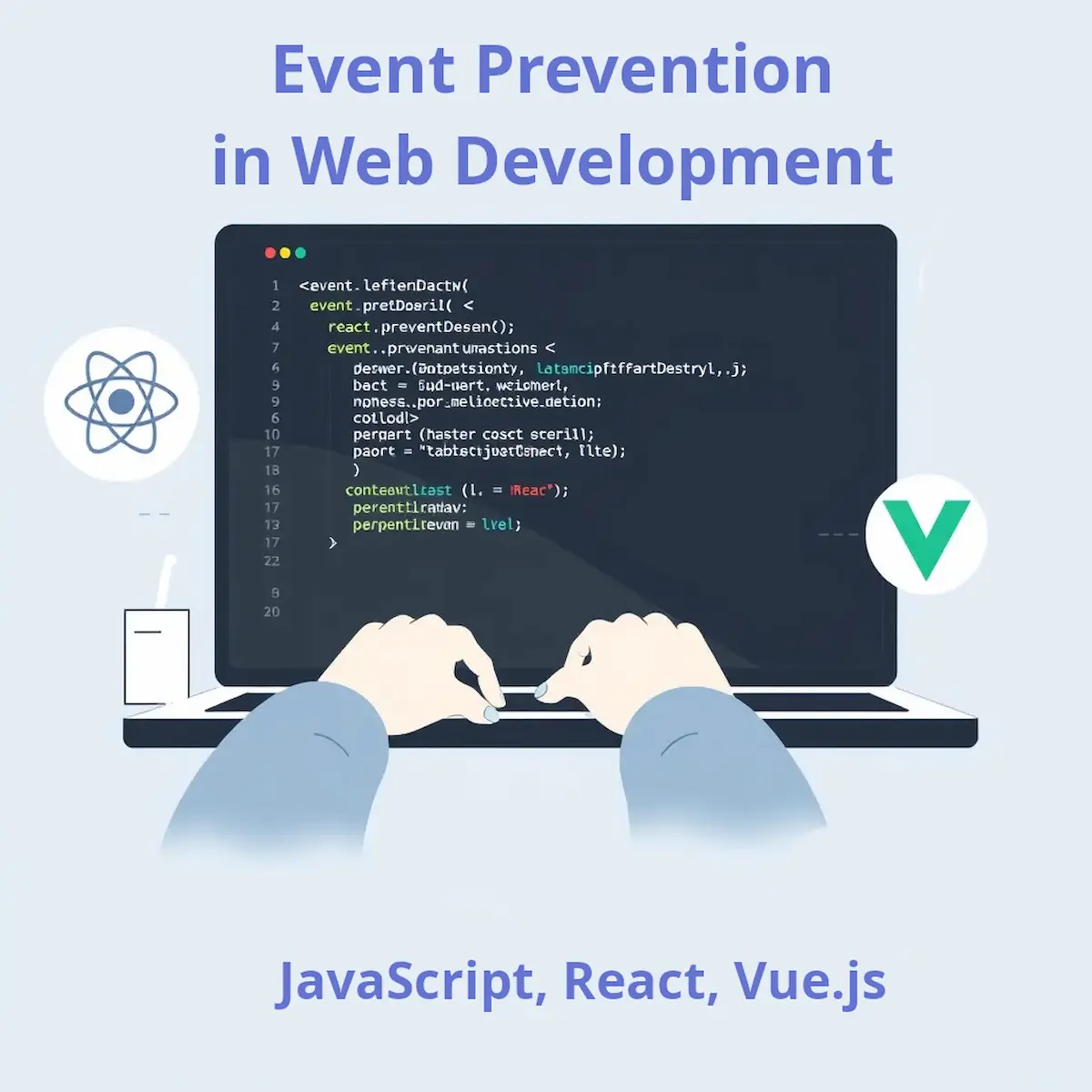
Event Prevention in Web Development: A Comprehensive Guide
- Ctrl Man
- Web Development , JavaScript
- 16 Jul, 2024
Event Prevention in Web Development: A Comprehensive Guide
Introduction
Event prevention is a crucial concept in web development that allows developers to control and customize user interactions. This guide will explore the importance of event prevention, its implementation in various contexts, and best practices for its use.
Understanding Event Prevention
In web development, events are actions triggered by user interactions, such as clicking a button or submitting a form. Each event has a default behavior built into the browser. Event prevention allows developers to override these default behaviors, enabling custom functionality and enhanced user experiences.
