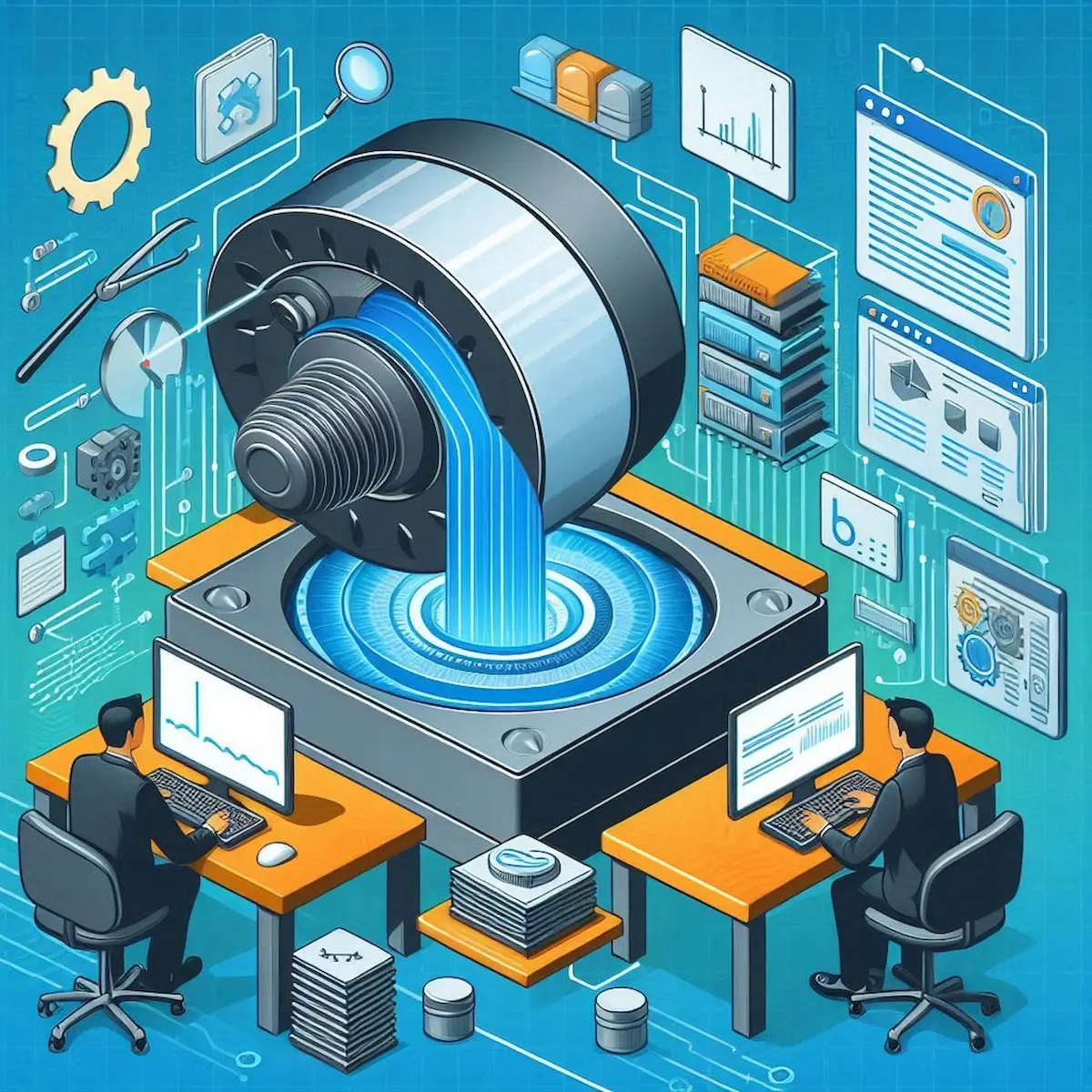
Mastering Asynchronous Data Streams: A Beginner's Guide to Accumulating Data Chunks
- Ctrl Man
- Programming , JavaScript , Node.js
- 11 Jun, 2024
Mastering Asynchronous Data Streams: A Beginner’s Guide to Accumulating Data Chunks
Introduction
Navigating the world of asynchronous programming can often feel daunting, especially when dealing with large amounts of data that come in pieces or chunks. Whether you’re working with HTTP responses, file I/O operations, or any other scenario involving streaming data, understanding how to effectively accumulate these chunks is crucial for a smooth and efficient development experience. In this article, we’ll explore the process behind accumulating data chunks using common patterns, such as the data += chunk;
technique.
Understanding Chunk Accumulation
When you encounter large datasets that arrive piece by piece rather than all at once, it’s important to have a mechanism in place to handle these chunks without losing any information. In the case of an HTTP response received over an asynchronous connection using Node.js’ https.get
method, data is delivered in chunks due to limitations on how much can be sent at once.
The line:
data += chunk;
plays a critical role here. It demonstrates the process of accumulating incoming chunks into a single variable or string (data
in our example). This ensures that all parts of the response are collected before they’re needed for further processing, such as parsing JSON content.
Step-by-Step Breakdown
- Initialization: At the beginning, you initialize your accumulation variable (e.g.,
let data = '';
) to store all received chunks. - Accumulation: As each chunk of data arrives through an event like
'data'
, it is concatenated (+=
) onto the current value stored in thedata
variable. - Completion Handling: Once all expected chunks have been processed (indicated by the
'end'
event), you now possess a complete string containing all received information, ready for further processing.
Asynchronous Nature of Data Accumulation
Accumulating data in this manner is inherently asynchronous due to the nature of handling streaming data. This approach ensures your application remains responsive and efficient:
- Non-blocking Operations: Your code continues running even while waiting for chunks to arrive, allowing it to execute other tasks without delay.
- Event-driven Processing: Each chunk triggers a callback that appends the data to
data
, keeping track of all received information until the'end'
event confirms everything is in place.
Example 1: HTTP Response Accumulation in Node.js
Let’s look at a practical example using Node.js to accumulate data chunks from an HTTP response:
const https = require('https');
https.get('https://jsonplaceholder.typicode.com/posts', (resp) => {
let data = '';
// A chunk of data has been received.
resp.on('data', (chunk) => {
data += chunk;
});
// The whole response has been received. Print out the result.
resp.on('end', () => {
console.log(JSON.parse(data));
});
}).on("error", (err) => {
console.log("Error: " + err.message);
});
In this example, each chunk of data from the HTTP response is accumulated into the data
variable until the entire response is received and processed.
Example 2: File Reading in Node.js
Another common scenario involves reading data from a file in chunks:
const fs = require('fs');
const readStream = fs.createReadStream('largefile.txt');
let data = '';
readStream.on('data', (chunk) => {
data += chunk;
});
readStream.on('end', () => {
console.log('File read complete:', data);
});
readStream.on('error', (err) => {
console.log('Error:', err);
});
Here, the readStream
object reads chunks of data from a large file and accumulates them into the data
variable.
Conclusion
Mastering the process of accumulating data chunks is essential for efficient asynchronous programming, particularly when dealing with large datasets or streaming data. By initializing an accumulation variable, concatenating chunks as they arrive, and handling the completion of data transfer, you can ensure that all information is properly gathered and ready for further processing. The examples provided demonstrate practical applications in both HTTP responses and file I/O operations, showcasing the versatility and importance of this technique in various programming scenarios.
Understanding and implementing these concepts will help you create more responsive and efficient applications, making the daunting task of handling asynchronous data streams more manageable and straightforward.