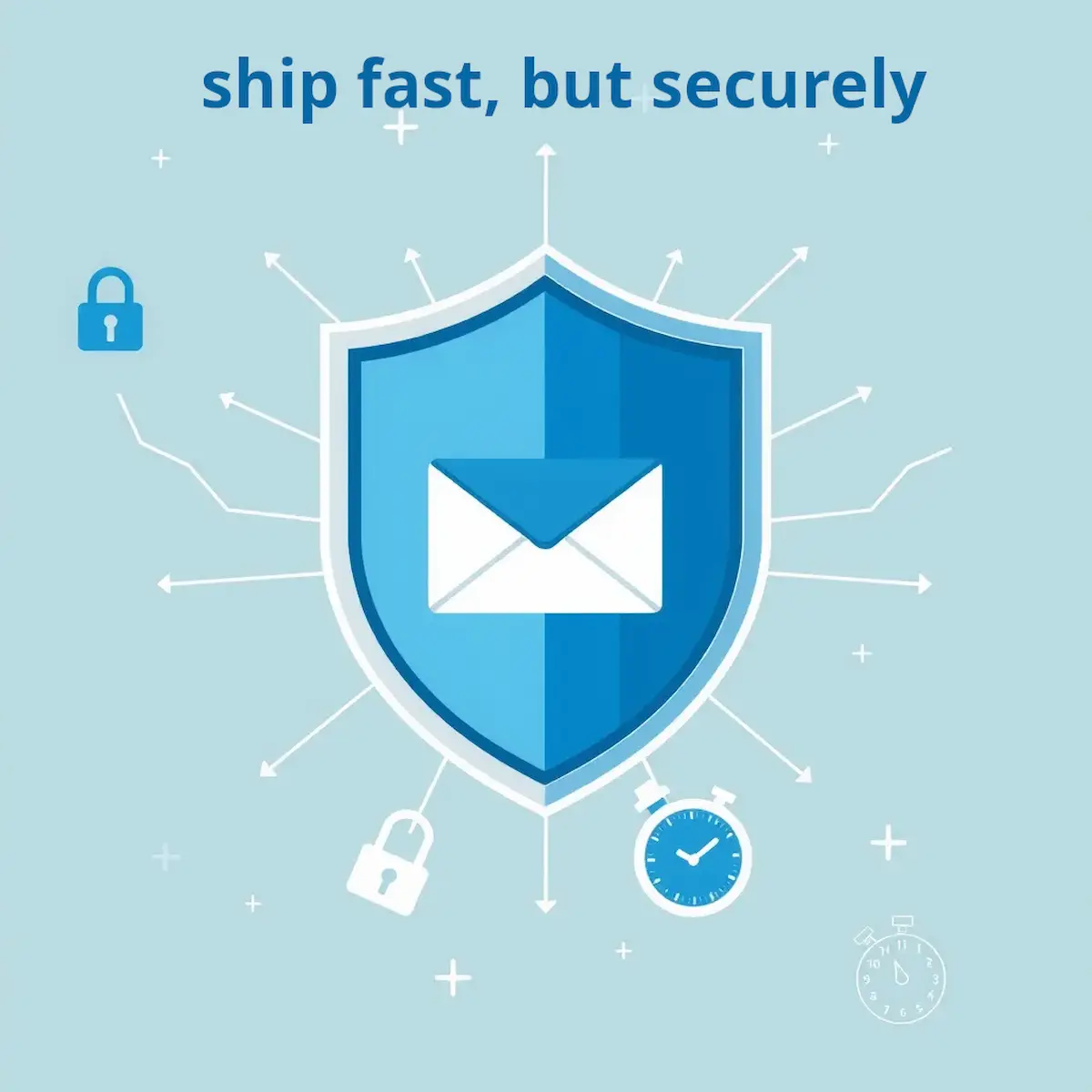
Securing Next.js API Endpoints: A Comprehensive Guide to Email Handling and Security Best Practices
- Ctrl Man
- Web Development , Security
- 20 Oct, 2024
Securing Next.js API Endpoints: A Comprehensive Guide to Email Handling and Security Best Practices
Introduction
In the fast-paced world of web development, rapid code deployment is often necessary. However, it’s crucial not to compromise security in the process. This article examines a Next.js API endpoint that interacts with Mailgun’s webhook service to handle incoming emails. The code we’ll be discussing is based on a widely used implementation found in thousands of instances of the popular shipfa.st boilerplate. While functional, this code leaves room for several security improvements. We’ll explore both its strengths and areas requiring attention to ensure your API endpoints remain secure and reliable.
Code Overview
Let’s start by examining the original code from the shipfa.st boilerplate:
import { NextResponse, NextRequest } from "next/server";
import { sendEmail } from "@/libs/mailgun";
import config from "@/config";
// This route is used to receive emails from Mailgun and forward them to
// our customer support email.
