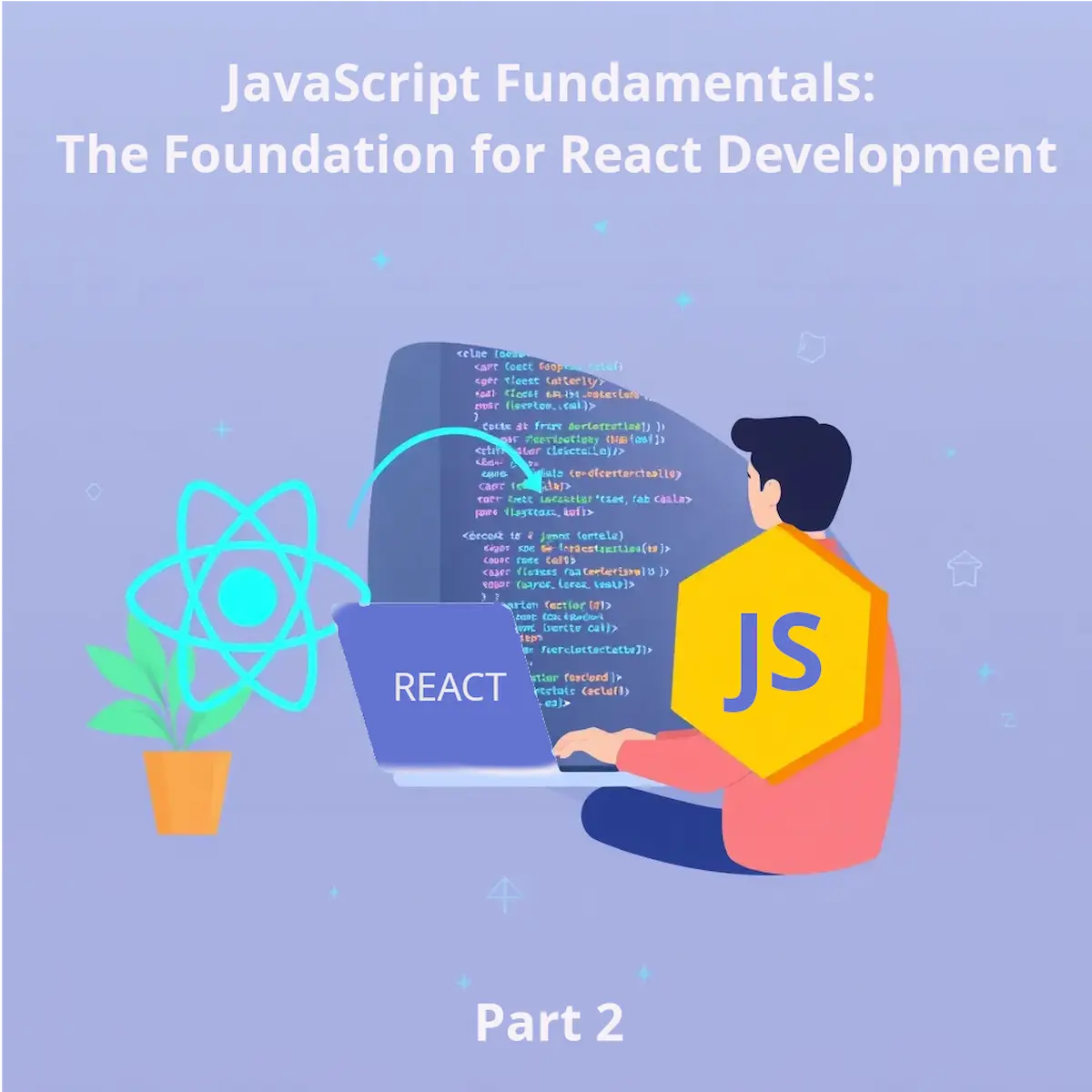
Introduction to React: Building on Your JavaScript Knowledge
- Ctrl Man
- Web Development , JavaScript Frameworks
- 19 Jul, 2024
Introduction to React: Building on Your JavaScript Knowledge
Transitioning to React
React is a powerful library developed by Facebook, primarily used for building user interfaces. It builds on JavaScript’s core principles and enhances them with components-driven architecture.
