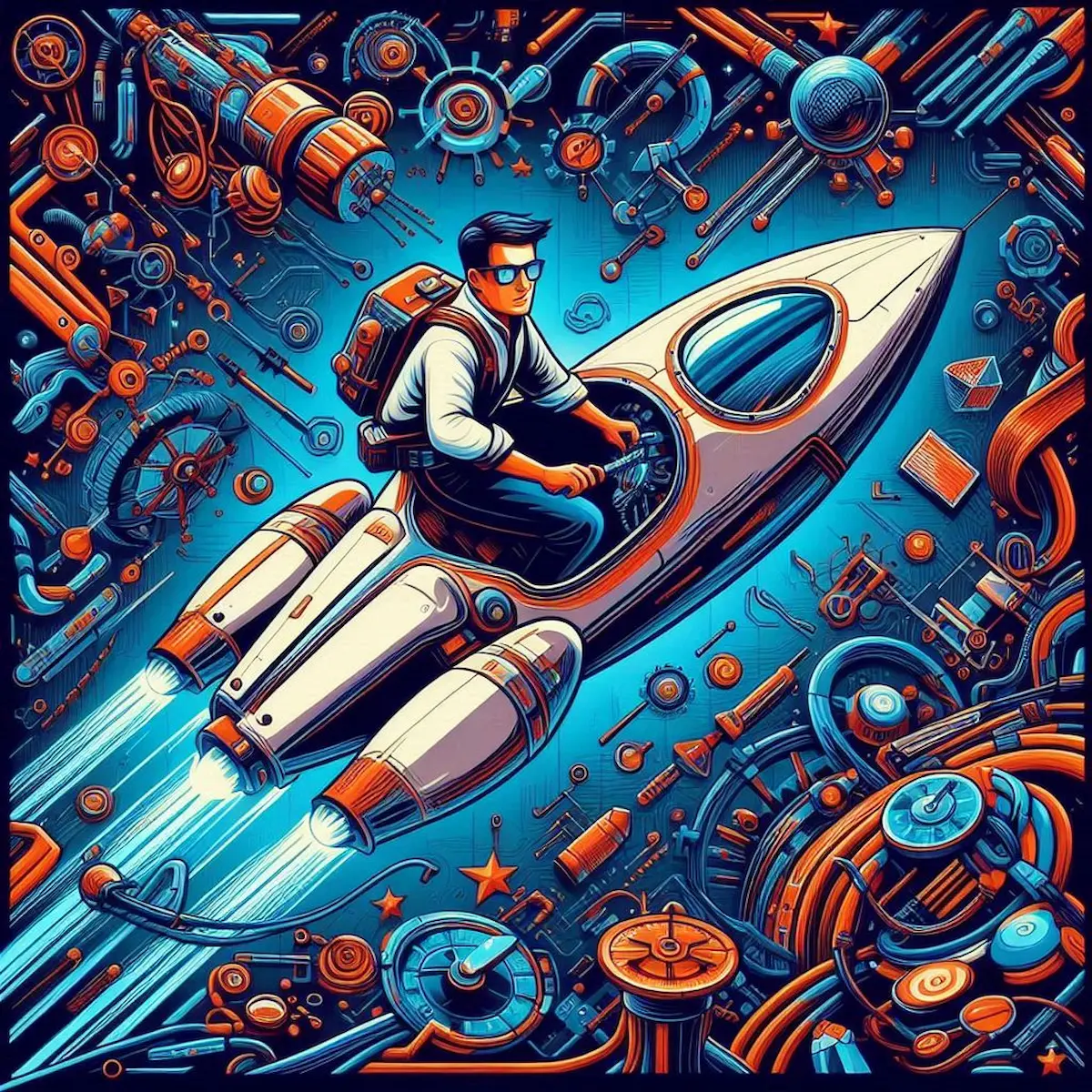
Web Development for Beginners: A Comprehensive Guide Using Rust
Web Development for Beginners: A Comprehensive Guide Using Rust
Introduction
Web development is an exciting field filled with opportunities to create dynamic and engaging user experiences. Rust, a modern systems programming language known for its safety, speed, and memory efficiency, is gaining popularity in web development through various frameworks and libraries. This guide aims to introduce beginners to web development using Rust, highlighting its unique features and capabilities.
