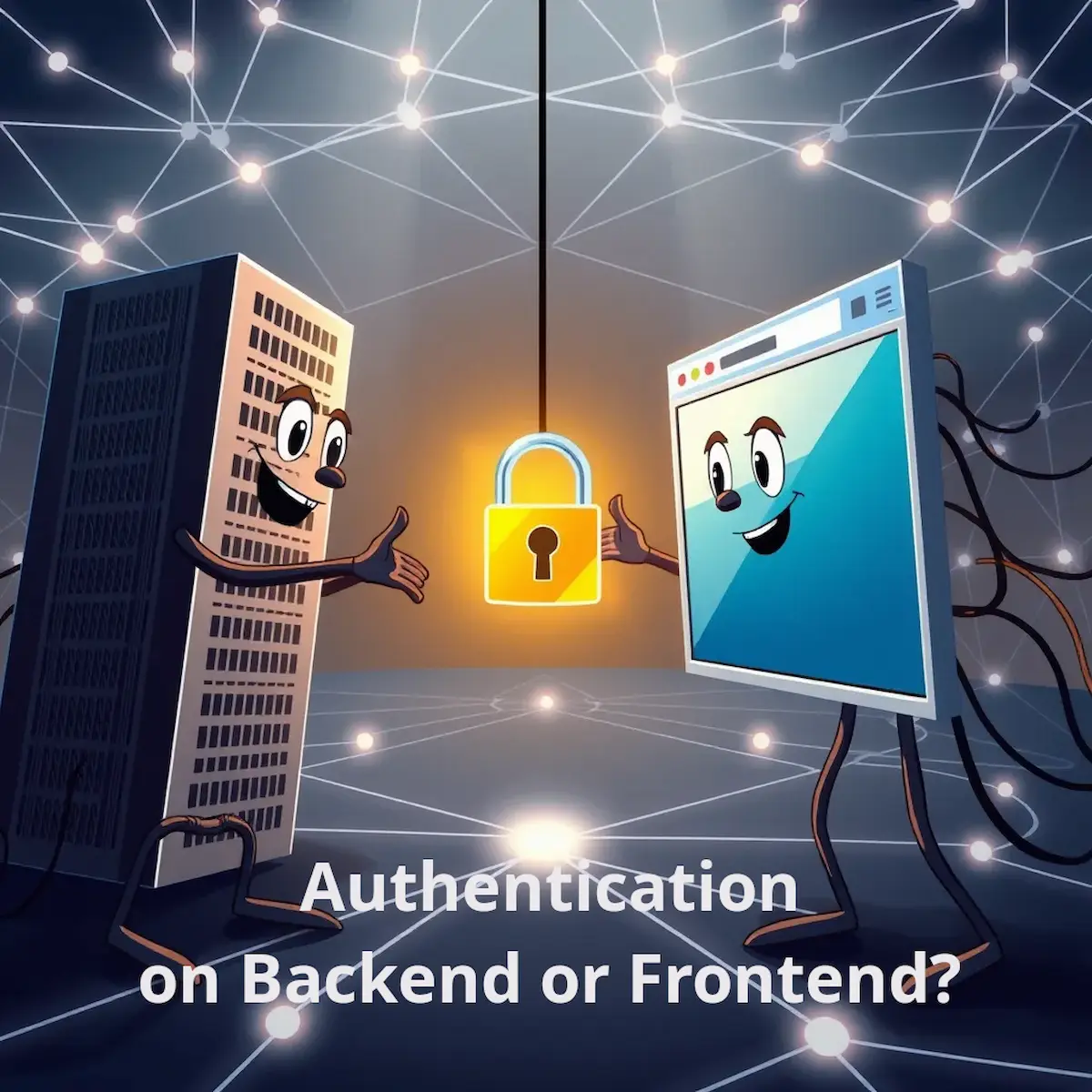
Implementing Authentication with the Lucia Library: Backend vs. Frontend Approaches
- Ctrl Man
- Technology , Security , Authentication
- 09 Oct, 2024
Implementing Authentication with the Lucia Library: Backend vs. Frontend Approaches
Authentication is a crucial aspect of modern web applications, ensuring that users are who they claim to be and protecting sensitive information. The Lucia library offers a flexible solution for implementing authentication in various environments. This article explores two primary approaches to implementing authentication with Lucia: a backend approach using Express.js and a frontend approach using Astro.
What is Lucia?
Lucia is an auth library for TypeScript that provides a simple, flexible, and customizable way to add authentication to your web applications. It supports various databases and adapters, making it versatile for different project requirements.
Lucia’s Abstract Approach to Authentication
The statement that Lucia has a more abstract approach to authentication implementation is indeed valid. Here’s why:
-
Database Agnostic: Lucia is designed to work with various databases through its adapter system. This abstraction allows developers to switch databases without changing the authentication logic.
import { lucia } from "lucia"; import { postgresAdapter } from "@lucia-auth/adapter-postgresql"; const auth = lucia({ adapter: postgresAdapter(pool), // other configurations });
-
Framework Independence:
While many auth libraries are tightly coupled with specific frameworks, Lucia provides a core that can be integrated with different frameworks (Express, Astro, SvelteKit, etc.) using middleware.
