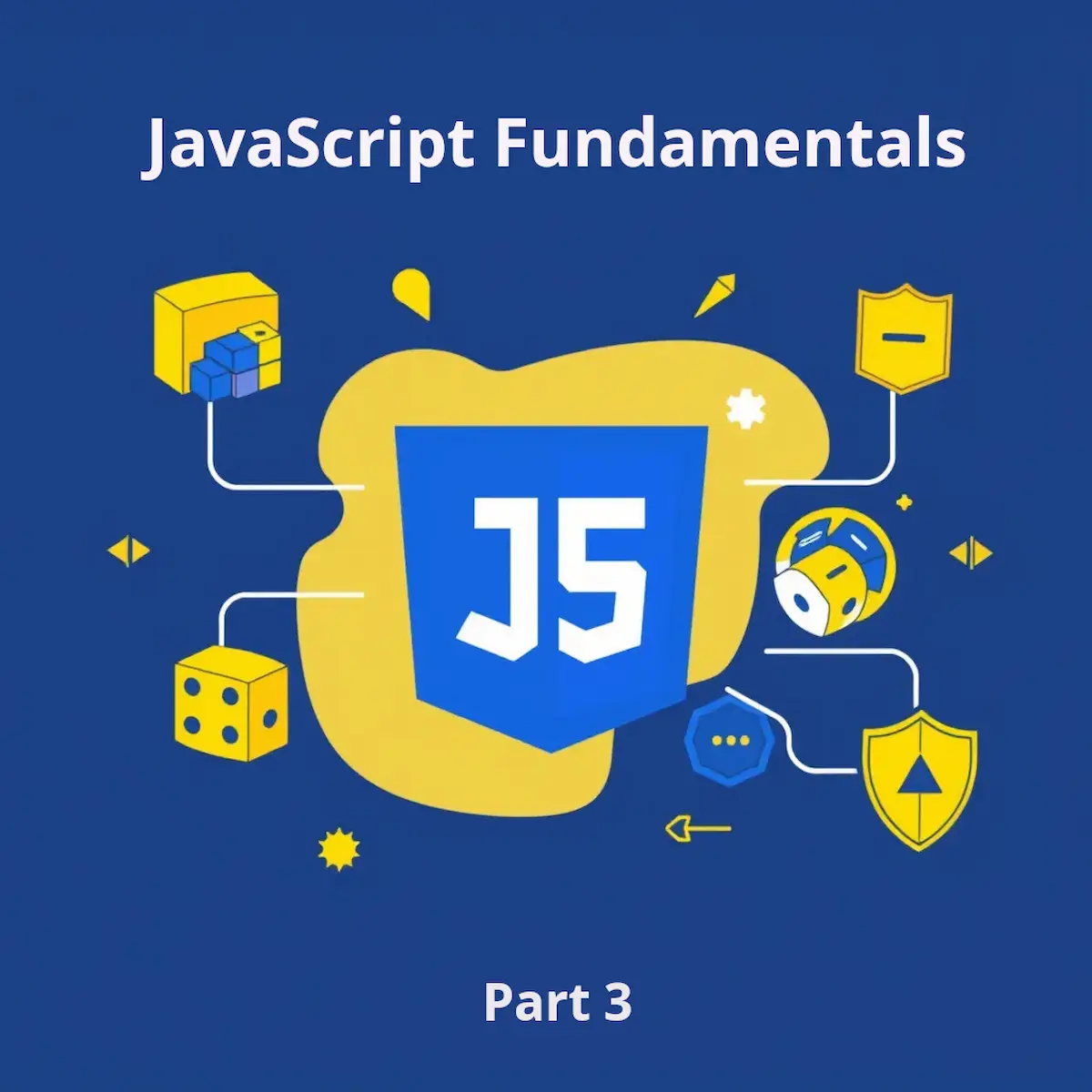
Mastering JavaScript Fundamentals: A Comprehensive Guide - Part 3
- Ctrl Man
- Programming , JavaScript
- 28 May, 2024
Mastering JavaScript: A Journey Through Code Debugging
Introduction
JavaScript is a powerful and essential language for web development. While it enables developers to create dynamic and interactive web applications, mastering it requires a deep understanding of its nuances and the ability to troubleshoot effectively. In this article, I’ll take you through a real-world example of debugging a JavaScript application to highlight key concepts and best practices.
