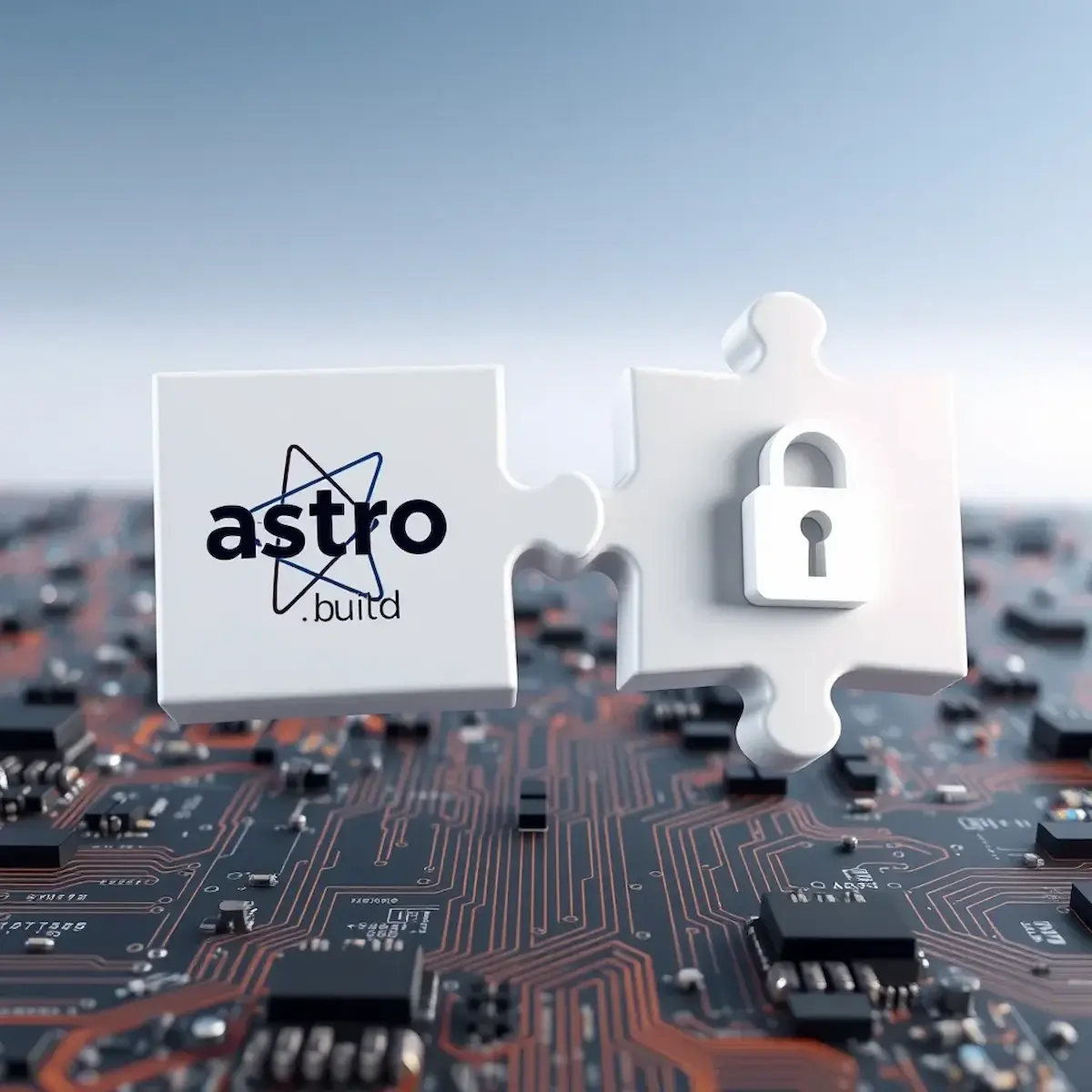
Testing GitHub OAuth Authentication Locally in Astro Build with Lucia and ngrok
- Ctrl Man
- Web Development , Authentication , OAuth , Astro Build
- 25 Sep, 2024
Setting Up Lucia for Astro Build: Testing GitHub Authentication Locally Using ngrok
Introduction
In this article, we will walk through the steps to set up a secure authentication system with Lucia and an Astro.build project. We’ll focus on integrating GitHub OAuth authentication and demonstrate how to test it locally using ngrok.
