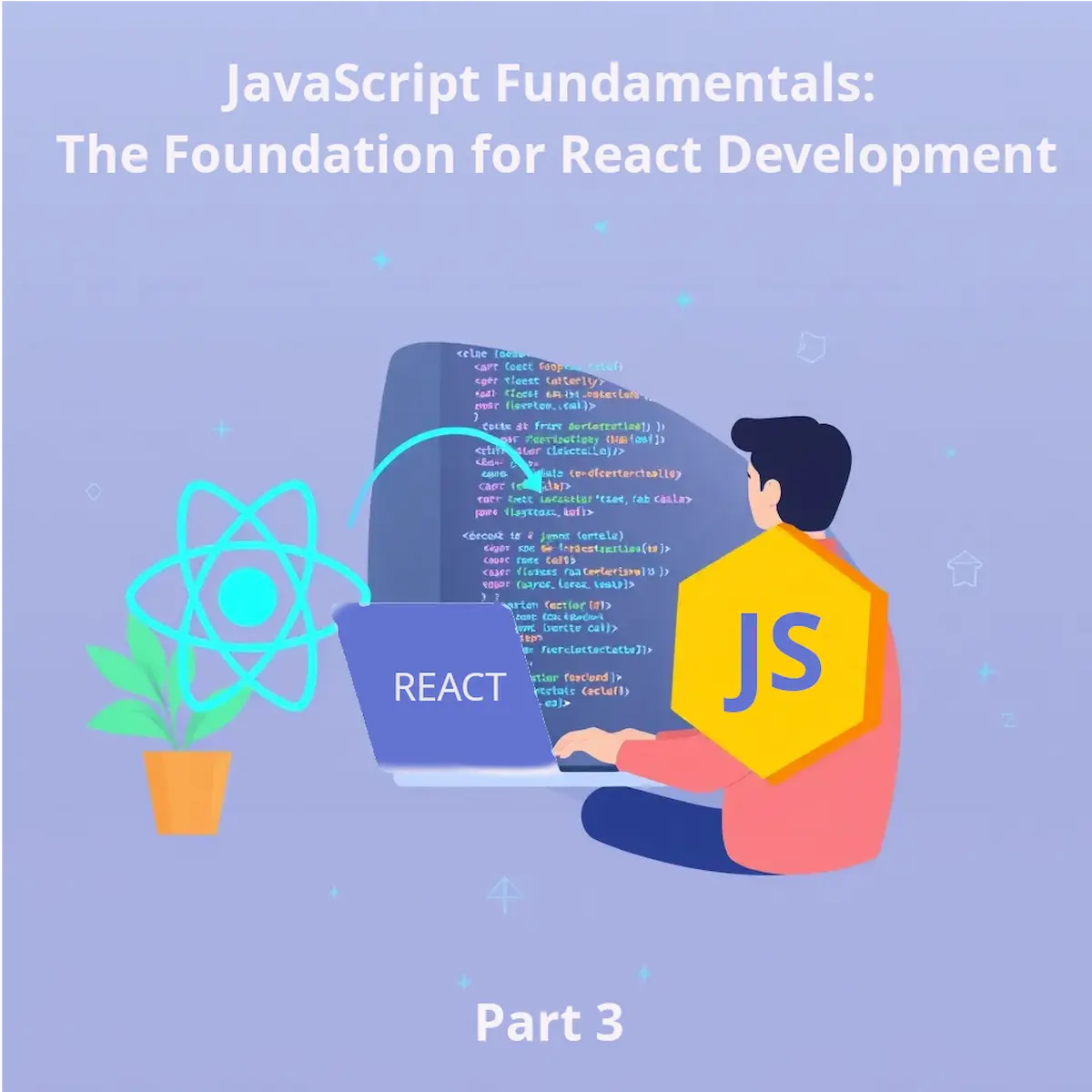
Advanced React Development and Best Practices
- Ctrl Man
- Web Development , JavaScript Frameworks
- 22 Jul, 2024
Advanced React Development and Best Practices
Advanced React Topics
Refs and the useRef
Hook
Refs allow you to interact with the DOM directly from functional components:
Example:
import React, { useRef } from 'react';
function Example() {
const inputRef = useRef(null);
function handleClick() {
// Use ref to access DOM element directly:
console.log(inputRef.current.value);
}
return (
<div>
<input type="text" ref={inputRef} />
<button onClick={handleClick}>Get Value</button>
</div>
);
}
ReactDOM.render(<Example />, document.getElementById('root'));
Form Handling and Custom Reset Functions
import React, { useState } from 'react';
function Form() {
const [name, setName] = useState('');
function handleSubmit(e) {
e.preventDefault();
// Handle form submission logic here:
}
return (
<form onSubmit={handleSubmit}>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<button type="submit">Submit</button>
<button onClick={() => setName('')}>Reset</button> {/* Custom reset function */}
</form>
);
}
ReactDOM.render(<Form />, document.getElementById('root'));
Other Important Hooks
React provides several other useful hooks:
- useEffect: Manages side effects in functional components, such as data fetching or DOM manipulation.
- useContext: Allows components to consume data from a React context without prop drilling.
- useReducer: Provides more complex state management, similar to Redux but built into React.
State Management Solutions
For larger applications, you might consider using state management libraries:
- Redux: A predictable state container that helps manage global application state.
- MobX: Provides simple and scalable state management using observable state.
- Recoil: A newer state management library developed by Facebook, designed for React’s concurrent mode.
These solutions can help manage complex state interactions and data flow in larger React applications.
Web Accessibility in React
As you learn JavaScript and React, it’s crucial to consider web accessibility. Both vanilla JavaScript and React applications should be built with accessibility in mind, ensuring that all users, including those with disabilities, can interact with your web content effectively. This includes using semantic HTML, managing focus for keyboard navigation, providing alternative text for images, and ensuring sufficient color contrast. React’s component-based architecture can be leveraged to create reusable, accessible UI elements.
Recommended Learning Path and Resources
To master both JavaScript and React, consider the following learning path:
- Learn JavaScript Fundamentals: Focus on variables, data types, functions, DOM manipulation, event handling.
- Build Projects in Vanilla JS: Start with simple projects like counters, to-do lists, or calculators.
- Transition to React: Understand the basics of components and JSX, then dive into more advanced features like
useRef
,useEffect
, and state management. - Practice Regularly: Apply what you’ve learned by building projects, either solo or in a community.
Resources:
- Official Docs: React
- Online Courses: React for Beginners on Codecademy
- Video Tutorials: ReactJS Tutorial by Academind on YouTube
- Books: React Key Concepts: Consolidate your knowledge of React’s core features by Maximilian Schwarzmüller.
By following this structured learning path, you’ll be well-equipped to tackle both the fundamentals and advanced features of JavaScript and React. Happy coding!
Conclusion
By mastering these advanced React concepts and following best practices, you’ll be well-equipped to build complex, efficient, and accessible web applications. Remember to continue practicing and exploring new React features as they emerge.