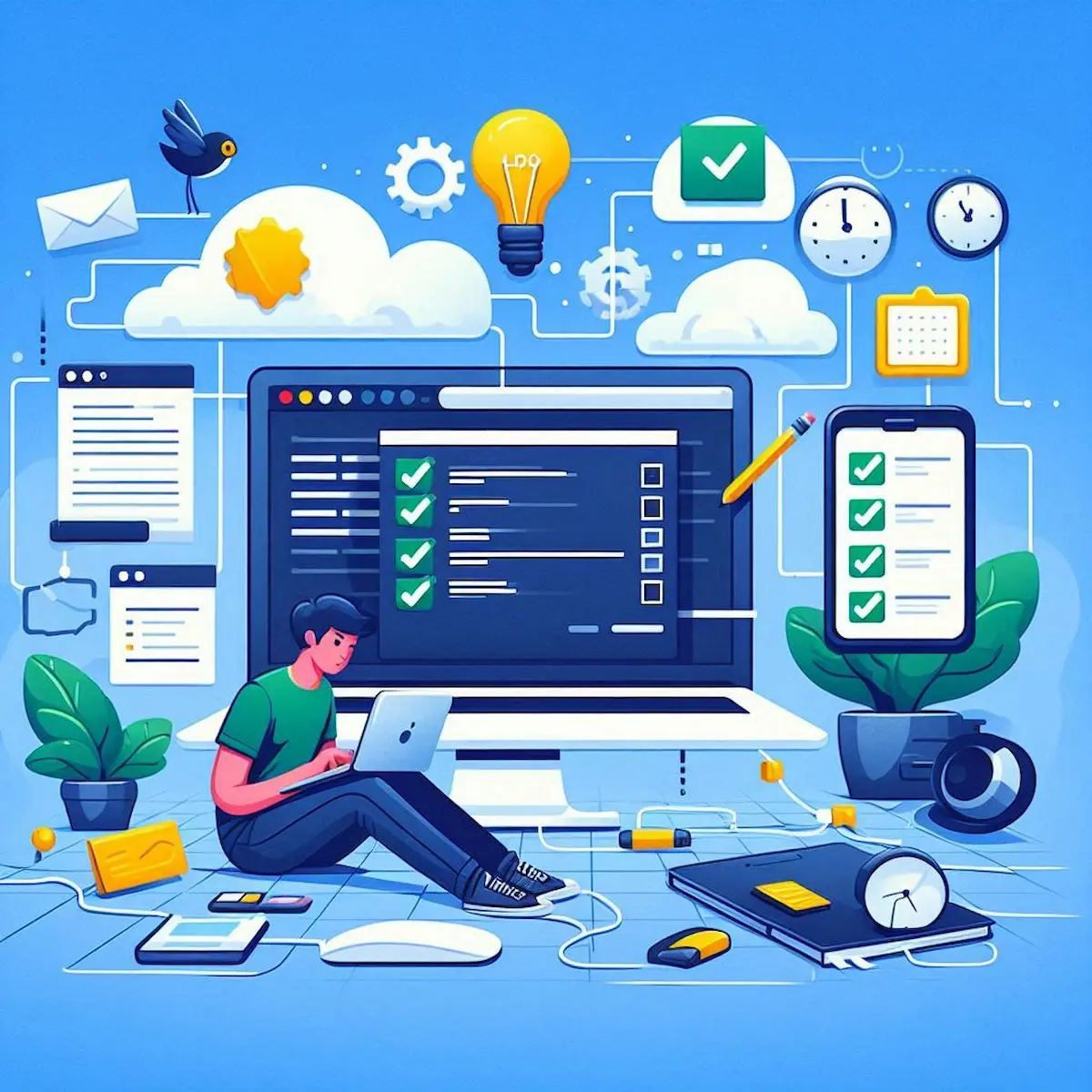
Building Your First Web App: A Beginner's Guide to Creating a To-Do List with Node.js and Express
- Ctrl Man
- Web Development , JavaScript
- 04 Jul, 2024
Building Your First Web App: A Beginner’s Guide to Creating a To-Do List with Node.js and Express
Introduction
Embarking on your web development journey can be both exciting and overwhelming. With countless frameworks, tools, and concepts to learn, it’s crucial to start with something simple yet practical. This guide will walk you through creating a basic to-do list application, providing a solid foundation for your web development skills.
