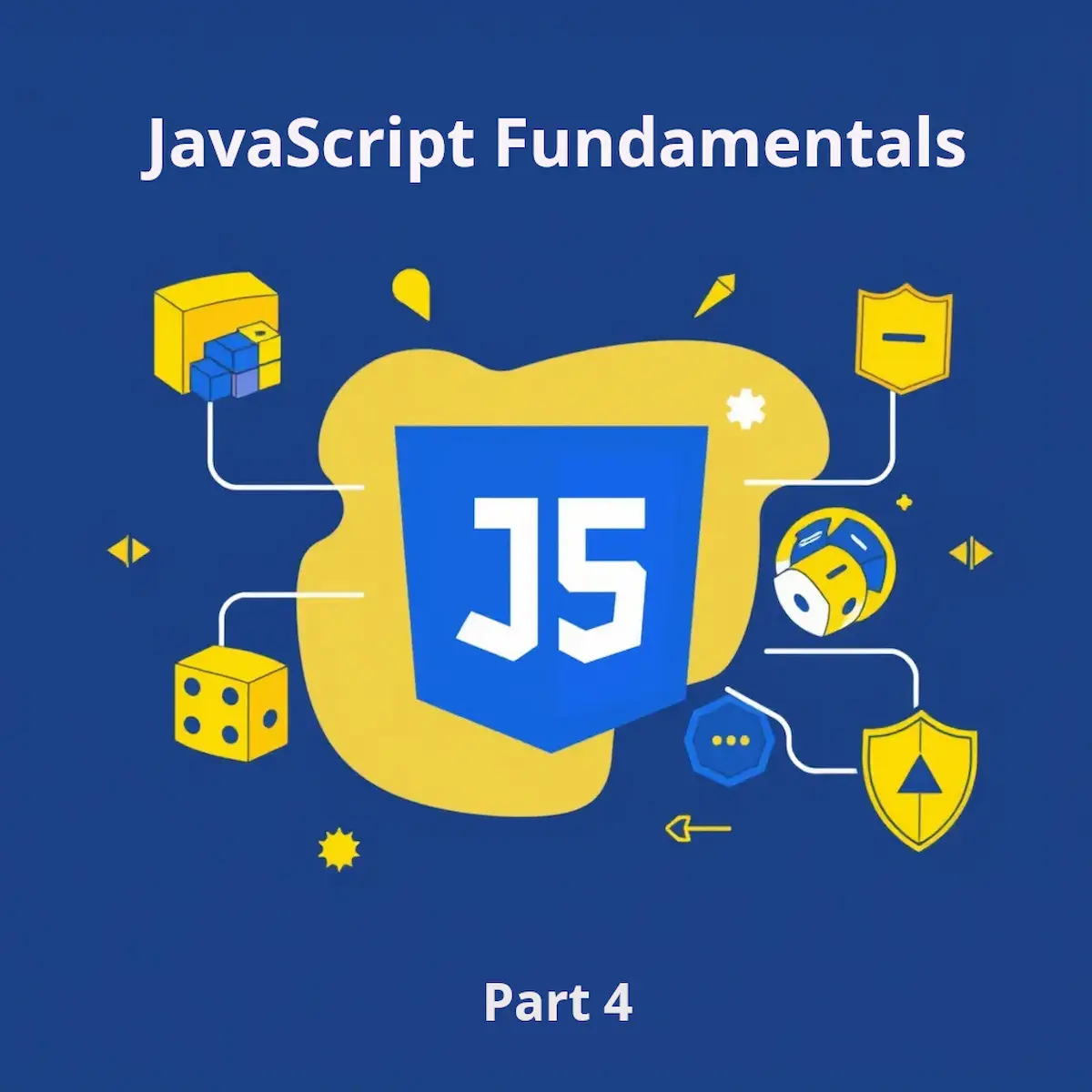
Mastering JavaScript Fundamentals: A Comprehensive Guide - Part 4
- Ctrl Man
- Programming , JavaScript
- 28 May, 2024
Mastering JavaScript as a Beginner: A Fun and Interactive Journey
Introduction
JavaScript is a programming language that brings web pages to life, making them interactive and dynamic. As a beginner, learning JavaScript might seem daunting, but fear not! With the right approach and a touch of creativity, you can embark on an exciting journey to master this versatile language. In this article, we’ll explore JavaScript through a fun and interactive example that will keep you engaged and motivated throughout the learning process.
