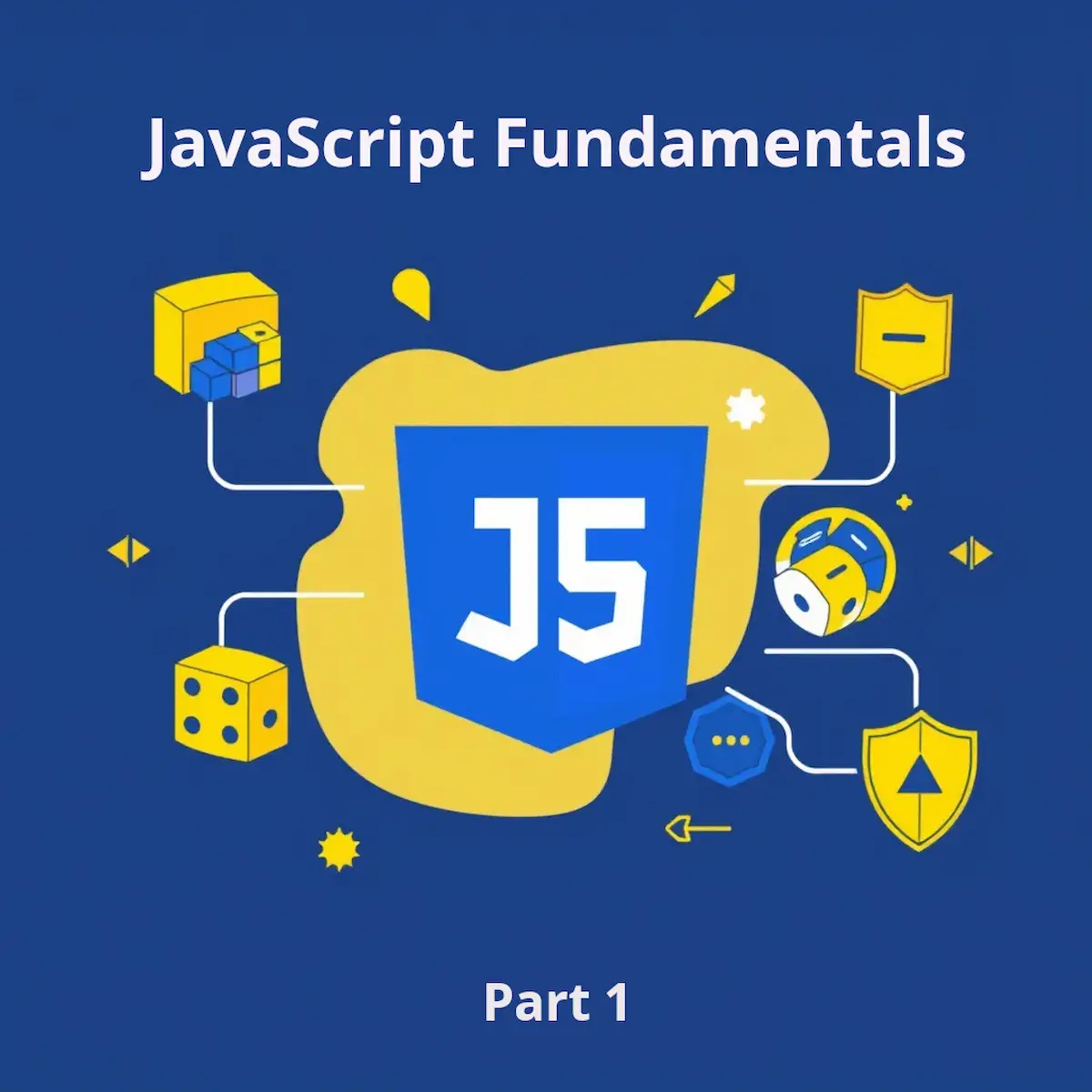
Mastering JavaScript Fundamentals: A Comprehensive Guide – Part 1
- Ctrl Man
- Programming , JavaScript
- 28 May, 2024
Introduction
JavaScript is a versatile programming language that plays a crucial role in web development. Whether you’re building dynamic websites or developing modern web applications, understanding the fundamentals of JavaScript is essential. In this comprehensive guide, we’ll explore some of the core concepts of JavaScript, including operators, array manipulation, random number generation, error handling, and more.
JavaScript Operators
JavaScript provides a variety of operators that allow you to perform different operations on values. One commonly used operator is the negation operator (!
), which inverts the boolean value of an expression. For example:
let x = true;
console.log(!x); // Output: false
Array Manipulation
Arrays are a fundamental data structure in JavaScript, and manipulating them is a common task. To add an element to an array, you can use the push()
method, which appends the element to the end of the array:
let myArray = [1, 2, 3];
myArray.push(4);
console.log(myArray); // Output: [1, 2, 3, 4]
Random Number Generation
Generating random numbers is a common requirement in various applications, such as games, simulations, and data analysis. In JavaScript, you can use the Math.random()
function to generate a random decimal number between 0 (inclusive) and 1 (exclusive):
let randomNumber = Math.random();
console.log(randomNumber); // Output: A random decimal number between 0 and 1
Error Handling
Errors are inevitable in programming, and it’s essential to handle them gracefully to ensure your application’s stability and reliability. JavaScript provides the try...catch
statement for handling exceptions and errors. The try
block contains the code that might throw an error, and the catch
block is executed if an error occurs:
try {
// Code that might throw an error
} catch (error) {
console.error(error);
}
How to automatically increment a variable in JavaScript?
You can use a loop or a counter variable to increment a value automatically:
let counter = 0;
// Using setInterval to increment every 1 second
setInterval(() => {
counter++;
console.log(counter);
}, 1000);
How to add an element to an array in JavaScript?
You can use the push()
method to add an element to the end of an array:
let myArray = [1, 2, 3];
myArray.push(4);
console.log(myArray); // Output: [1, 2, 3, 4]
How to generate a random number in JavaScript?
You can use the Math.random()
function to generate a random decimal number between 0 (inclusive) and 1 (exclusive):
let randomNumber = Math.random();
console.log(randomNumber);
How to handle errors in JavaScript?
You can use the try...catch
statement to handle errors in JavaScript:
try {
// Code that might throw an error
} catch (error) {
console.error(error);
}
Additional Tips
- Use descriptive variable names and comments to make your code more readable and maintainable.
- Test your code thoroughly to ensure it works as expected and handles different scenarios and edge cases.
- Utilize online resources, such as MDN Web Docs, to learn more about JavaScript concepts and best practices.
- Stay updated with the latest JavaScript features and updates by regularly checking the official ECMAScript specification.
FAQ Section
What is the difference between Math.round()
and Math.floor()
?
The Math.round()
function rounds a number to the nearest integer, while Math.floor()
rounds a number down to the nearest integer.
How do I add an element to an array in JavaScript?
You can use the push()
method to add an element to the end of an array, or the unshift()
method to add an element to the beginning of an array.
How do I generate a random number in JavaScript?
You can use the Math.random()
function to generate a random decimal number between 0 (inclusive) and 1 (exclusive). If you need a random integer within a specific range, you can use additional operations like multiplication and rounding.
How do I handle errors in JavaScript?
You can use the try...catch
statement to handle errors in JavaScript. The code that might throw an error is placed inside the try
block, and the catch
block is executed if an error occurs, allowing you to handle the error gracefully.
Conclusion
By following these tips and best practices, you’ll be well on your way to mastering JavaScript fundamentals and building robust and efficient web applications.