By the end of this tutorial, you’ll have built a functional web application that allows users to add and view tasks. We’ll be using Node.js and Express.js, two powerful tools in modern web development.
Prerequisites
Before we begin, make sure you have the following installed on your computer:
- Node.js (Download from nodejs.org)
- A text editor (We recommend Visual Studio Code, but any text editor will do)
Setting Up Your Project
-
Create a new directory for your project:
mkdir todo-list-app cd todo-list-app
-
Initialize a new Node.js project:
npm init -y
-
Install the required dependencies:
npm install express ejs
Creating the Server
Let’s start by setting up our server. Create a new file called app.js
in your project directory:
const express = require('express');
const app = express();
const port = 3000;
// Set up EJS as the view engine
app.set('view engine', 'ejs');
// Middleware to parse form data
app.use(express.urlencoded({ extended: true }));
// Array to store our to-do items
let items = [];
// Route to display the to-do list
app.get('/', (req, res) => {
let today = new Date();
let options = { weekday: 'long', month: 'long', day: 'numeric' };
let day = today.toLocaleDateString("en-US", options);
res.render('index', { kindOfDay: day, items: items });
});
// Route to handle adding new items
app.post('/', (req, res) => {
let newItem = req.body.newItem;
if (newItem) {
items.push(newItem);
}
res.redirect('/');
});
// Start the server
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
Creating the View
Now, let’s create our view. Make a new directory called views
and create a file named index.ejs
inside it:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>To-Do List</title>
<style>
body { font-family: Arial, sans-serif; max-width: 500px; margin: 0 auto; padding: 20px; }
h1 { color: #333; }
form { margin-bottom: 20px; }
input[type="text"] { width: 70%; padding: 10px; }
button { padding: 10px 20px; background-color: #4CAF50; color: white; border: none; cursor: pointer; }
ul { list-style-type: none; padding: 0; }
li { background-color: #f1f1f1; margin-bottom: 10px; padding: 10px; border-radius: 5px; }
</style>
</head>
<body>
<h1><%= kindOfDay %>'s To-Do List</h1>
<form action="/" method="POST">
<input type="text" name="newItem" placeholder="Add a new item" required>
<button type="submit">Add</button>
</form>
<ul>
<% items.forEach(item => { %>
<li><%= item %></li>
<% }); %>
</ul>
</body>
</html>
Running Your Application
To start your application, run the following command in your terminal:
node app.js
Now, open your web browser and navigate to http://localhost:3000
. You should see your to-do list application!
Understanding the Code
Let’s break down what’s happening in our application:
-
Server Setup: We use Express to create a server and set up EJS as our view engine.
-
Routes:
- The GET route (
/
) renders our main page with the current date and list of items. - The POST route (
/
) handles form submissions, adding new items to our list.
- The GET route (
-
View: Our EJS template displays the current date, a form for adding new items, and the list of existing items.
-
Styling: We’ve included some basic CSS to make our app look presentable.
Next Steps
Congratulations! You’ve built your first web application. Here are some ideas to enhance your to-do list app:
- Persistence: Use a database like MongoDB to store your items permanently.
- User Authentication: Add login functionality so each user can have their own list.
- Delete and Edit: Implement features to remove or modify existing items.
- Due Dates: Allow users to set due dates for their tasks.
- Categories: Let users organize tasks into different categories.
Conclusion
This tutorial has introduced you to the basics of web development using Node.js and Express. You’ve learned how to set up a server, handle routes, process form data, and render dynamic content. As you continue your journey, remember that web development is vast and ever-evolving. Keep practicing, exploring new concepts, and building more complex projects.
Happy coding!