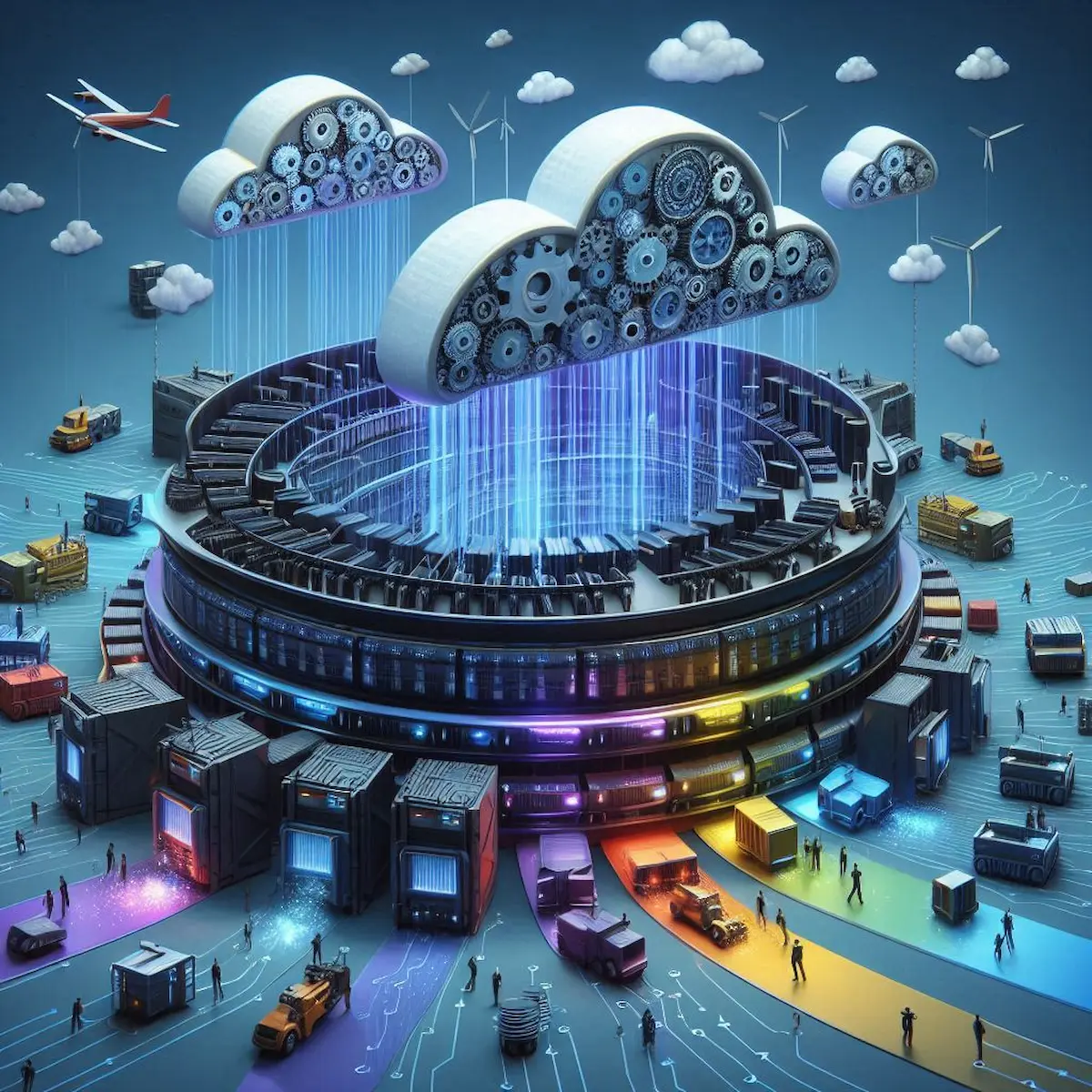
Automatically Converting Weather Codes into Descriptive Text with JavaScript
- Ctrl Man
- JavaScript , APIs , Weather Data
- 11 Jun, 2024
Automatically Converting Weather Codes into Descriptive Text with JavaScript
Introduction
Weather APIs often return forecasts and conditions using numeric codes, which can be challenging for users without extensive knowledge of these codes. This article provides an easy-to-follow guide on how to create a function that translates weather code values into human-readable descriptions.
Understanding Weather Codes
Before diving in, it’s essential to understand the basics of weather codes. These codes typically represent different conditions such as clear skies (0
), partly cloudy (2
), rain (61
), snow (71
), and many more. The mapping between these numeric values and their descriptions can vary depending on the source API. For example, the National Weather Service API has a different set of codes compared to the OpenWeatherMap API.
Sample Mapping
For demonstration purposes, let’s create a JavaScript object that maps a few common weather codes to their descriptions:
const weatherCodeToDescription = {
0: 'Clear sky',
1: 'Mainly clear',
2: 'Partly cloudy',
// ... add more codes and descriptions here,
};
Implementing the Conversion Logic
We’ll create a function that takes in the weather code as an input and returns the corresponding description. If the provided code is not found in our mapping, it will return a default message.
function convertWeatherCode(code) {
const description = weatherCodeToDescription[code] || 'Unknown';
console.log(`The weather for this code (${code}) is ${description}`);
}
Using the Function
Now let’s test the function with some common and uncommon codes:
convertWeatherCode(0); // Output: The weather for this code (0) is Clear sky
convertWeatherCode(2); // Output: The weather for this code (2) is Partly cloudy
convertWeatherCode(54); // Output: The weather for this code (54) is Unknown
Incorporating into an API Response
To integrate the code conversion functionality with API requests, you can make a call to retrieve data in JSON format and then pass it through your convertWeatherCode
function:
const url = 'https://api.weather.gov/forecast';
fetch(url)
.then(response => {
if (!response.ok) throw new Error('Network response was not ok');
return response.json();
})
.then(data => {
const weatherCode = data.properties.periods[0].detailedForecast; // Adjust based on actual API response structure
convertWeatherCode(weatherCode);
})
.catch(error => console.error('Error fetching weather data:', error));
Handling API Responses
When dealing with actual APIs, the structure of JSON responses may vary. Make sure to adapt the code extraction logic according to the specific format returned by your chosen weather API.
Conclusion
Automating the conversion of weather codes into descriptive text can greatly enhance user experience, especially for those who are new to using weather APIs or need a quick and easy way to understand current conditions. By implementing a simple mapping function like the one demonstrated here, you ensure that users receive clear and understandable descriptions without needing in-depth knowledge of code mappings.
Next Steps
- Expand your mapping: Include more common codes as well as those from less frequent weather occurrences.
- Error Handling: Implement better error handling to account for situations where data retrieval fails or when unexpected API formats are encountered.
- Dynamic Updates: Integrate the function into a system that can update descriptions in real-time, reflecting changes in the current weather conditions.
Advanced Considerations
- Internationalization: If your application serves a global audience, consider translating weather descriptions into different languages.
- API Changes: Keep track of updates in weather APIs to ensure your mapping stays accurate and up-to-date.
By following these steps, you’ll be well on your way to creating a useful and user-friendly tool for anyone interested in understanding weather forecasts more easily.