// See more: https://shipfa.st/docs/features/emails
export async function POST(req: NextRequest) {
try {
// extract the email content, subject and sender
const formData = await req.formData();
const sender = formData.get("From");
const subject = formData.get("Subject");
const html = formData.get("body-html");
// send email to the admin if forwardRepliesTo is set & emailData exists
if (config.mailgun.forwardRepliesTo && html && subject && sender) {
await sendEmail({
to: config.mailgun.forwardRepliesTo,
subject: `${config?.appName} | ${subject}`,
html: `<div><p><b>- Subject:</b> ${subject}</p><p><b>- From:</b> ${sender}</p><p><b>- Content:</b></p><div>${html}</div></div>`,
replyTo: String(sender),
});
}
} catch (e) {
console.error(e?.message);
return NextResponse.json({ error: e?.message }, { status: 500 });
}
return NextResponse.json({});
}
This code is designed as a Next.js API endpoint that processes incoming emails via Mailgun’s webhook service. It extracts essential information such as the sender’s email, subject, and HTML content, then forwards this data to an admin email specified in the configuration file.
Strengths
- Core Functionality: The main logic for extracting and forwarding email content works as intended.
- Error Handling: The use of a try-catch block prevents the application from crashing unexpectedly.
Areas for Improvement
- Input Validation: The code lacks validation for important fields, posing security risks.
- Authentication: There’s no authentication mechanism to ensure requests come from Mailgun.
- Rate Limiting: Without rate limiting, the endpoint is vulnerable to abuse.
- Error Handling: Detailed error messages might expose sensitive information.
- Code Organization: Business logic is mixed with request handling, affecting maintainability.
Comprehensive Security Improvements
Let’s address these concerns with a more secure implementation:
import { NextResponse, NextRequest } from "next/server";
import { sendEmail } from "@/libs/mailgun";
import config from "@/config";
import crypto from 'crypto';
import validator from 'validator';
import rateLimit from 'express-rate-limit';
// Rate limiting middleware
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100 // limit each IP to 100 requests per windowMs
});
// Verify Mailgun signature
function verifyMailgunWebhook(timestamp, token, signature) {
const encodedToken = crypto
.createHmac('sha256', process.env.MAILGUN_API_KEY)
.update(timestamp + token)
.digest('hex');
return encodedToken === signature;
}
export async function POST(req: NextRequest) {
// Apply rate limiting
await limiter(req, NextResponse);
try {
const formData = await req.formData();
// Verify Mailgun signature
const timestamp = formData.get('timestamp');
const token = formData.get('token');
const signature = formData.get('signature');
if (!verifyMailgunWebhook(timestamp, token, signature)) {
return NextResponse.json({ error: 'Invalid signature' }, { status: 403 });
}
const sender = formData.get("From");
const subject = formData.get("Subject");
const html = formData.get("body-html");
// Input validation
if (!sender || !validator.isEmail(sender)) {
return NextResponse.json({ error: 'Invalid sender email' }, { status: 400 });
}
if (!subject || !html) {
return NextResponse.json({ error: 'Missing required fields' }, { status: 400 });
}
// Sanitize HTML content
const sanitizedHtml = validator.escape(html);
if (config.mailgun.forwardRepliesTo) {
await sendEmail({
to: config.mailgun.forwardRepliesTo,
subject: `${config?.appName} | ${subject}`,
html: `<div><p><b>- Subject:</b> ${subject}</p><p><b>- From:</b> ${sender}</p><p><b>- Content:</b></p><div>${sanitizedHtml}</div></div>`,
replyTo: String(sender),
});
}
return NextResponse.json({ status: 'success' });
} catch (e) {
console.error('Error processing email:', e);
return NextResponse.json({ error: 'Internal server error' }, { status: 500 });
}
}
Key Improvements
- Input Validation: We now use the
validator
library to check the sender’s email and sanitize HTML content. - Authentication: We’ve implemented Mailgun signature verification to ensure requests come from Mailgun.
- Rate Limiting: The
express-rate-limit
middleware protects against abuse. - Error Handling: Error messages are now generic to avoid exposing sensitive information.
- Code Organization: The signature verification logic is separated into its own function for better maintainability.
Additional Security Considerations
1. Environment Variables
Ensure that sensitive information like API keys are stored as environment variables, not in the code.
2. HTTPS
Always use HTTPS in production to encrypt data in transit.
3. Regular Updates
Keep all dependencies up-to-date to benefit from the latest security patches.
4. Logging
Implement structured logging for better monitoring and debugging without exposing sensitive data.
5. Content Security Policy (CSP)
Implement a strong Content Security Policy to mitigate risks like XSS attacks.
Conclusion
By implementing these security measures, we’ve significantly improved the robustness and security of our Next.js API endpoint for email handling. Remember, security is an ongoing process. Regularly review and update your security practices to stay ahead of potential threats.