Prerequisites
- Astro Build Project: Ensure your Astro build project is set up.
- Lucia Authentication Library: Installed in your project.
- GitHub OAuth App: Created with the necessary credentials: Client ID and Client Secret you can get here.
Step-by-Step Guide
1. Setting Up Lucia Configuration
First, let’s create a basic configuration for Lucia in auth.ts
.
import { Lucia } from "lucia";
import { BetterSqlite3Adapter } from "@lucia-auth/adapter-sqlite";
import { db } from "./db";
import type { DatabaseUser } from "./db";
const adapter = new BetterSqlite3Adapter(db, {
user: "user",
session: "session"
});
export const lucia = new Lucia(adapter, {
sessionCookie: {
attributes: {
secure: import.meta.env.PROD
}
},
getUserAttributes: (attributes) => {
return {
username: attributes.username,
githubId: attributes.github_id
};
}
});
declare module "lucia" {
interface Register {
Lucia: typeof lucia;
DatabaseUserAttributes: Omit<DatabaseUser, "id">;
}
}
export const github = new GitHub(
import.meta.env.GITHUB_CLIENT_ID,
import.meta.env.GITHUB_CLIENT_SECRET
);
2. Setting Up Environment Variables
Create a .env
file to store your environment-specific variables.
# For production
GITHUB_CLIENT_ID=your_production_client_id
GITHUB_CLIENT_SECRET=your_production_client_secret
# For development
VITE_GITHUB_CLIENT_ID=your_development_client_id
VITE_GITHUB_CLIENT_SECRET=your_development_client_secret
3. Setting Up Nginx (Optional)
If you’re using Nginx as a reverse proxy, configure it to handle the OAuth callback.
server {
listen 80;
server_name localhost;
location /auth/github/callback {
proxy_pass http://localhost:3000; # Assuming your Astro app runs on port 3000
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
}
4. Testing Locally Using ngrok
To test your GitHub OAuth setup locally, you can use ngrok to expose your localhost to the internet.
-
Install ngrok:
- Download and install the appropriate version for Windows: Download ngrok
-
Add Authtoken: Run the following command to add your authtoken to the default
ngrok.yml
configuration file.ngrok config add-authtoken 2mYdlXwJyGxEn7x1uxVl1ZHiPJK_EqUgoP4eV5r8nhqZtucu
-
Deploy Your App: Run the following command to expose your local server on port
4321
.ngrok http http://localhost:4321
-
Update GitHub OAuth Settings: Go to the GitHub OAuth app settings and update the Authorization callback URL with the ngrok-generated public URL.
-
Test Locally:
- Open your browser and initiate the OAuth login flow from GitHub.
- The OAuth process should redirect to
http://localhost:4321/auth/github/callback
via ngrok.
Full Example of auth.ts
Here is the full example of how you can set up auth.ts
for both local development and production environments:
import { Lucia } from "lucia";
import { BetterSqlite3Adapter } from "@lucia-auth/adapter-sqlite";
import { db } from "./db";
import { GitHub } from "arctic";
import type { DatabaseUser } from "./db";
const adapter = new BetterSqlite3Adapter(db, {
user: "user",
session: "session"
});
export const lucia = new Lucia(adapter, {
sessionCookie: {
attributes: {
secure: import.meta.env.PROD
}
},
getUserAttributes: (attributes) => {
return {
username: attributes.username,
githubId: attributes.github_id
};
}
});
declare module "lucia" {
interface Register {
Lucia: typeof lucia;
DatabaseUserAttributes: Omit<DatabaseUser, "id">;
}
}
export const github = new GitHub(
import.meta.env.GITHUB_CLIENT_ID,
import.meta.env.GITHUB_CLIENT_SECRET,
{
redirectUri: import.meta.env.PROD
? "https://ctrlman.dev/auth/github/callback" // Production URL
: "http://localhost:4321/auth/github/callback" // Localhost URL
}
);
Conclusion
By following the steps outlined in this article, you should be able to set up a secure GitHub OAuth authentication system for your Astro build project and test it locally using ngrok. This setup ensures that your application is both flexible and robust, providing a seamless development experience while maintaining security.
If you encounter any issues or have further questions, feel free to reach out! Happy coding!