Understanding Web Frameworks in Rust
What is a Web Framework?
A web framework is a software framework designed to support the development of web applications, including web services, web resources, and web APIs. It provides a standard way to build and deploy web applications on the internet.
Rust Web Frameworks
Rust offers several robust web frameworks that provide powerful features for building web applications. Let’s explore some of the most popular ones:
1. Actix-web
Actix-web is a powerful, pragmatic, and extremely fast web framework for Rust.
use actix_web::{get, App, HttpServer, Responder};
#[get("/")]
async fn hello() -> impl Responder {
"Hello, world!"
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
HttpServer::new(|| {
App::new().service(hello)
})
.bind("127.0.0.1:8080")?
.run()
.await
}
2. Rocket
Rocket is a web framework for Rust that focuses on ease-of-use, expressiveness, and speed.
#[macro_use] extern crate rocket;
#[get("/")]
fn hello() -> &'static str {
"Hello, world!"
}
#[launch]
fn rocket() -> _ {
rocket::build().mount("/", routes![hello])
}
3. Warp
Warp is a super-easy, composable web server framework for warp speeds.
use warp::Filter;
#[tokio::main]
async fn main() {
let hello = warp::path::end().map(|| "Hello, World!");
warp::serve(hello)
.run(([127, 0, 0, 1], 8080))
.await;
}
Rust’s Memory Management: A Key Advantage
One of Rust’s standout features is its unique approach to memory management, which sets it apart from many other programming languages used in web development.
Stack vs. Heap Memory
Understanding the difference between stack and heap memory is crucial for efficient web development in Rust.
Stack Memory
- Fast allocation and deallocation
- Fixed size, known at compile-time
- Automatically managed
Heap Memory
- Dynamic size, flexible allocation
- Slower than stack memory
- Safely handled by Rust’s ownership system
Rust’s Ownership System
Rust’s ownership system is a novel approach to memory management that provides memory safety without a garbage collector.
Key concepts:
- Ownership: Each value has an owner variable.
- Borrowing: References allow data use without taking ownership.
- Lifetimes: The compiler ensures references are valid.
Example:
fn main() {
let s1 = String::from("hello");
let len = calculate_length(&s1);
println!("The length of '{}' is {}.", s1, len);
}
fn calculate_length(s: &String) -> usize {
s.len()
}
Building a Simple Web Application in Rust
Let’s create a basic “Hello, World!” web application using the Actix-web framework to demonstrate Rust’s web development capabilities.
-
Set up a new Rust project:
cargo new hello_rust_web cd hello_rust_web
-
Add Actix-web to your
Cargo.toml
:[dependencies] actix-web = "4.0"
-
Replace the contents of
src/main.rs
with:use actix_web::{get, App, HttpServer, Responder}; #[get("/")] async fn hello() -> impl Responder { "Hello, Rust Web Developer!" } #[actix_web::main] async fn main() -> std::io::Result<()> { HttpServer::new(|| { App::new().service(hello) }) .bind("127.0.0.1:8080")? .run() .await }
-
Run your application:
cargo run
Visit http://localhost:8080
in your browser to see your Rust web application in action!
Conclusion
Rust offers a powerful and safe approach to web development, combining high performance with strong safety guarantees. While the learning curve may be steeper than with some other languages, the benefits in terms of performance, safety, and maintainability make it an excellent choice for modern web development.
As you continue your journey into Rust web development, explore more advanced topics such as:
- Database integration with Rust (e.g., Diesel ORM)
- Asynchronous programming in Rust
- RESTful API design principles
- WebSocket implementation for real-time applications
Remember, the Rust community is vibrant and supportive. Don’t hesitate to seek help on forums, chat channels, or local Rust meetups as you build your skills.
Additional Resources
- The Rust Programming Language Book
- Rust by Example
- Actix-web Documentation
- Awesome Rust - A curated list of Rust code and resources
Happy coding, and welcome to the world of Rust web development!