The Problem
I recently faced a challenge while working on a drum kit application. The goal was simple: play different drum sounds when buttons are clicked or corresponding keys are pressed. However, the initial code had issues that resulted in errors and unexpected behavior.
Initial Code and Errors
Here’s the initial version of the code:
var NumberOfDB = document.querySelectorAll(".drum").length;
var audio = document.getElementById("myAudio");
for (var i = 0; i < NumberOfDB; i++) {
document.querySelectorAll(".drum")[i].addEventListener("click", function () {
makeSound(this.innerHTML);
});
}
document.addEventListener("keypress", function(event) {
makeSound(event.key);
alert("pressed");
});
function makeSound(key) {
var sound = new Audio();
switch (key) {
case "w":
sound.src = "sounds/tom-1.mp3";
break;
case "a":
sound.src = "sounds/tom-2.mp3";
break;
case "s":
sound.src = "sounds/tom-3.mp3";
break;
case "d":
sound.src = "sounds/tom-4.mp3";
break;
case "j":
sound.src = "sounds/snare.mp3";
break;
case "k":
sound.src = "sounds/crash.mp3";
break;
case "l":
sound.src = "sounds/kick-bass.mp3";
break;
}
sound.play();
}
Understanding Event Listeners
Before diving into the errors, let’s take a moment to understand event listeners. An event listener is a function that is triggered in response to a specific event, such as a click or key press. In the code above, we’re using addEventListener
to attach event listeners to the drum buttons and the document. The event listener function is called whenever the specified event occurs.
The Errors
The issues included syntax errors, doubled alerts, and performance warnings like ‘[Violation] ‘keypress’ handler took 1386ms’. These errors were reported by the TypeScript compiler and the browser console.
Debugging and Fixing the Errors
-
Syntax Errors: Missing commas and an extra closing parenthesis were causing the code to break. After fixing these syntax errors, the code still had functional issues.
-
Doubled Alerts: The alert was being triggered twice because both the click and keypress events were firing the
makeSound
function. -
Performance Issues: The ‘keypress’ event handler was taking too long, leading to delays and potential duplicated events.
Optimized Solution
To address these issues, I optimized the code by preloading audio files, using flags to track keydown states, and replacing the keypress
event with keydown
for better performance.
Here is the optimized version:
var audioMap = {
"w": "sounds/tom-1.mp3",
"a": "sounds/tom-2.mp3",
"s": "sounds/tom-3.mp3",
"d": "sounds/tom-4.mp3",
"j": "sounds/snare.mp3",
"k": "sounds/crash.mp3",
"l": "sounds/kick-bass.mp3"
};
var audioCache = {}; // Cache for preloaded audio elements
document.querySelectorAll(".drum").forEach(function(drum) {
drum.addEventListener("click", function() {
makeSound(this.innerHTML);
});
});
var keyDownFlag = {}; // Flag to track if a key is being held down
document.addEventListener("keydown", function(event) {
if (event.target.tagName.toLowerCase() !== "input" && !keyDownFlag[event.key]) {
keyDownFlag[event.key] = true;
makeSound(event.key);
console.log("Key pressed:", event.key);
}
});
document.addEventListener("keyup", function(event) {
keyDownFlag[event.key] = false;
});
function makeSound(key) {
var sound = audioCache[key];
if (!sound) {
sound = new Audio(audioMap[key]);
audioCache[key] = sound;
}
sound.currentTime = 0; // Reset audio time to play from the beginning
sound.play();
}
Conclusion
Debugging JavaScript can be a challenging but rewarding process. By methodically identifying and resolving errors, you can significantly improve the performance and reliability of your code. This experience has reinforced the importance of careful debugging and optimization in JavaScript development. Happy coding!
Support
If you found this article helpful, share your debugging experiences or any tips you have in the comments below. For more articles on JavaScript and web development, follow my blog!