-
Customizable Sessions:
Lucia abstracts session management, allowing developers to easily customize session duration, data storage, and invalidation without dealing with low-level details.
const session = await auth.createSession({ userId: user.id, attributes: {} });
-
Flexible Authentication Methods:
Lucia doesn’t prescribe specific authentication methods. It provides a foundation that can be extended to support various auth strategies (password, OAuth, MFA, etc.).
-
Separation of Concerns:
Lucia separates core authentication logic from request/response handling, making it easier to reason about and test authentication flows.
Comparison with other libraries:
- Passport.js: More concrete in its approach, often requiring specific implementations for each strategy.
- Firebase Auth: Provides a higher-level abstraction but is tightly coupled with Firebase ecosystem.
- Auth0: Offers abstraction but as a third-party service, while Lucia keeps control in your application.
Conclusion: Lucia’s abstract approach offers flexibility and adaptability, allowing developers to implement authentication in a way that best fits their specific project needs without being constrained by rigid, framework-specific patterns.
Introduction
When implementing authentication with the Lucia library, developers face a critical decision: should the authentication logic reside on the backend or the frontend? This choice has significant implications for security, performance, and overall application architecture. Let’s explore the pros and cons of each approach to help you make an informed decision for your project.
Backend (Express)
Pros
-
Security
-
Sensitive Operations: Sensitive operations like password hashing and user management are handled on the server.
import { createHash } from 'crypto'; const hashedPassword = createHash('sha256').update('password').digest('hex');
-
Centralized Control: Full control over authentication processes allows for custom logic, role-based access, and centralized session management.
-
Easier Integration: Easier integration with other server-side middleware such as logging, auditing, or security measures.
-
-
Better API Protection
-
Securing Routes: Securing routes based on user authentication can be more straightforward.
import { authenticate } from '@lucia-auth/express'; app.get('/protected', authenticate(), (req) => { // Only authenticated users can access this route });
-
-
Centralized State Management
- Managing sessions and user states is easier in a centralized server environment.
Cons
- Increased Complexity
- More boilerplate code and additional server-side logic can increase complexity.
- Latency
- Each authentication request may introduce latency due to the round trip to the server.
- Scalability Concerns
- Managing more server resources and infrastructure for user sessions as your application scales.
Frontend (Astro)
Pros of Frontend Approach
- Performance
- Reduced latency since authentication checks can be done client-side, leading to faster responses and interactions.
- Simplicity
- Easier implementation in smaller applications or prototypes, allowing for rapid development without server overhead.
- Immediate Feedback
- Immediate feedback and interactions in the user interface improve the overall experience.
- Reduced Server Load
- Offloading authentication logic can reduce the load on your server, allowing it to handle more requests for other functionalities.
Cons of Frontend Approach
- Security Risks
- Exposing authentication logic on the client-side can lead to vulnerabilities such as token manipulation or client-side attacks.
- Limited Control
- Less flexibility in implementing complex authentication flows like multi-factor authentication or custom session management.
- State Management
- Managing authentication states and user sessions can become complicated, especially when handling multiple clients or sessions.
Security Considerations
For Backend (Express)
-
Secure Password Hashing:
import { createHash } from 'crypto'; const hashedPassword = createHash('sha256').update('password').digest('hex');
-
Token Manipulation Protection: Use libraries like
@lucia-auth/express
for secure token management.
For Frontend (Astro)
- Token Manipulation Protection: Implement client-side validation and use HTTPS to protect against man-in-the-middle attacks.
- CSRF Protection:
Utilize libraries like
@lucia/auth
to mitigate CSRF attacks.
Conclusion
Backend (Express): Opt for a backend implementation if security, centralized control, and API protection are your main priorities. This is especially important for larger applications or those requiring stringent security measures.
Frontend (Astro): Consider frontend implementation for simpler applications, prototypes, or when performance and user experience are critical. However, be cautious about the potential security implications.
Choosing the Right Approach
The choice between backend and frontend approaches often depends on the specific needs of your application, including its scale, security requirements, and the complexity of the authentication logic you plan to implement. For instance:
-
Large-Scale Applications: If you are building a large-scale application that requires robust security measures, centralized control over user sessions, and stringent API protection, then a backend implementation using Express is likely the better choice.
-
Small Applications or Prototypes: When working on small applications or prototypes where performance and user experience are critical, but security can be less stringent, a frontend approach with Astro might suffice.
Tips for Implementing Authentication
- Security Best Practices: Always prioritize security by using secure password hashing algorithms, protecting tokens from manipulation, and keeping authentication logic secure.
- Testing: Thoroughly test your implementation under various conditions to ensure reliability and robustness.
- Documentation: Maintain clear and comprehensive documentation to help future developers understand the system.
By considering these factors, you can make an informed decision that aligns with your project’s goals and requirements.
Final Thoughts
In summary, both approaches have their merits and drawbacks. The key is to carefully consider your application’s specific requirements before making the decision. Whether you choose backend or frontend implementation, ensure that you balance security needs with performance and ease of use to provide the best possible user experience while protecting sensitive data and operations.