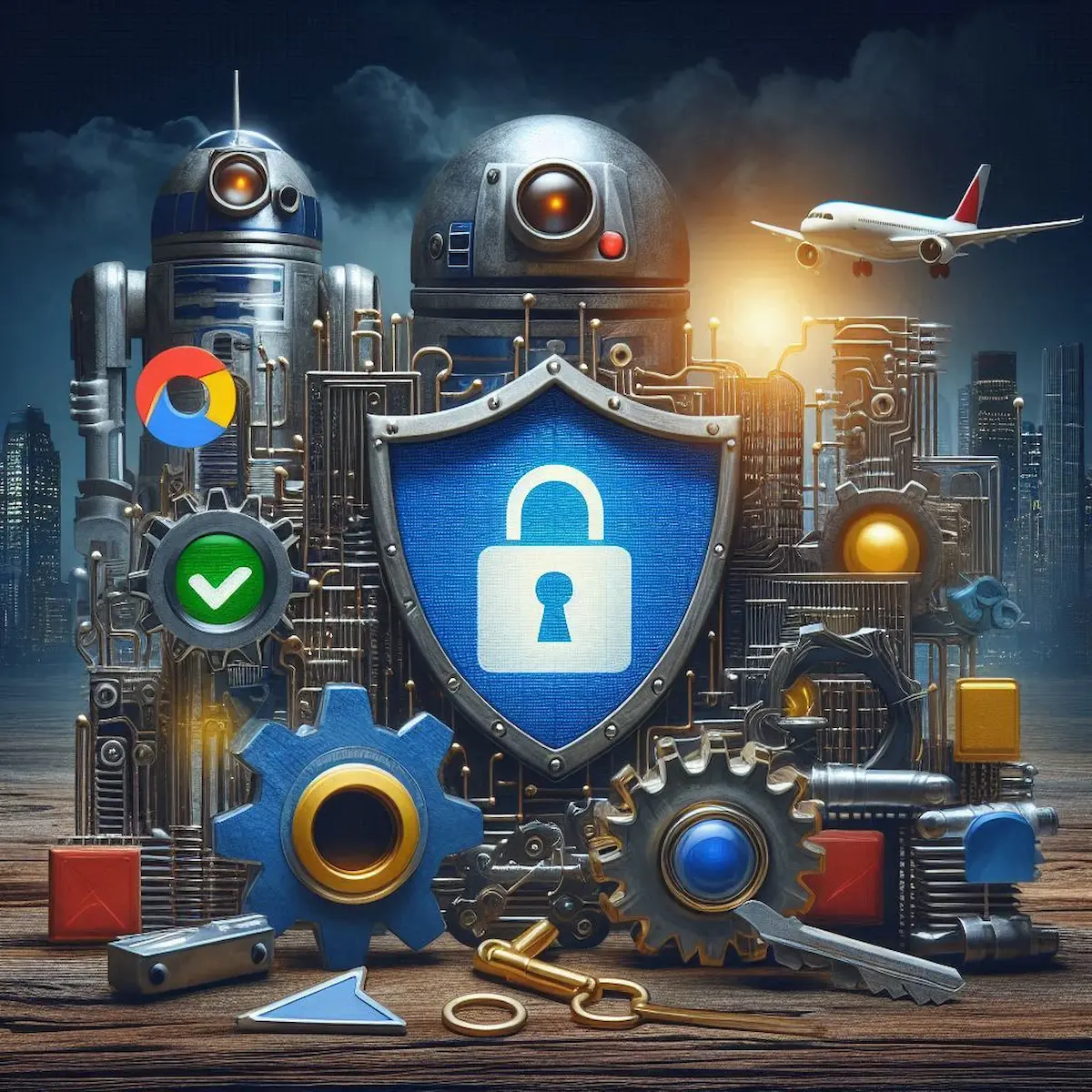
Integrating Google reCAPTCHA for Enhanced Website Security
- Ctrl Man
- Web Development , Security , API Integration
- 26 Jun, 2024
Integrating Google reCAPTCHA for Enhanced Website Security
Introduction
In an era where cyber threats are increasingly sophisticated, protecting your website from automated attacks is crucial. Google’s reCAPTCHA service offers a powerful solution to distinguish between human users and automated scripts, significantly enhancing your website’s security. This comprehensive guide will walk you through the process of integrating reCAPTCHA, exploring its features, and implementing best practices for optimal protection.
Understanding reCAPTCHA
Google’s reCAPTCHA is an advanced security service that leverages machine learning and adaptive challenges to protect websites from spam and abuse. By analyzing user behavior and interactions, reCAPTCHA can effectively differentiate between legitimate users and malicious bots.
Key Features and Benefits
- Intelligent User Verification: reCAPTCHA uses risk analysis algorithms to assess the likelihood of a user being human, often without requiring explicit challenges.