The Role of event.preventDefault()
event.preventDefault()
is a method in JavaScript that stops the default action associated with an event. This powerful tool is essential for creating interactive and responsive web applications.
Key Use Cases for Event Prevention
1. Form Handling
Purpose: To control form submission and perform client-side validation.
Example:
const form = document.querySelector('form');
form.addEventListener('submit', (event) => {
event.preventDefault();
// Custom form validation logic
if (validateForm()) {
// Proceed with form submission
form.submit();
}
});
2. Navigation Control
Purpose: To manage page navigation and implement single-page application (SPA) behavior.
Example:
document.querySelectorAll('a').forEach(link => {
link.addEventListener('click', (event) => {
event.preventDefault();
// Custom navigation logic
navigateToPage(link.href);
});
});
3. Custom UI Interactions
Purpose: To create unique user interface elements and interactions.
Example:
const draggable = document.querySelector('.draggable');
draggable.addEventListener('mousedown', (event) => {
event.preventDefault();
// Custom drag-and-drop logic
initiateDrag(event);
});
Implementing Event Prevention in Different Contexts
Vanilla JavaScript
document.querySelector('form').addEventListener('submit', (event) => {
event.preventDefault();
// Custom form handling logic
});
React
function FormComponent() {
const handleSubmit = (event) => {
event.preventDefault();
// Custom form handling logic
};
return (
<form onSubmit={handleSubmit}>
{/* Form fields */}
</form>
);
}
Vue.js
<template>
<form @submit.prevent="handleSubmit">
</form>
</template>
<script>
export default {
methods: {
handleSubmit() {
// Custom form handling logic
}
}
}
</script>
Best Practices for Event Prevention
-
Use Sparingly: Only prevent default behavior when necessary. Overuse can lead to unexpected user experiences.
-
Provide Clear Feedback: When preventing default actions, ensure users understand what’s happening through visual cues or notifications.
-
Consider Accessibility: Ensure that custom event handling doesn’t interfere with accessibility features or keyboard navigation.
-
Performance Optimization: Be mindful of the computational cost of your custom event handlers, especially for frequently triggered events.
-
Browser Compatibility: Test your event prevention code across different browsers to ensure consistent behavior.
Drawbacks of Event Prevention
While event prevention offers significant control over user interactions, it’s essential to be aware of potential drawbacks:
- Reduced Browser Functionality: Overuse of
event.preventDefault()
can hinder users who rely on default browser behaviors, such as right-clicking or using keyboard shortcuts. - Increased Development Complexity: Custom event handling often requires additional code and testing, potentially increasing development time and complexity.
- Potential for User Confusion: If custom interactions are not intuitive or well-designed, users might become confused or frustrated.
- Accessibility Challenges: As mentioned earlier, improper implementation can create barriers for users with disabilities.
Accessibility Considerations
Ensuring that custom event handling doesn’t hinder accessibility is paramount. Here are some key points to consider:
- Keyboard Navigation: Ensure that all interactive elements can be accessed and operated using the keyboard. Provide clear focus indicators for keyboard users.
- Screen Reader Compatibility: Test how custom interactions are handled by screen readers. Provide appropriate ARIA attributes and semantic HTML to convey information to screen reader users.
- Alternative Text: If images or other non-text content are involved in custom interactions, provide alternative text to describe their purpose and functionality.
- Focus Management: When preventing default actions, ensure that focus is managed correctly. For instance, if a link’s default navigation behavior is prevented, provide a clear alternative way for users to navigate to the linked content.
- User Testing: Involve users with disabilities in the testing process to identify and address potential accessibility issues.
Advanced Techniques
Event Delegation
Use event delegation to handle events efficiently for multiple elements:
document.querySelector('ul').addEventListener('click', (event) => {
if (event.target.tagName === 'LI') {
event.preventDefault();
// Handle list item click
}
});
Passive Event Listeners
For scroll performance optimization:
document.addEventListener('scroll', (event) => {
// Scroll handling logic
}, { passive: true });
Conclusion
Event prevention is a powerful technique in web development that allows for greater control over user interactions and browser behavior. By understanding when and how to use event.preventDefault()
, developers can create more dynamic, responsive, and user-friendly web applications. However, it’s crucial to use it judiciously, considering potential drawbacks and accessibility implications.