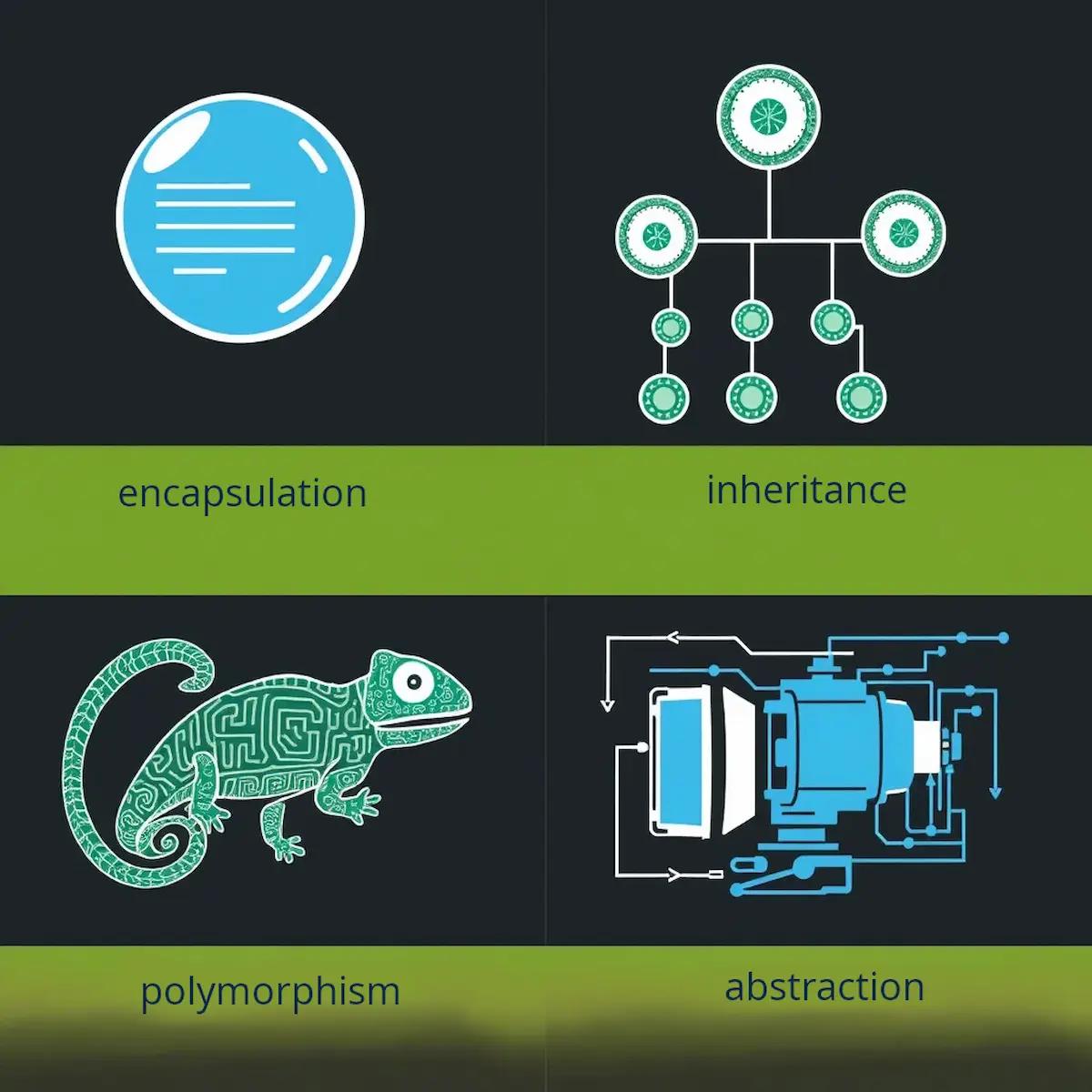
Understanding OOP Concepts: A Guide for Junior Web Developers
- Ctrl Man
- Web Development , Programming Fundamentals
- 26 Sep, 2024
Understanding OOP Concepts: A Guide for Junior Web Developers
As a junior web developer, one of the most crucial skills you need to develop is a strong understanding of Object-Oriented Programming (OOP). While these concepts are foundational in many programming languages like Java and Python, they also apply directly to JavaScript. Mastering these principles will not only make your code more robust but also help you excel during job interviews.
1. Encapsulation
What is Encapsulation? Encapsulation involves bundling data (attributes) and methods (functions) within a single unit or class, while restricting direct access to some of the object’s components through controlled access methods. This ensures that the internal representation of an object remains hidden from outside interference.
Example in JavaScript
class Car {
constructor(model, year) {
this.model = model;
this.__year = year; // Private attribute
}
getYear() {
return this.__year;
}
setYear(year) {
if (year > 2000) {
this.__year = year;
} else {
console.log("Invalid year");
}
}
}
const myCar = new Car('Toyota', 2010);
console.log(myCar.getYear()); // Output: 2010
myCar.setYear(2023); // Sets the year to 2023
console.log(myCar.getYear()); // Output: 2023
Potential Interview Questions
- What is encapsulation, and why is it important?
