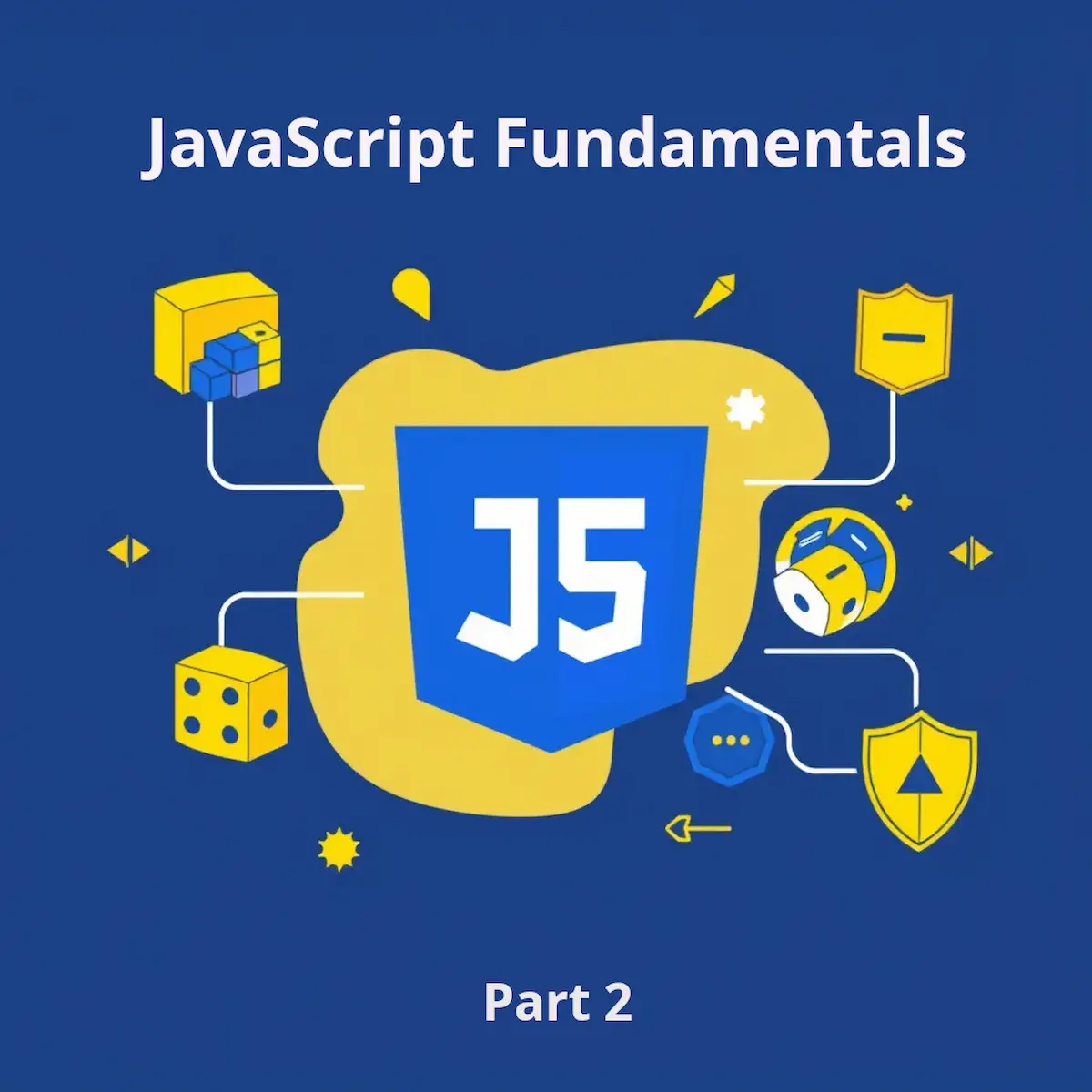
Mastering JavaScript Fundamentals: A Comprehensive Guide - Part 2
- Ctrl Man
- Programming , JavaScript
- 28 May, 2024
Introduction
In this guide, we will cover essential concepts in JavaScript, including operators, array manipulation, random number generation, and error handling. Understanding these concepts is crucial for beginner web developers to build a strong foundation in JavaScript.
JavaScript Operators
JavaScript operators are used to perform operations on variables and values. There are different types of operators, including:
- Arithmetic operators (e.g.,
+
,-
,*
,/
) - Comparison operators (e.g.,
==
,!=
,===
,!==
) - Logical operators (e.g.,
&&
,||
,!
) - Assignment operators (e.g.,
=
,+=
,-=
,*=
)
Array Manipulation
Arrays are a fundamental data structure in JavaScript. Here are some essential methods for manipulating arrays:
push()
: adds one or more elements to the end of an arraypop()
: removes the last element from an arrayshift()
: removes the first element from an arrayunshift()
: adds one or more elements to the beginning of an arraysplice()
: adds or removes elements from a specific position in an array
Random Number Generation
Generating random numbers is a common task in JavaScript. You can use the Math.random()
function to generate a random number between 0 and 1. To generate a random number within a specific range, you can use the following formula:
Math.floor(Math.random() * (max - min + 1)) + min
Error Handling
Error handling is crucial in JavaScript to prevent errors from crashing your application. You can use try-catch
blocks to catch and handle errors:
try {
// code that might throw an error
} catch (error) {
// handle the error
}
FAQ Section
-
What is the difference between
==
and===
in JavaScript? –==
compares two values for equality after converting both variables to a common type.===
, known as the strict equality operator, compares both value and type without converting. -
How can I remove a specific element from an array? – You can use the
splice()
method, which allows you to remove elements from any position in an array. For example,array.splice(index, 1)
will remove the element at the specified index. -
What is a closure in JavaScript? – A closure is a function that remembers the variables from the place where it is defined, regardless of where it is executed later.
-
How do I prevent a form from submitting automatically with JavaScript? – You can prevent a form from submitting by calling the
preventDefault()
method on the form’s submit event:form.onsubmit = function(event) { event.preventDefault(); };
-
Can you explain the
this
keyword in JavaScript? – Thethis
keyword refers to the object that is executing the current piece of code. Its value can change depending on the context in which it is used, such as in different types of functions or event handlers. -
What are JavaScript Promises and how do they work? – Promises are objects that represent the eventual completion (or failure) of an asynchronous operation. They provide a way to handle asynchronous operations by offering success and error callbacks.
-
How do I copy an array without modifying the original in JavaScript? – You can create a shallow copy of an array using the spread syntax:
let newArray = [...originalArray];
-
What is event bubbling and event capturing in JavaScript? – Event bubbling and capturing are two ways of event propagation in the HTML DOM API. In bubbling, the event starts from the target element and bubbles up to the ancestors, while in capturing, it starts from the top and goes down to the target element.
-
How can I convert a string to a number in JavaScript? – You can convert a string to a number using the
parseInt()
orparseFloat()
functions, or by prefixing the string with a+
sign. -
What is the use of the
map()
function in JavaScript? – Themap()
function creates a new array by calling a provided function on every element in the calling array.
Conclusion
In this guide, we covered essential concepts in JavaScript, including operators, array manipulation, random number generation, and error handling. By mastering these concepts, you’ll be well on your way to becoming a proficient JavaScript developer. Remember to practice coding exercises and use online resources to continue learning and improving your skills.