What is React and Why Use It?
React introduces several key concepts that build upon JavaScript, including the Virtual DOM. The Virtual DOM is a lightweight copy of the actual DOM that React uses to optimize rendering performance. When changes occur in a React application, they’re first applied to the Virtual DOM. React then efficiently updates only the necessary parts of the actual DOM, reducing the number of costly DOM manipulations.
Let’s look at a simple example using the useState
hook, which is fundamental to managing state in functional components:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
In this example, useState
allows us to add state to a functional component, managing a count
variable that updates when the button is clicked.
How React Builds on JavaScript Knowledge
React’s syntax and concepts are deeply rooted in JavaScript, making it an easy transition for those already proficient in JS:
- Components as Functions/Classes: Directly leverage JavaScript to define reusable UI elements.
- Props and State: Continue using these core JS concepts for managing data within components.
- Event Handling: Utilizes standard JavaScript event listeners with a slightly different syntax.
Basic React Concepts
Components and JSX
React uses components to build the UI, which can be defined in both functional or class-based ways:
Functional Component Example:
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
Class-Based Component Example:
class Greeting extends React.Component {
render() {
const { name } = this.props;
return <h1>Hello, {name}!</h1>;
}
}
JSX is a syntax extension for JavaScript that makes it easier to work with the DOM:
Example using JSX:
function Greeting(name) {
return (
<div>
<h1>Hello, {name}!</h1>
</div>
);
}
Props and State
Props allow components to receive data from their parents:
Parent Component Example:
<ParentComponent name="Alice">
</ParentComponent>
Child Component Example:
function ParentComponent({ name }) {
return <Greeting name={name} />;
}
State is used for local data management within a component. Here’s an example:
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
incrementCount = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<button onClick={this.incrementCount}>Increment</button>
<p>Count is: {this.state.count}</p>
</div>
);
}
}
Comparative Examples: JavaScript vs React
Example 1: Simple Counter
JavaScript Approach:
function counter() {
let count = 0;
document.getElementById('counter').innerHTML = count++;
const incrementCount = () => {
count++;
document.getElementById('counter').innerHTML = count;
};
}
// Call function on page load or user interaction
counter();
React Approach:
import React from 'react';
function Counter() {
return (
<div>
<button onClick={() => setState({ count: state.count + 1 })}>
Increment
</button>
{state.count}
</div>
);
}
ReactDOM.render(<Counter />, document.getElementById('root'));
Example 2: Dynamic Button Content
JavaScript Approach:
function buttonContent() {
document.getElementById('button').innerHTML = 'Click me!';
const clickHandler = () => {
if (document.getElementById('button').innerHTML === 'Clicked') return;
document.getElementById('button').innerHTML = 'Click me!';
};
}
// Call function on page load or user interaction
clickHandler();
React Approach:
import React from 'react';
function ButtonContent() {
const handleClick = () => {
setState({ text: 'Clicked' });
setTimeout(() => {
setState({ text: 'Click me!' });
}, 1000);
};
return (
<div
>
<button onClick={handleClick}>
{state.text}
</button>
</div>
);
}
ReactDOM.render(<ButtonContent />, document.getElementById('root'));
Example 3: Fetching Data from an API
JavaScript Approach:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
React Approach:
import React, { useState, useEffect } from 'react';
function FetchData() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data))
.catch(error => console.error('Error:', error));
}, []);
return (
<div>
{data ? <p>Data: {JSON.stringify(data)}</p> : <p>Loading...</p>}
</div>
);
}
ReactDOM.render(<FetchData />, document.getElementById('root'));
Conclusion
With these basics of React, you can start building more complex and interactive user interfaces. In our next article, we’ll explore advanced React concepts and best practices.