The Simon Game: A Hands-On Learning Experience
One of the best ways to learn programming is by building something tangible and interactive. In this article, we’ll guide you through the process of creating a classic game called “Simon Game” using JavaScript. The Simon Game is a memory-based game where you’ll need to remember a sequence of colors and repeat them back. It’s a perfect project for beginners as it covers various JavaScript concepts while keeping the learning process engaging and entertaining.
Understanding Callback Functions: The Heart of Asynchronous JavaScript
As you dive into building the Simon Game, you’ll encounter a crucial concept in JavaScript: callback functions. These functions act like messages you send to other functions, asking them to call you back when they’re done with their work. Imagine you’re at a restaurant, and you order some food. Instead of waiting at the counter until your food is ready, you’re given a buzzer. When your food is cooked, the buzzer goes off, letting you know it’s time to pick up your meal. Callback functions work in a similar way, allowing your code to continue running while waiting for a specific task to complete, such as fetching data from a server or handling user interactions.
Bringing Interactivity to Life: Event Handlers and DOM Manipulation
One of the key aspects of building the Simon Game is handling user interactions. In JavaScript, you’ll learn about event handlers, which allow you to respond to user actions such as clicks, keystrokes, and more. You’ll also explore DOM (Document Object Model) manipulation, which enables you to dynamically update and modify the content and structure of a web page based on user interactions and game logic.
Here’s an example of how to handle user interactions and DOM manipulation using jQuery:
gamePattern = [];
var randomNumber;
buttonColours = ["red", "blue", "green", "yellow"];
var randomChosenColour;
function nextSequence() {
randomNumber = Math.floor(Math.random() * 4);
}
nextSequence();
console.log(randomNumber);
randomChosenColour = buttonColours[randomNumber];
gamePattern.push(randomChosenColour);
// Flashing effect using animate() function
var buttonId = "#" + randomChosenColour;
var flashingDuration = 300; // Duration of each flash in milliseconds
var numberOfFlashes = 5; // Number of times the button will flash
function flashButton(buttonId, duration, flashes) {
var button = $(buttonId);
for (var i = 0; i < flashes; i++) {
button.animate({ opacity: 0 }, duration).animate({ opacity: 1 }, duration);
}
}
flashButton(buttonId, flashingDuration, numberOfFlashes);
// Play sound for the selected button color
function playSound(color) {
var sound = new Audio("sounds/" + color + ".mp3");
sound.play();
}
playSound(randomChosenColour); // Play sound for the selected color
Adding Visual and Audio Cues: Animations and Sound Effects
To enhance the user experience and make the Simon Game more engaging, you’ll learn how to incorporate animations and sound effects using JavaScript. You’ll discover techniques for creating flashing effects on buttons and playing audio files corresponding to different colors. These visual and auditory cues will not only make your game more enjoyable but also reinforce your understanding of JavaScript concepts like event handling and function callbacks.
Here’s how you can animate the button and play sound:
// Attach click event listener to the button with the corresponding color ID
$("#" + randomChosenColour).click(function() {
playSound(randomChosenColour);
flashButton(buttonId, flashingDuration, numberOfFlashes);
});
Debugging and Problem-Solving Strategies
As with any programming endeavor, you may encounter challenges and bugs along the way. This article will guide you through effective debugging and problem-solving strategies, such as using the browser’s developer tools, logging values to the console, and step-by-step debugging. By learning these essential skills, you’ll develop a solid foundation for identifying and resolving issues in your code, a crucial aspect of becoming a proficient JavaScript developer.
Here’s an example of setting up user interactions and checking the user’s input against the game pattern:
var userClickedPattern = [];
$(".btn").click(function () {
userChosenColour = $(this).attr("id");
userClickedPattern.push(userChosenColour);
console.log(userChosenColour);
$("#" + userChosenColour).addClass("pressed");
setTimeout(function () {
$("#" + userChosenColour).removeClass("pressed");
}, 100);
checkAnswer(userClickedPattern.length - 1);
});
function checkAnswer(currentLevel) {
if (gamePattern[currentLevel] === userClickedPattern[currentLevel]) {
console.log("success");
if (userClickedPattern.length === gamePattern.length) {
setTimeout(function () {
nextSequence();
}, 1000);
}
} else {
console.log("wrong");
}
}
Conclusion
Learning JavaScript as a beginner can be an exciting and rewarding journey, especially when you approach it through a fun and interactive project like the Simon Game. By following the step-by-step guidance provided in this article, you’ll not only master essential JavaScript concepts but also develop valuable problem-solving skills and gain hands-on experience in building a fully functional game. So, get ready to dive into the world of JavaScript and embark on an engaging adventure that will unleash your creativity and set you on the path to becoming a skilled developer.