- How can you achieve encapsulation in JavaScript?
- Can you bypass encapsulation? If so, how?
2. Inheritance
What is Inheritance? Inheritance allows a class (child or derived class) to inherit attributes and methods from another class (parent or base class), promoting code reuse and creating hierarchical relationships.
Example in JavaScript
class Vehicle {
constructor(make, model) {
this.make = make;
this.model = model;
}
displayInfo() {
console.log(`Make: ${this.make}, Model: ${this.model}`);
}
}
class Car extends Vehicle {
constructor(make, model, doors) {
super(make, model);
this.doors = doors;
}
displayCarInfo() {
this.displayInfo();
console.log(`Doors: ${this.doors}`);
}
}
const myCar = new Car('Toyota', 'Corolla', 4);
myCar.displayCarInfo(); // Output:
// Make: Toyota, Model: Corolla
// Doors: 4
Potential Interview Questions
- What is inheritance, and how does it work?
- What is the difference between single and multiple inheritance in JavaScript (if applicable)?
- How does
super()
function work in JavaScript?
3. Polymorphism
What is Polymorphism? Polymorphism allows methods to behave differently based on the object they are acting upon, typically achieved through method overriding or operator overloading.
Example in JavaScript (Method Overriding)
class Animal {
speak() {
console.log("The animal makes a sound");
}
}
class Dog extends Animal {
speak() {
console.log("The dog barks");
}
}
class Cat extends Animal {
speak() {
console.log("The cat meows");
}
}
function makeAnimalSpeak(animal) {
animal.speak();
}
const myDog = new Dog();
const myCat = new Cat();
makeAnimalSpeak(myDog); // Output: The dog barks
makeAnimalSpeak(myCat); // Output: The cat meows
Potential Interview Questions
- What is polymorphism, and how does it relate to method overriding?
- How does JavaScript implement polymorphism?
- What is the difference between compile-time and run-time polymorphism in JavaScript (if applicable)?
4. Data Abstraction
What is Data Abstraction? Data abstraction is the concept of providing only essential information to the outside world while hiding implementation details, typically achieved through abstract classes or interfaces.
Example in JavaScript
class Shape {
area() {
// Method body should be implemented by derived classes
}
}
class Rectangle extends Shape {
constructor(width, height) {
super();
this.width = width;
this.height = height;
}
area() {
return this.width * this.height;
}
}
const myRectangle = new Rectangle(5, 10);
console.log(myRectangle.area()); // Output: 50
Potential Interview Questions
- What is data abstraction?
- How can you achieve abstraction in JavaScript?
- What is the difference between abstraction and encapsulation?
5. Interface
What is an Interface? An interface defines a blueprint of a class with abstract methods that must be implemented by derived classes.
Example in JavaScript
class Payment {
processPayment(amount) {
// Method body should be implemented by derived classes
}
}
class PayPalPayment extends Payment {
processPayment(amount) {
console.log(`Processing PayPal payment of ${amount}`);
}
}
class CreditCardPayment extends Payment {
processPayment(amount) {
console.log(`Processing credit card payment of ${amount}`);
}
}
const myPayPal = new PayPalPayment();
myPayPal.processPayment(100); // Output: Processing PayPal payment of 100
Potential Interview Questions
- What is an interface, and how is it different from a class?
- What’s the role of abstract methods in an interface?
- How does JavaScript simulate interfaces?
6. Abstract Base Classes
What are Abstract Base Classes? Abstract base classes (ABCs) provide a way to define abstract methods that must be implemented by derived classes, but cannot be instantiated directly.
Example in JavaScript
class Animal {
sound() {
// Method body should be implemented by derived classes
}
}
class Dog extends Animal {
sound() {
return "Bark";
}
}
class Cat extends Animal {
sound() {
return "Meow";
}
}
const myDog = new Dog();
console.log(myDog.sound()); // Output: Bark
Potential Questions
- What is an abstract class, and how is it different from a concrete class?
- Can you instantiate an abstract class in JavaScript?
- Why would you use an abstract class instead of a concrete class?
Key Differences Between Concepts
-
Encapsulation vs. Abstraction:
- Encapsulation: Hides data within a class to protect it from the outside world.
- Abstraction: Hides complexity by showing only essential details.
-
Inheritance vs. Polymorphism:
- Inheritance: Creates relationships between parent and child classes.
- Polymorphism: Allows objects to be treated as instances of their parent class but with different behaviors.
-
Abstract Classes vs. Interfaces:
- Abstract Classes: Can have concrete methods and attributes.
- Interfaces: In languages like JavaScript, only declare methods that must be implemented by the class.
Summary of Potential Interview Questions
- Can you explain the concepts of OOP with real-life examples?
- What’s the difference between an abstract class and an interface in JavaScript?
- How does polymorphism enhance the flexibility of your code?
- How does encapsulation improve the security of a class?
Understanding these fundamental OOP concepts is crucial for writing maintainable, scalable, and efficient code. By mastering them, you will not only become a better developer but also excel during job interviews. Keep practicing and stay motivated!