- Adaptive Security: The service continuously learns and adapts to new threat patterns, providing evolving protection against emerging bot techniques.
- Multiple Versions:
- reCAPTCHA v2: Offers checkbox and invisible options.
- reCAPTCHA v3: Provides a score-based system for assessing user interactions without interrupting the user flow.
- Cross-Platform Support: Works seamlessly across desktop and mobile devices.
- Accessibility: Includes audio alternatives for visually impaired users.
- Customization Options: Allows for styling adjustments to match your website’s design.
Setting up Google reCAPTCHA
Step-by-Step Guide
1. Register Your Website
a. Navigate to the reCAPTCHA Admin Console. b. Sign in with your Google account. c. Click on the ”+” icon to add a new site. d. Choose the reCAPTCHA type (v2 or v3) based on your needs. e. Add your domain(s) to the list of allowed domains.
2. Obtain API Keys
a. After registration, you’ll receive two important keys: - Site Key: Used in client-side code (public) - Secret Key: Used in server-side verification (keep this private) b. Store these keys securely, preferably as environment variables.
3. Integrate reCAPTCHA into Your Frontend
a. Add the reCAPTCHA script to your HTML:
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
b. Insert the reCAPTCHA widget in your form:
<form action="?" method="POST">
<div class="g-recaptcha" data-sitekey="YOUR_SITE_KEY"></div>
<input type="submit" value="Submit">
</form>
4. Server-Side Verification (Node.js/Express.js Example)
a. Install the axios
package to make HTTP requests:
npm install axios
b. Implement the verification logic:
const axios = require('axios');
const express = require('express');
const app = express();
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
app.post('/submit-form', async (req, res) => {
const { 'g-recaptcha-response': recaptchaResponse } = req.body;
try {
const verificationURL = 'https://www.google.com/recaptcha/api/siteverify';
const result = await axios.post(verificationURL, null, {
params: {
secret: process.env.RECAPTCHA_SECRET_KEY,
response: recaptchaResponse
}
});
if (result.data.success) {
// reCAPTCHA verification passed
res.send('Form submitted successfully!');
} else {
// reCAPTCHA verification failed
res.status(400).send('reCAPTCHA verification failed. Please try again.');
}
} catch (error) {
console.error('Error verifying reCAPTCHA:', error);
res.status(500).send('An error occurred during verification.');
}
});
app.listen(3000, () => console.log('Server running on port 3000'));
Best Practices and Advanced Implementation
Security Considerations
- Environment Variables: Always store your Secret Key in environment variables, never in your source code.
- HTTPS: Ensure your website uses HTTPS to protect the reCAPTCHA token during transmission.
- Backend Validation: Always verify the reCAPTCHA response on your server, never relying solely on client-side checks.
Implementing reCAPTCHA v3
reCAPTCHA v3 operates invisibly, returning a score that indicates the likelihood of the user being a bot.
- Include the reCAPTCHA v3 script
<script src="https://www.google.com/recaptcha/api.js?render=YOUR_SITE_KEY"></script>
- Execute reCAPTCHA on form submission
grecaptcha.ready(function() {
grecaptcha.execute('YOUR_SITE_KEY', {action: 'submit'}).then(function(token) {
// Add token to form
document.getElementById('g-recaptcha-response').value = token;
});
});
- **Verify the score on your server and decide on an appropriate threshold (e.g., 0.5)
Error Handling and User Experience
- Implement graceful degradation if reCAPTCHA fails to load.
- Provide clear error messages if verification fails.
- Consider offering alternative verification methods for accessibility.
Troubleshooting Common Issues
-
“Error for site owner: Invalid domain for site key”
- Solution: Ensure the domain you’re using is listed in the reCAPTCHA admin console.
-
reCAPTCHA not rendering
- Check for JavaScript console errors.
- Verify that your Site Key is correct.
-
Verification always failing
- Double-check your Secret Key.
- Ensure your server’s clock is synchronized (NTP).
-
Performance impacts
- Use the
async defer
attributes when loading the reCAPTCHA script. - For v3, consider preloading the reCAPTCHA script for critical pages.
- Use the
Conclusion
Integrating Google reCAPTCHA into your website is a powerful step towards enhancing security and protecting against automated threats. By following this guide and adhering to best practices, you can effectively implement reCAPTCHA while maintaining a smooth user experience. Remember to stay updated with the latest reCAPTCHA features and continuously monitor its effectiveness in your specific use case.
For the most up-to-date information and advanced configurations, always refer to the official Google reCAPTCHA documentation.
By taking proactive steps to secure your website with tools like reCAPTCHA, you’re not just protecting your data – you’re safeguarding your users’ trust and your brand’s reputation in the digital landscape.