
<h1>
to<h6>
,<p>
,<strong>
,<em>
- Lists:
<ul>
,<ol>
,<li>
- Links and images:
<a>
,<img>
- Forms:
<form>
,<input>
,<textarea>
,<button>
CSS Mastery
- Selectors: Element, class, ID, attribute, and pseudo-selectors
- Box model:
margin
,border
,padding
,width
,height
- Typography:
font-family
,font-size
,font-weight
,line-height
- Colors and backgrounds
- Layout techniques: Flexbox and CSS Grid
- Responsive design with media queries
Hands-On Project: Portfolio Website
Apply your HTML and CSS skills by creating a responsive portfolio website showcasing your projects, skills, and contact information.
3. JavaScript: Bringing Interactivity to Life
JavaScript empowers you to create dynamic, interactive web experiences. Master these fundamental concepts:
- Variables, data types, and operators
- Control structures:
if/else
,switch
, loops (for
,while
) - Functions and scope
- Arrays and objects
- DOM manipulation
- Event handling
- Asynchronous programming with Promises and async/await
- ES6+ features: Arrow functions, template literals, destructuring
Interactive Project: To-Do List Application
Develop a feature-rich to-do list app using vanilla JavaScript, demonstrating DOM manipulation, event handling, and local storage integration.
4. Advanced Web Technologies
Elevate your skills with modern frameworks and tools:
Frontend Frameworks
-
React:
- Component-based architecture
- Virtual DOM for optimized rendering
- State management with hooks
- React Router for navigation
-
Vue.js:
- Progressive framework with gentle learning curve
- Template syntax and reactive data binding
- Vue CLI for rapid project setup
- Vuex for state management
Backend Technologies
-
Node.js and Express:
- Server-side JavaScript
- RESTful API development
- Database integration (MongoDB, PostgreSQL)
- Authentication and authorization
-
GraphQL:
- Efficient data querying and manipulation
- Schema definition and resolvers
- Integration with frontend frameworks
5. Deployment and DevOps
Bring your creations to life with modern deployment strategies:
-
Version Control Workflow:
- Git branching strategies (e.g., Git Flow)
- Pull requests and code reviews
- Continuous Integration (CI) with GitHub Actions or GitLab CI
-
Containerization:
- Docker basics for consistent environments
- Docker Compose for multi-container applications
-
Cloud Deployment:
- Platform as a Service (PaaS): Heroku, Netlify
- Infrastructure as a Service (IaaS): AWS, Google Cloud Platform, DigitalOcean
-
Continuous Deployment (CD):
- Automated testing and deployment pipelines
- Blue-green deployments for zero-downtime updates
6. Web Security Best Practices
Protect your applications and users with these security measures:
- HTTPS implementation with SSL/TLS certificates
- Cross-Site Scripting (XSS) prevention
- SQL injection safeguards
- Cross-Site Request Forgery (CSRF) protection
- Security headers configuration
- Regular dependency audits and updates
Conclusion: Your Web Development Journey Begins
Congratulations on taking your first steps into the vast world of web development! Remember, the key to mastery is consistent practice and a passion for learning. As you progress, consider these next steps:
- Build a diverse project portfolio to showcase your skills
- Contribute to open-source projects on GitHub
- Attend web development meetups and conferences
- Stay updated with the latest trends and technologies through blogs and podcasts
- Consider specializing in areas like progressive web apps (PWAs) or serverless architectures
With dedication and creativity, you’ll soon be crafting extraordinary web experiences that captivate users and solve real-world problems. Happy coding!