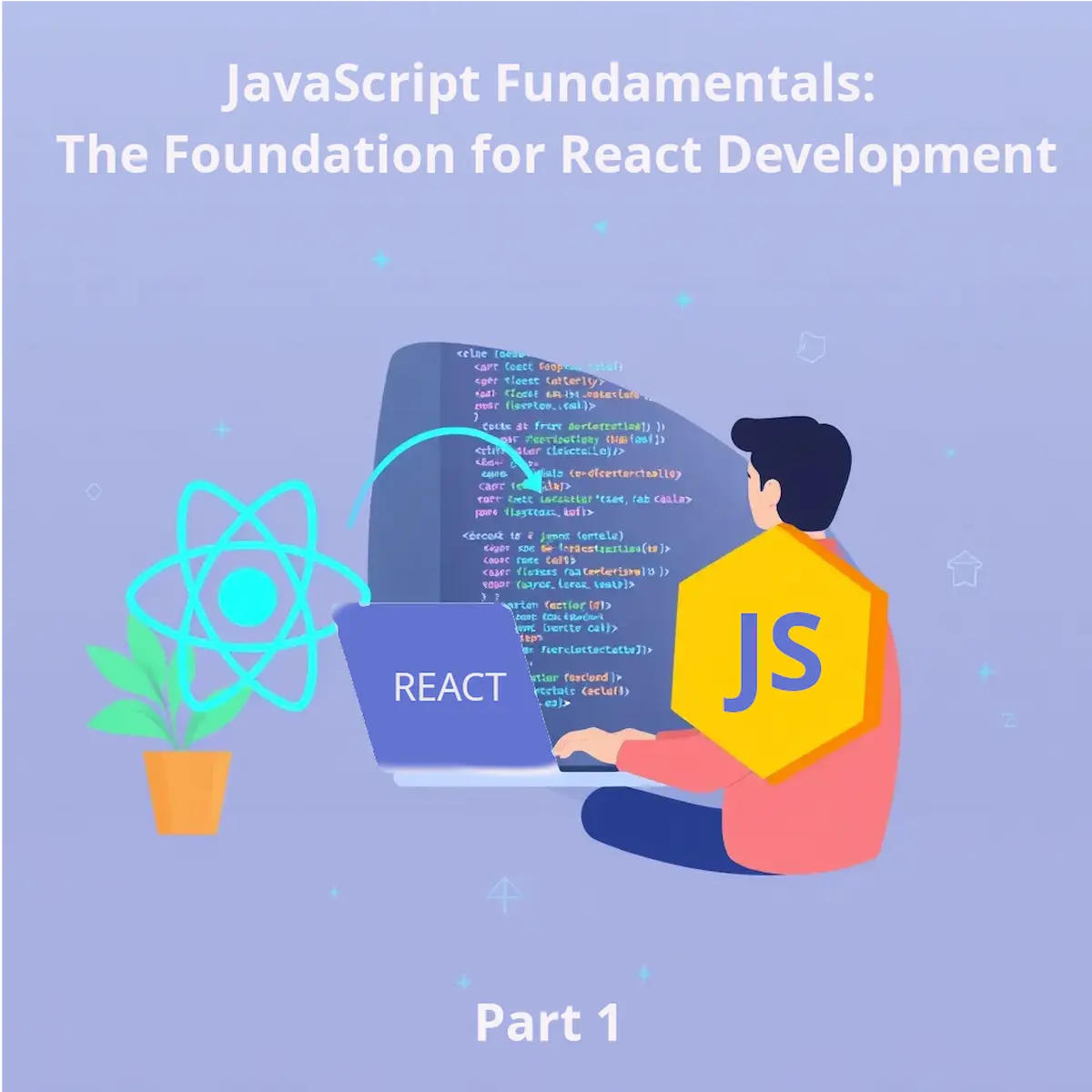
JavaScript Fundamentals: The Foundation for React Development
- Ctrl Man
- Web Development , Programming Languages
- 18 Jul, 2024
JavaScript Fundamentals: The Foundation for React Development
Introduction: Why Learn JavaScript Before React?
As you embark on your journey to learning web development, it’s crucial to understand the foundational role that JavaScript plays in creating dynamic user experiences online. JavaScript serves as the foundation for React, a popular library for building user interfaces. While React enhances and simplifies many aspects of web development, it’s built upon core JavaScript principles. Understanding JavaScript deeply allows you to leverage React’s full potential and troubleshoot issues more effectively. This article will guide you through the essential JavaScript concepts before introducing React, helping you build a solid foundation for your web development journey.
JavaScript Fundamentals
Variables, Data Types, and Functions
JavaScript allows us to store values using variables:
let age = 25;
Here age
is a variable storing an integer value. There are several data types supported by JavaScript, including:
-
Numbers:
let num = 10;
-
Strings:
let greeting = "Hello, world!";
-
Booleans:
let isOnline = true;
Functions in JavaScript enable us to write reusable code blocks:
function greet(name) {
console.log(`Hi ${name}, welcome to my site!`);
}
Arrays and Objects
Arrays and objects are crucial data structures in JavaScript, extensively used in React development.
Arrays are ordered lists of values:
let fruits = ['apple', 'banana', 'orange'];
console.log(fruits[1]); // Output: 'banana'
// Adding an item to the end of the array
fruits.push('grape');
Objects are collections of key-value pairs:
let person = {
name: 'Alice',
age: 30,
isStudent: false
};
console.log(person.name); // Output: 'Alice'
// Adding a new property
person.occupation = 'Engineer';
Asynchronous JavaScript
Asynchronous operations are common in web development, especially when dealing with APIs. Here’s a simple example using fetch
:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
This code fetches data from an API asynchronously, allowing other code to run while waiting for the response.
DOM Manipulation with Vanilla JavaScript
The Document Object Model (DOM) provides an interface for scripts to interact with HTML documents. We can change elements on the page using vanilla JavaScript without relying on a framework:
document.getElementById('myButton').addEventListener('click', function() {
console.log('Button was clicked!');
});
Event Handling in JavaScript
JavaScript’s ability to handle various events makes it indispensable for creating interactive web applications:
Click Events Example:
const button = document.querySelector('#some-button');
button.addEventListener('click', () => {
alert('Button clicked!');
});
Conclusion
Understanding these JavaScript fundamentals provides a solid foundation for learning React. In our next article, we’ll explore how React builds upon these concepts to create powerful user interfaces.